File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
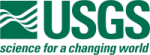 |
Isis 3 Programmer Reference
|
9 #include "ControlDisplayProperties.h"
14 #include <QColorDialog>
16 #include <QInputDialog>
19 #include <QXmlStreamWriter>
23 #include "XmlStackedHandlerReader.h"
74 emit supportAdded(prop);
111 while(red + green + blue < 300) {
113 green = rand() % 256;
117 return QColor(red, green, blue, 60);
121 void ControlDisplayProperties::save(QXmlStreamWriter &stream,
const Project *project,
123 stream.writeStartElement(
"displayProperties");
125 stream.writeAttribute(
"displayName",
displayName());
129 dataBuffer.open(QIODevice::ReadWrite);
130 QDataStream propsStream(&dataBuffer);
134 stream.writeCharacters(dataBuffer.data().toHex());
136 stream.writeEndElement();
176 foreach(display, displays) {
183 m_displayProperties = displayProperties;
187 bool ControlDisplayProperties::XmlHandler::startElement(
const QString &namespaceURI,
188 const QString &localName,
const QString &qName,
const QXmlAttributes &atts) {
189 if (XmlStackedHandler::startElement(namespaceURI, localName, qName, atts)) {
190 if (localName ==
"displayProperties") {
203 bool ControlDisplayProperties::XmlHandler::characters(
const QString &ch) {
206 return XmlStackedHandler::characters(ch);
210 bool ControlDisplayProperties::XmlHandler::endElement(
const QString &namespaceURI,
211 const QString &localName,
const QString &qName) {
212 if (localName ==
"displayProperties") {
213 QByteArray hexValues(m_hexData.toLatin1());
214 QDataStream valuesStream(QByteArray::fromHex(hexValues));
215 valuesStream >> *m_displayProperties->m_propertyValues;
218 return XmlStackedHandler::endElement(namespaceURI, localName, qName);
228 (*m_propertyValues)[prop] = value;
231 emit propertyChanged(
this);
247 QVariant callerData = caller->data();
This class is designed to serialize QColor in a human-readable form.
static QList< ControlDisplayProperties * > senderToData(QObject *sender)
This is for the slots that have a list of display properties as associated data.
@ None
Null display property for bit-flag purposes.
This is free and unencumbered software released into the public domain.
Property m_propertiesUsed
This indicated whether any widgets with this DisplayProperties is using a particular property.
static QColor randomColor()
Creates and returns a random color for the intial color of the footprint polygon.
File name manipulation and expansion.
virtual void pushContentHandler(XmlStackedHandler *newHandler)
Push a contentHandler and maybe continue parsing...
@ ShowLabel
True if the control net should show its display name (bool)
This is the GUI communication mechanism for cubes.
void toggleShowLabel()
Change the visibility of the display name.
@ Selected
The selection state of this control net (bool)
virtual ~ControlDisplayProperties()
destructor
Manage a stack of content handlers for reading XML files.
The main project for ipce.
void setSelected(bool)
Change the selected state associated with this cube.
ControlDisplayProperties(QString displayName, QObject *parent=NULL)
ControlDisplayProperties constructor.
bool supports(Property prop)
Support may come later, please make sure you are connected to the supportAdded signal.
QString displayName() const
Returns the display name.
QMap< int, QVariant > * m_propertyValues
This is a map from Property to value – the reason I use an int is so Qt knows how to serialize this Q...
void setShowLabel(bool)
Change the visibility of the display name associated with this cube.
void setColor(QColor newColor)
Change the color associated with this cube.
void addSupport(Property prop)
Call this with every property you support, otherwise they will not communicate properly between widge...
void setValue(Property prop, QVariant value)
This is the generic mutator for properties.
Property
This is a list of properties and actions that are possible.
QVariant getValue(Property prop) const
Get a property's associated data.
This is free and unencumbered software released into the public domain.