Loading [MathJax]/jax/output/NativeMML/config.js
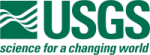 |
Isis 3 Programmer Reference
|
14 using std::ostringstream;
19 #include "PvlObject.h"
46 DbAccess::DbAccess(
const QString &dbaccFile,
47 const QString &defProfileName) :
DbProfile(
"Database"),
48 _defProfileName(defProfileName), _profiles() {
62 DbAccess::DbAccess(
PvlObject &pvl,
const QString &defProfileName) :
63 DbProfile(
"Database"), _defProfileName(defProfileName),
93 QString defName(name);
94 if(defName.isEmpty()) {
193 else if(
exists(
"DefaultProfile")) {
194 return (
value(
"DefaultProfile"));
PvlGroupIterator endGroup()
Returns the ending group index.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
QList< Isis::PvlGroup >::iterator PvlGroupIterator
The counter for groups.
Contains Pvl Groups and Pvl Objects.
ProfileList _profiles
List of profiles.
QString Name() const
Returns the name of this property.
bool exists(const QString &key) const
Checks for the existance of a keyword.
T & getNth(int nth)
Returns the nth value in the collection.
T & get(const K &key)
Returns the value associated with the name provided.
Container for cube-like labels.
QString _defProfileName
Name of default profile.
QString getDefaultProfileName() const
Determine the name of the default profile.
bool exists(const K &key) const
Checks the existance of a particular key in the list.
void load(const QString &filename)
Loads a Database access configuration file.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
void loadkeys(PvlContainer &pvl)
Loads DbProfile keys from the given Pvl construct.
A DbProfile is a container for access parameters to a database.
void add(const K &key, const T &value)
Adds the element to the list.
PvlGroupIterator beginGroup()
Returns the beginning group index.
const DbProfile getProfile(const QString &name="") const
Retrieves the specified access profile.
QString value(const QString &key, int nth=0) const
Returns the specified value for the given keyword.
This is free and unencumbered software released into the public domain.