File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
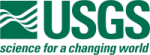 |
Isis 3 Programmer Reference
|
11 #include "tnt/tnt_array2d.h"
71 void Solve(
const double x[],
const double y[],
72 const double xp[],
const double yp[],
int n);
77 void Scale(
double scaleFactor);
void Compute(double x, double y)
Compute (xp,yp) given (x,y).
AMatrix Inverse() const
Returns the inverse Affine matrix.
TNT::Array2D< double > AMatrix
Affine Matrix.
double p_xp
x' value of the (x',y') coordinate
void Rotate(double rot)
Apply a translation to the current affine transform.
AMatrix p_matrix
Affine forward matrix.
AMatrix p_invmat
Affine inverse matrix.
void ComputeInverse(double xp, double yp)
Compute (x,y) given (xp,yp).
AMatrix Forward() const
Returns the forward Affine matrix.
AMatrix invert(const AMatrix &a) const
Compute the inverse of a matrix.
double x() const
Returns the computed x.
static AMatrix getIdentity()
Return an Affine identity matrix.
std::vector< double > Coefficients(int var)
Return the affine coeffients for the entered variable (1 or 2).
void Solve(const double x[], const double y[], const double xp[], const double yp[], int n)
Given a set of coordinate pairs (n >= 3), compute the affine transform that best fits the points.
void checkDims(const AMatrix &am) const
Checks affine matrix to ensure it is a 3x3 standard form transform.
double y() const
Returns the computed y.
double p_yp
y' value of the (x',y') coordinate
double p_x
x value of the (x,y) coordinate
void Translate(double tx, double ty)
Apply a translation to the current affine transform.
~Affine()
Destroys the Affine object.
double xp() const
Returns the computed x'.
Affine()
Constructs an Affine transform.
double yp() const
Returns the computed y'.
double p_y
y value of the (x,y) coordinate
void Identity()
Set the forward and inverse affine transform to the identity.
void Scale(double scaleFactor)
Apply a scale to the current affine transform.
This is free and unencumbered software released into the public domain.
std::vector< double > InverseCoefficients(int var)
Return the inverse affine coeffients for the entered variable (1 or 2).