File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
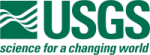 |
Isis 3 Programmer Reference
|
7 #include "CubeAttribute.h"
14 #include "IException.h"
15 #include "Preference.h"
16 #include "SpecialPixel.h"
83 vector<QString> result;
85 QString str =
toString().remove(QRegExp(
"^\\+"));
87 QStringList strSplit = str.split(
",", QString::SkipEmptyParts);
88 foreach (QString commaTok, strSplit) {
90 if (commaTok.contains(
'-')) {
92 int start =
toInt(commaTok.mid(0, commaTok.indexOf(
"-")));
93 int end =
toInt(commaTok.mid(commaTok.indexOf(
"-") + 1));
95 direction = (start <= end) ? 1 : -1;
97 for (
int band = start; band != end; band += direction) {
104 result.push_back(commaTok);
122 bool CubeAttributeInput::isBandRange(QString attribute)
const {
123 return QRegExp(
"[0-9,\\-]+").exactMatch(attribute);
129 for (
unsigned int i = 0; i <
bands.size(); i++) {
140 QList<bool (CubeAttributeInput::*)(QString)
const> CubeAttributeInput::testers() {
141 QList<bool (CubeAttributeInput::*)(QString)
const> result;
143 result.append(&CubeAttributeInput::isBandRange);
170 if (pixelTypeAtts.isEmpty() || pixelTypeAtts.last() ==
"PROPAGATE")
178 return attributeList(&CubeAttributeOutput::isRange).isEmpty();
272 if (!formatList.isEmpty()) {
273 QString formatString = formatList.last();
275 if (formatString ==
"BSQ" || formatString ==
"BANDSEQUENTIAL")
290 &CubeAttributeOutput::isFileFormat);
295 double result =
Null;
298 QString range =
attributeList(&CubeAttributeOutput::isRange).last();
301 if (rangeList.count() == 2 && rangeList.first() !=
"")
302 result =
toDouble(rangeList.first());
310 double result =
Null;
313 QString range =
attributeList(&CubeAttributeOutput::isRange).last();
316 if (rangeList.count() == 2 && rangeList.last() !=
"")
317 result =
toDouble(rangeList.last());
364 QString pixelTypeAtt =
attributeList(&CubeAttributeOutput::isPixelType).last();
366 if (pixelTypeAtt ==
"8BIT" || pixelTypeAtt ==
"8-BIT" || pixelTypeAtt ==
"UNSIGNEDBYTE") {
367 result = UnsignedByte;
369 else if (pixelTypeAtt ==
"16BIT" || pixelTypeAtt ==
"16-BIT" || pixelTypeAtt ==
"SIGNEDWORD") {
372 else if (pixelTypeAtt ==
"16UBIT" || pixelTypeAtt ==
"16-UBIT" || pixelTypeAtt ==
"UNSIGNEDWORD") {
373 result = UnsignedWord;
375 else if (pixelTypeAtt ==
"32BIT" || pixelTypeAtt ==
"32-BIT" || pixelTypeAtt ==
"REAL") {
378 else if (pixelTypeAtt ==
"32UINT" || pixelTypeAtt ==
"32-UINT" || pixelTypeAtt ==
"UNSIGNEDINTEGER") {
379 result = UnsignedInteger;
381 else if (pixelTypeAtt ==
"32INT" || pixelTypeAtt ==
"32-INT" || pixelTypeAtt ==
"SIGNEDINTEGER") {
382 result = SignedInteger;
404 if (!labelAttachmentAtts.isEmpty()) {
405 QString labelAttachmentAtt = labelAttachmentAtts.last();
407 if (labelAttachmentAtt ==
"DETACHED")
409 else if (labelAttachmentAtt ==
"EXTERNAL")
417 bool CubeAttributeOutput::isByteOrder(QString attribute)
const {
418 return QRegExp(
"(M|L)SB").exactMatch(attribute);
422 bool CubeAttributeOutput::isFileFormat(QString attribute)
const {
423 return QRegExp(
"(BANDSEQUENTIAL|BSQ|TILE)").exactMatch(attribute);
427 bool CubeAttributeOutput::isLabelAttachment(QString attribute)
const {
428 return QRegExp(
"(ATTACHED|DETACHED|EXTERNAL)").exactMatch(attribute);
432 bool CubeAttributeOutput::isPixelType(QString attribute)
const {
433 QString expressions =
"(8-?BIT|16-?BIT|32-?BIT|UNSIGNEDBYTE|SIGNEDWORD|UNSIGNEDWORD|REAL";
434 expressions +=
"|32-?UINT|32-?INT|UNSIGNEDINTEGER|SIGNEDINTEGER)";
435 return QRegExp(expressions).exactMatch(attribute);
440 bool CubeAttributeOutput::isRange(QString attribute)
const {
441 return QRegExp(
"[\\-+E0-9.]*:[\\-+E0-9.]*").exactMatch(attribute);
446 QString result =
"Tile";
449 result =
"BandSequential";
460 if (!byteOrderAtts.isEmpty()) {
461 QString byteOrderAtt = byteOrderAtts.last();
462 result = (byteOrderAtt ==
"LSB")? Lsb : Msb;
476 &CubeAttributeOutput::isByteOrder);
483 result.append(&CubeAttributeOutput::isByteOrder);
484 result.append(&CubeAttributeOutput::isFileFormat);
485 result.append(&CubeAttributeOutput::isLabelAttachment);
486 result.append(&CubeAttributeOutput::isPixelType);
487 result.append(&CubeAttributeOutput::isRange);
Format
These are the possible storage formats of ISIS cubes.
void setMaximum(double max)
Set the output cube attribute maximum.
@ DetachedLabel
The input label is in a separate data file from the image.
void setPixelType(PixelType type)
Set the pixel type to that given by the parameter.
This is free and unencumbered software released into the public domain.
QString LabelAttachmentName(LabelAttachment labelType)
Return the string representation of the contents of a variable of type LabelAttachment.
QStringList attributeList(bool(ChildClass::*tester)(QString) const) const
Get a list of attributes that the tester returns true on.
bool IsLsb()
Return true if this host is an LSB first machine and false if it is not.
File name manipulation and expansion.
void setAttribute(QString newValue, bool(ChildClass::*tester)(QString) const)
Set the attribute(s) for which tester returns true to newValue.
Manipulate and parse attributes of output cube filenames.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
bool IsSpecial(const double d)
Returns if the input pixel is special.
void setFileFormat(Cube::Format fmt)
Set the format to the fmt parameter.
ByteOrder
Tests the current architecture for byte order.
void setAttributes(const FileName &fileName)
Replaces the current attributes with the attributes in the given file name.
@ AttachedLabel
The input label is embedded in the image file.
void setByteOrder(ByteOrder order)
Set the order according to the parameter order.
LabelAttachment
Input cube label type tracker.
int toInt(const QString &string)
Global function to convert from a string to an integer.
@ Tile
Cubes are stored in tile format, that is the order of the pixels in the file (on disk) is BSQ within ...
PixelType pixelType() const
Return the pixel type as an Isis::PixelType.
bool propagateMinimumMaximum() const
Return true if the min/max are to be propagated from an input cube.
QString byteOrderString() const
Return the byte order as a string.
QString fileFormatString() const
Return the file format as a string.
CubeAttributeOutput()
Constructs an empty CubeAttributeOutput.
~CubeAttributeOutput()
Destroys the object.
const double Null
Value for an Isis Null pixel.
@ Bsq
Cubes are stored in band-sequential format, that is the order of the pixels in the file (on disk) is:
PixelType
Enumerations for Isis Pixel Types.
double toDouble(const QString &string)
Global function to convert from a string to a double.
Namespace for the standard library.
ByteOrder byteOrder() const
Return the byte order as an Isis::ByteOrder.
void setLabelAttachment(LabelAttachment attachment)
Set the label attachment type to the parameter value.
QString PixelTypeName(Isis::PixelType pixelType)
Returns string name of PixelType enumeration entered as input parameter.
double maximum() const
Return the output cube attribute maximum.
double minimum() const
Return the output cube attribute minimum.
Cube::Format fileFormat() const
Return the file format an Cube::Format.
Parent class for CubeAttributeInput and CubeAttributeOutput.
bool propagatePixelType() const
Return true if the pixel type is to be propagated from an input cube.
@ ExternalLabel
The label is pointing to an external DN file - the label is also external to the data.
void setMinimum(double min)
Set the output cube attribute minimum.
This is free and unencumbered software released into the public domain.
QString toString() const
Return a string-representation of this cube attributes.