File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
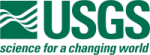 |
Isis 3 Programmer Reference
|
8 #ifndef CubeIoHandler_h
9 #define CubeIoHandler_h
12 #include <QThreadPool>
14 #include "Constants.h"
16 #include "PixelType.h"
21 template <
typename A>
class QList;
22 template <
typename A,
typename B>
class QMap;
23 template <
typename A,
typename B>
struct QPair;
27 class CubeCachingAlgorithm;
112 const Pvl &label,
bool alreadyOnDisk);
119 void clearCache(
bool blockForWriteCache =
true)
const;
147 void setChunkSizes(
int numSamples,
int numLines,
int numBands);
237 int startLine,
int numLines,
238 int startBand,
int numBands)
const;
241 const Buffer &cube2,
int &startX,
int &startY,
int &startZ,
242 int &endX,
int &endY,
int &endZ)
const;
253 int &startSample,
int &startLine,
int &startBand,
254 int &endSample,
int &endLine,
int &endBand)
const;
259 const Buffer &justRequested)
const;
QThreadPool * m_ioThreadPool
This contains threads for doing cube writes (and later maybe cube reads).
A section of raw data on the disk.
int m_samplesInChunk
The number of samples in a cube chunk.
void addCachingAlgorithm(CubeCachingAlgorithm *algorithm)
This will add the given caching algorithm to the list of attempted caching algorithms.
int m_numLines
The number of lines in the cube.
double m_base
The additive offset of the data on disk.
QList< RawCubeChunk * > * m_lastProcessByLineChunks
This is an optimization for process by line.
This is free and unencumbered software released into the public domain.
QByteArray * m_nullChunkData
A raw cube chunk's data when it was all NULL's. Used for speed.
CubeIoHandler & operator=(const CubeIoHandler &other)
Disallow assignments of this object.
int getBandCountInChunk() const
EndianSwapper * m_byteSwapper
A helper that swaps byte order to and from file order.
virtual void updateLabels(Pvl &labels)=0
Function to update the labels with a Pvl object.
PixelType pixelType() const
CubeIoHandler * m_ioHandler
The IO Handler instance to put the buffers into.
BigInt getBytesPerChunk() const
void setVirtualBands(const QList< int > *virtualBandList)
This changes the virtual band list.
void writeIntoRaw(const Buffer &buffer, RawCubeChunk &output, int index) const
Write the intersecting area of the buffer into the chunk.
QFile * m_dataFile
The file containing cube data.
QMutex * m_writeThreadMutex
This enables us to block while the write thread is working.
Container for cube-like labels.
int m_numBands
The number of physical bands in the cube.
BufferToChunkWriter(const BufferToChunkWriter &other)
This is disabled.
int getSampleCountInChunk() const
double m_multiplier
The multiplicative factor of the data on disk.
volatile int m_idealFlushSize
Ideal write cache flush size.
ByteOrder
Tests the current architecture for byte order.
Buffer for reading and writing cube data.
CubeIoHandler(QFile *dataFile, const QList< int > *virtualBandList, const Pvl &label, bool alreadyOnDisk)
Creates a new CubeIoHandler using a RegionalCachingAlgorithm.
void freeChunk(RawCubeChunk *chunkToFree) const
If the chunk is dirty, then we write it to disk.
void getChunkPlacement(int chunkIndex, int &startSample, int &startLine, int &startBand, int &endSample, int &endLine, int &endBand) const
Get the X/Y/Z (Sample,Line,Band) range of the chunk at the given index.
void run()
This is the asynchronous computation.
void blockUntilThreadPoolEmpty() const
This blocks (doesn't return) until the number of active runnables in the thread pool goes to 0.
RawCubeChunk * getChunk(int chunkIndex, bool allocateIfNecessary) const
Retrieve the cached chunk at the given chunk index, if there is one.
RawCubeChunk * getNullChunk(int chunkIndex) const
This creates a chunk filled with NULLs whose placement is at chunkIndex's position.
BigInt getDataStartByte() const
virtual ~CubeIoHandler()
Cleans up all allocated memory.
int getChunkCountInBandDimension() const
QMutex * dataFileMutex()
Get the mutex that this IO handler is using around I/Os on the given data file.
This is the parent of the caching algorithms.
Handles converting buffers to and from disk.
long long int BigInt
Big int.
QMap< int, bool > * m_dataIsOnDiskMap
The map from chunk index to on-disk status, all true if not allocated.
int getChunkIndex(const RawCubeChunk &) const
Given a chunk, what's its index in the file.
virtual void writeRaw(const RawCubeChunk &chunkToWrite)=0
This needs to write the chunkToWrite directly to disk with no modifications to the data itself.
int m_linesInChunk
The number of lines in a cube chunk.
void writeIntoDouble(const RawCubeChunk &chunk, Buffer &output, int startIndex) const
Write the intersecting area of the chunk into the buffer.
BufferToChunkWriter(CubeIoHandler *ioHandler, QList< Buffer * > buffersToWrite)
Create a BufferToChunkWriter which is designed to asynchronously move the given buffers into the cube...
int getChunkCountInSampleDimension() const
QTime * m_timer
Used to calculate the lifetime of an instance of this class.
int m_numSamples
The number of samples in the cube.
int m_consecutiveOverflowCount
How many times the write cache has overflown in a row.
void findIntersection(const RawCubeChunk &cube1, const Buffer &cube2, int &startX, int &startY, int &startZ, int &endX, int &endY, int &endZ) const
Find the intersection between the buffer area and the cube chunk.
ByteOrder m_byteOrder
The byte order (endianness) of the data on disk.
PixelType
Enumerations for Isis Pixel Types.
void read(Buffer &bufferToFill) const
Read cube data from disk into the buffer.
int m_bandsInChunk
The number of physical bands in a cube chunk.
QList< int > * m_virtualBands
Converts from virtual band to physical band.
void synchronousWrite(const Buffer &bufferToWrite)
This method takes the given buffer and synchronously puts it into the Cube's cache.
This is free and unencumbered software released into the public domain.
~BufferToChunkWriter()
We're done writing our buffers into the cube, clean up.
void minimizeCache(const QList< RawCubeChunk * > &justUsed, const Buffer &justRequested) const
Apply the caching algorithms and get rid of excess cube data in memory.
static bool bufferLessThan(Buffer *const &lhs, Buffer *const &rhs)
This is used for sorting buffers into the most efficient write order.
QMap< int, RawCubeChunk * > * m_rawData
The map from chunk index to chunk for cached data.
void flushWriteCache(bool force=false) const
This attempts to write the so-far-unwritten buffers from m_writeCache into the cube's RawCubeChunk ca...
void clearCache(bool blockForWriteCache=true) const
Free all cube chunks (cached cube data) from memory and write them to disk.
QPair< QMutex *, QList< Buffer * > > * m_writeCache
These are the buffers we need to write to raw cube chunks.
QPair< QList< RawCubeChunk * >, QList< int > > findCubeChunks(int startSample, int numSamples, int startLine, int numLines, int startBand, int numBands) const
Get the cube chunks that correspond to the given cube area.
void write(const Buffer &bufferToWrite)
Write buffer data into the cube data on disk.
This is free and unencumbered software released into the public domain.
This class is designed to handle write() asynchronously.
int getChunkCountInLineDimension() const
void writeNullDataToDisk() const
Write all NULL cube chunks that have not yet been accessed to disk.
BufferToChunkWriter & operator=(const BufferToChunkWriter &rhs)
This is disabled.
PixelType m_pixelType
The format of each DN in the cube.
int getChunkCount() const
void setChunkSizes(int numSamples, int numLines, int numBands)
This should be called once from the child constructor.
CubeIoHandler(const CubeIoHandler &other)
Disallow copying of this object.
virtual void readRaw(RawCubeChunk &chunkToFill)=0
This needs to populate the chunkToFill with unswapped raw bytes from the disk.
BigInt m_startByte
The start byte of the cube data.
QList< CubeCachingAlgorithm * > * m_cachingAlgorithms
The caching algorithms to use, in order of priority.
QList< Buffer * > * m_buffersToWrite
The buffers that need pushed into the IO handler; we own these.
bool m_useOptimizedCubeWrite
This is true if the Isis preference for the cube write thread is optimized.
int getLineCountInChunk() const
bool m_lastOperationWasWrite
If the last operation was a write then we need to flush the cache when reading.
This is free and unencumbered software released into the public domain.
BigInt getDataSize() const