File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
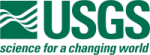 |
Isis 3 Programmer Reference
|
9 #include "ControlPointList.h"
13 #include "IException.h"
28 int size = list.size();
29 for(
int i = 0; i < size; i++) {
39 QString msg =
"Can't open or invalid file list [" +
61 int index = mqCpList.indexOf(QString(psCpId));
63 if(index == -1 || index >=
Size())
77 return mqCpList.size();
90 if(piIndex >= 0 && piIndex < size) {
91 return (mqCpList.value(piIndex));
95 QString msg =
"Index [" + num +
"] is invalid";
109 return mqCpList.indexOf(QString(psCpId));
112 QString msg =
"Requested control point id [" + psCpId +
"] ";
113 msg +=
"does not exist in the list";
127 QString sPointsNotFound =
"";
129 for(
int i = 0; i < size; i++) {
132 sPointsNotFound +=
", ";
134 sPointsNotFound += mqCpList.value(i);
void RegisterStatistics(Pvl &pcPvlLog)
Register invalid control point and calculate the valid & invalid point count.
QVector< bool > mbFound
holds one to one correspondence with "mqCpList" on whether the point was valid
A single keyword-value pair.
File name manipulation and expansion.
Container for cube-like labels.
QString ControlPointId(int piIndex)
Return a control point id given an index.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
int Size() const
How many control points in the list.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
virtual ~ControlPointList()
Destructor.
bool HasControlPoint(const QString &psCpId)
Determines whether or not the requested control point id exists in the list.
@ Programmer
This error is for when a programmer made an API call that was illegal.
int ControlPointIndex(const QString &psCpId)
return a list index given a control point id
ControlPointList(const FileName &psFileName)
Creates a ControlPointList from a list of control point ids'.
Internalizes a list of files.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....