File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
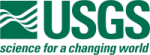 |
Isis 3 Programmer Reference
|
13 #include <tnt/tnt_array1d.h>
14 #include "CollectorMap.h"
15 #include "IException.h"
68 template <
typename TokenStore = QString>
92 CSVParser(
const QString &str,
const char &delimiter =
',',
93 bool keepEmptyParts =
true) {
94 parse(str, delimiter, keepEmptyParts);
137 int parse(
const QString &str,
const char &delimiter =
',',
138 bool keepEmptyParts =
true) {
140 str.split(delimiter, keepEmptyParts? QString::KeepEmptyParts : QString::SkipEmptyParts);
142 for(
int i = 0 ; i < tokens.size() ; i++) {
260 CSVReader(
const QString &csvfile,
bool header =
false,
int skip = 0,
262 const bool ignoreComments =
true);
287 return ((nrows < 0) ? 0 : nrows);
336 if(nskip >= 0)
_skip = nskip;
449 void read(
const QString &fname);
460 template <
typename T> TNT::Array1D<T>
convert(
const CSVAxis &data)
const;
494 std::istream &
load(std::istream &ifile);
531 template <
typename T>
533 TNT::Array1D<T> out(data.dim());
534 for(
int i = 0 ; i < data.dim() ; i++) {
CSVAxis getColumn(int index) const
Parse and return a column specified by index order.
Parser::TokenList CSVAxis
Row/Column token list.
int columns() const
Determine the number of columns in the input source.
bool isTableValid(const CSVTable &table) const
Indicates if all rows have the same number of columns.
void setComment(const bool ignore=true)
Allows the user to indicate comment disposition.
Reads strings and parses them into tokens separated by a delimiter character.
CSVAxis getRow(int index) const
Parse and return the requested row by index.
bool haveHeader() const
Returns true if a header is present in the input source.
void setHeader(const bool gotIt=true)
Allows the user to indicate header disposition.
void clear()
Discards all lines read from an input source.
TNT::Array1D< TokenType > TokenList
List of tokens.
virtual ~CSVParser()
Destructor.
void setDelimiter(const char &delimiter)
Set the delimiter character that separate tokens in the strings.
const TokenType & operator()(const int nth) const
Returns the nth token in the parsed string.
void setSkipEmptyParts()
Indicate multiple occurances of delimiters are one token.
CSVReader()
Default constructor for CSV reader.
friend std::istream & operator>>(std::istream &is, CSVReader &csv)
Input read operator for input stream sources.
std::vector< QString > CSVList
Input source line container.
CSVAxis getHeader() const
Retrieve the header from the input source if it exists.
TNT::Array1D< T > convert(const CSVAxis &data) const
Converts a row or column of data to the specified type.
CollectorMap< int, int > CSVColumnSummary
Column summary for all rows.
TNT::Array1D< CSVAxis > CSVTable
Table of all rows/columns.
bool _ignoreComments
Ignore comments on read.
bool keepEmptyParts() const
Returns true when preserving succesive tokens, false when they are treated as one token.
CSVParser(const QString &str, const char &delimiter=',', bool keepEmptyParts=true)
Constructor that parses strings according to given parameters.
std::istream & load(std::istream &ifile)
Reads all lines from the input stream until an EOF is encoutered.
TokenList result() const
Returns the list of tokens.
CSVTable getTable() const
Parse and return all rows and columns in a table array.
TNT::Array1D< int > CSVIntVector
Integer array def.
int _skip
Number of lines to skip.
CSVList _lines
List of lines from file.
int firstRowIndex() const
Computes the index of the first data.
void read(const QString &fname)
Reads the entire contents of a file for subsequent parsing.
CSVColumnSummary getColumnSummary(const CSVTable &table) const
Computes a row summary of the number of distinct columns in table.
void setKeepEmptyParts()
Indicate multiple occurances of delimiters are empty tokens.
int size() const
Reports the total number of lines read from the stream.
double toDouble(const QString &string)
Global function to convert from a string to a double.
void setSkip(int nskip)
Indicate the number of lines at the top of the source to skip to data.
Collector/container for arbitrary items.
bool _keepParts
Keep empty parts between delimiter.
bool _header
Indicates presences of header.
char getDelimiter() const
Reports the character used to delimit tokens in strings.
CSVParser< QString > Parser
Defines single line parser.
TokenList _elements
List of tokens parsed from string.
CSVParser()
Default constructor
CSV Parser seperates fields (tokens) from a string with a delimeter.
char _delimiter
Separator of values.
TNT::Array1D< double > CSVDblVector
Double array def.
int parse(const QString &str, const char &delimiter=',', bool keepEmptyParts=true)
Parser method accepting string, delimiter and multiple token handling.
int size() const
Returns the number of tokens in the parsed string.
virtual ~CSVReader()
Destructor (benign)
This is free and unencumbered software released into the public domain.
int rows() const
Reports the number of rows in the table.
TokenStore TokenType
Token storage type.
int getSkip() const
Reports the number of lines to skip.