Loading [MathJax]/jax/output/NativeMML/config.js
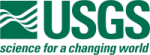 |
Isis 3 Programmer Reference
|
9 #include "SpecialPixel.h"
10 #include "IException.h"
12 #include "NormModel.h"
79 double emission,
double demincidence,
double dememission,
80 double dn,
double &albedo,
double &mult,
double &base) {
85 psurf = GetPhotoModel()->
CalcSurfAlbedo(phase, demincidence, dememission);
96 QString msg =
"Albedo math divide by zero error";
119 if(pharef < 0.0 || pharef >= 180.0) {
120 QString msg =
"Invalid value of normalization pharef [" +
137 if(incref < 0.0 || incref >= 90.0) {
138 QString msg =
"Invalid value of normalization incref [" +
155 if(emaref < 0.0 || emaref >= 90.0) {
156 QString msg =
"Invalid value of normalization emaref [" +
170 if(incmat < 0.0 || incmat >= 90.0) {
171 QString msg =
"Invalid value of normalization incmat [" +
double p_normIncref
The reference incidence angle.
@ Unknown
A type of error that cannot be classified as any of the other error types.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
double CalcSurfAlbedo(double pha, double inc, double ema)
Calculate the surface brightness using photometric angle information.
Container for cube-like labels.
double p_normThresh
Used to amplify variations in the input image.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
void SetNormPharef(const double pharef)
Set parameters needed for albedo normalization.
Albedo(Pvl &pvl, PhotoModel &pmodel)
Constructs an Albedo object.
@ Traverse
Search child objects.
virtual void NormModelAlgorithm(double pha, double inc, double ema, double dn, double &albedo, double &mult, double &base)
Performs the normalization.
Contains multiple PvlContainers.
double p_normAlbedo
The albedo.
double p_normIncmat
Incmat.
void SetNormIncref(const double incref)
Set the normalization function parameter.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
double p_normEmaref
The reference emission angle.
virtual void SetStandardConditions(bool standard)
Sets whether standard conditions will be used.
void SetNormAlbedo(const double albedo)
Set the normalization function parameter.
void SetNormEmaref(const double emaref)
Set the normalization function parameter.
void SetNormThresh(const double thresh)
Set the normalization function parameter.
void SetNormIncmat(const double incmat)
Set the normalization function parameter.
double p_normPharef
The reference phase angle.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....