Loading [MathJax]/jax/output/NativeMML/config.js
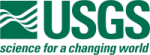 |
Isis 3 Programmer Reference
|
11 #include "BandSpinBox.h"
12 #include "IException.h"
23 list.push_back(QString::number(1));
43 disconnect(
this, 0, 0, 0);
49 .findGroup(
"Dimensions");
54 for(
int i = 1; i <=
p_bands; i++) {
55 list.push_back(QString::number(i));
57 p_map[
"BandNumber"] = list;
60 if(pvl.
findObject(
"IsisCube").hasGroup(
"BandBin")) {
62 .findGroup(
"BandBin");
63 for(
int i = 0; i < bandBin.
keywords(); i++) {
65 if(bandBin[i].size() ==
p_bands) {
66 for(
int j = 0; j < bandBin[i].size(); j++) {
67 list.push_back(QString(bandBin[i][j]));
69 QString bandBinName = bandBin[i].
name();
70 p_map[bandBinName] = list;
102 if(
p_map.contains(key)) {
124 if((key < 0) || (key >= (
int)
p_map.size())) {
142 if((val < 1) || (val >
p_bands)) {
143 std::cout <<
"BandSpinBox: Bad index in textFromValue" << std::endl;
144 return QString(
"Error");
151 std::cout <<
"BandSpinBox: Bad value for p_lastKey in textFromValue" << std::endl;
152 return QString(
"Error");
171 std::cout <<
"BandSpinBox: Bad text in valueFromText" << std::endl;
183 QFontMetrics fm(font());
186 for(
int i = minimum(); i <= maximum(); i++) {
187 w = qMax(w, fm.width(((
BandSpinBox *)
this)->textFromValue(i)));
190 QSize s = QSpinBox::sizeHint();
191 int neww = s.width() + w;
202 s.setWidth(neww + 5);
224 if(count == 0)
return QValidator::Invalid;
225 if(count > 0 && exact)
return QValidator::Acceptable;
226 return QValidator::Intermediate;
void setKey(QString key)
Sets the key to the provided key.
QStringList p_keys
List of all the keys.
Container for cube-like labels.
int valueFromText(const QString &text) const
gets the value (int) using p_map.
void setBandBin(Pvl &pvl, const QString &key="BandNumber")
Sets the band bin.
QString textFromValue(int val) const
Gets the text using p_map.
Contains multiple PvlContainers.
BandSpinBox(QWidget *parent=0)
BandSpinBox constructor.
QSize sizeHint() const
returns a size hint for the spin box
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
QString name() const
Returns the container name.
QMap< QString, QStringList > p_map
The maps the last key to all the keys.
@ Programmer
This error is for when a programmer made an API call that was illegal.
QString p_lastKey
The last key.
int p_bands
Number of bands.
int keywords() const
Returns the number of keywords contained in the PvlContainer.
QValidator::State validate(QString &input, int &pos) const
returns how valid the value from the spin box is.
QStringList BandBinKeys()
returns the list of keys.
This is free and unencumbered software released into the public domain.