File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
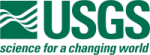 |
Isis 3 Programmer Reference
|
11 #include "AbstractPlotTool.h"
14 #include <QHBoxLayout>
17 #include <QStackedWidget>
20 #include "CubePlotCurve.h"
21 #include "MdiCubeViewport.h"
39 QString selectWindowWhatsThis =
40 "<b>Function:</b> This will allow the selection of a window to place new "
41 "plot curves into. Current curves in this window will be replaced by "
42 "new plot curves. You cannot paste plot curves into this window.";
63 if (!windowVariant.isNull() && windowVariant.canConvert<
PlotWindow *>()) {
85 if (!windowVariant.isNull() && windowVariant.canConvert<
PlotWindow *>()) {
87 window->
paint(vp, painter);
105 QHBoxLayout *layout =
new QHBoxLayout;
106 layout->addWidget(
new QLabel(
"Plot Into:"));
108 layout->addStretch(1);
109 toolBarWidget->setLayout(layout);
111 return toolBarWidget;
140 if (viewport == activeViewport ||
142 viewports.append(viewport);
161 if (!windowVariant.isNull() && windowVariant.canConvert<
PlotWindow *>()) {
163 windows.append(window);
180 if (currentIndex != -1) {
206 QVariant windowVariant = QVariant::fromValue((
PlotWindow *) window);
208 if (currentWindowIndex != -1) {
237 newCurve->setTitle(name);
260 if (curIndex != -1) {
264 if (!windowVariant.isNull() && windowVariant.canConvert<
PlotWindow *>()) {
269 if (!window && createIfNeeded) {
306 connect(newPlotWindow, SIGNAL(closed()),
307 newPlotWindow, SLOT(deleteLater()));
309 QString originalTitle = newPlotWindow->windowTitle();
310 QString titleToTry = originalTitle;
311 bool titleUsed =
false;
316 if (titleNumber > 1) {
317 titleToTry = originalTitle +
" " + QString::number(titleNumber);
323 titleUsed = titleUsed ||
328 newPlotWindow->setWindowTitle(titleToTry);
334 newPlotWindow->windowTitle(), QVariant::fromValue(newPlotWindow));
337 connect(newPlotWindow, SIGNAL(destroyed(
QObject *)),
339 connect(newPlotWindow, SIGNAL(plotChanged()),
342 return newPlotWindow;
366 viewport->viewport()->repaint();
void showWindow()
Shows the plot window, and raises it to the front of any overlapping sibling widgets.
Cube display widget for certain Isis MDI applications.
virtual QString fileName() const
Returns the opened cube's filename.
This is free and unencumbered software released into the public domain.
This is a plot curve with information relating it to a particular cube or region of a cube.
void setPen(const QPen &pen)
Sets the plot pen to the passed-in pen.
Units
These are all the possible units for the x or y data in a plot curve.
virtual void update(MdiCubeViewport *activeViewport)
This is provided to allow children to react to tool updates.
bool isLinked() const
Is the viewport linked with other viewports.
QStringList sourceCube() const
This method returns the cube view port associated with the curve.
void setColor(const QColor &color)
Set the color of this curve and it's markers.
This is free and unencumbered software released into the public domain.
This is free and unencumbered software released into the public domain.
virtual void paint(MdiCubeViewport *vp, QPainter *painter)
Paint plot curve information onto the viewport.