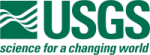 |
Isis 3 Programmer Reference
|
11 #include <QGlobalStatic>
105 *
this = *
this + angle2;
116 *
this = *
this - angle2;
127 return *
this * (double)value;
138 *
this = *
this * value;
149 return *
this / (double)value;
159 *
this = *
this / value;
187 return !(*
this == angle2);
198 return *
this < angle2 || *
this == angle2;
210 return *
this > angle2 || *
this == angle2;
248 virtual QString
toString(
bool includeUnits =
true)
const;
250 virtual double angle(
const Units& unit)
const;
262 QDebug operator<<(QDebug dbg,
const Isis::Angle &angleToPrint);
@ Degrees
Degrees are generally considered more human readable, 0-360 is one circle, however most math does not...
Angle operator*(double value) const
Multiply this angle by a double and return the resulting angle.
Angle operator-(const Angle &angle2) const
Subtract angle value from another and return the resulting angle.
virtual QString toString(bool includeUnits=true) const
Get the angle in human-readable form.
bool operator>(const Angle &angle2) const
Test if the other angle is greater than the current angle.
void operator/=(double value)
Divide this angle by a double and return the resulting angle.
double m_radians
The angle measure, always stored in radians.
bool operator>=(const Angle &angle2) const
Test if the other angle is greater than or equal to the current angle.
double unitWrapValue(const Units &unit) const
Return wrap value in desired units.
void operator+=(const Angle &angle2)
Add angle value to another as double and replace original.
Angle operator/(int value) const
Divide this angle by an integer and return the resulting angle.
bool operator<(const Angle &angle2) const
Test if the other angle is less than the current angle.
Angle operator*(int value) const
Multiply this angle by an integer and return the resulting angle.
bool operator==(const Angle &angle2) const
Test if another angle is equal to this angle.
Units
The set of usable angle measurement units.
Defines an angle and provides unit conversions.
void setRadians(double radians)
Set the angle in units of Radians.
void operator-=(const Angle &angle2)
Subtract angle value from another and set this instance to the resulting angle.
virtual ~Angle()
Destroys the angle object.
Angle operator/(double value) const
Divide this angle by a double.
bool isValid() const
This indicates whether we have a legitimate angle stored or are in an unset, or invalid,...
void operator*=(double value)
Multiply this angle by a double and set this instance to the resulting angle.
Angle()
Constructs a blank angle object which needs a value to be set in order to do any calculations.
static Angle fullRotation()
Makes an angle to represent a full rotation (0-360 or 0-2pi).
Angle operator+(const Angle &angle2) const
Add angle value to another.
double degrees() const
Get the angle in units of Degrees.
virtual void setAngle(const double &angle, const Units &unit)
Set angle value in desired units.
bool operator<=(const Angle &angle2) const
Test if the other angle is less than or equal to the current angle.
bool operator!=(const Angle &angle2) const
Test if another angle is not equal to this angle.
Angle & operator=(const Angle &angle2)
Assign angle object equal to another.
virtual double angle(const Units &unit) const
Return angle value in desired units.
void setDegrees(double degrees)
Set the angle in units of Degrees.
@ Radians
Radians are generally used in mathematical equations, 0-2*PI is one circle, however these are more di...
This is free and unencumbered software released into the public domain.
double radians() const
Convert an angle to a double.