File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
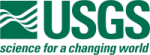 |
Isis 3 Programmer Reference
|
7 #include "GisGeometry.h"
11 #include <QScopedPointer>
19 #include "IException.h"
20 #include "ImagePolygon.h"
21 #include "GisTopology.h"
22 #include "SpecialPixel.h"
33 GisGeometry::GisGeometry() : m_type(None), m_geom(0), m_preparedGeom(0) {
51 m_type(GeosGis), m_geom(0), m_preparedGeom(0){
86 m_type(t), m_geom(0), m_preparedGeom(0) {
101 QString(
"Unknown GIS type given [%1]").arg(
typeToString(t)),
165 if (
this != &geom ) {
218 return (1 == GEOSisValid(this->
m_geom));
228 QString result =
"Not defined!";
231 char *reason = GEOSisValidReason(this->
m_geom);
257 QString gtype = gstrType.toLower();
258 if (
"wkt" == gtype)
return (
WKT);
259 if (
"wkb" == gtype)
return (
WKB);
260 if (
"cube" == gtype)
return (
IsisCube);
261 if (
"isiscube" == gtype)
return (
IsisCube);
262 if (
"geometry" == gtype)
return (
GeosGis);
263 if (
"geosgis" == gtype)
return (
GeosGis);
264 if (
"gis" == gtype)
return (
GeosGis);
265 if (
"geos" == gtype)
return (
GeosGis);
324 QScopedPointer<GisGeometry> geom(
new GisGeometry());
329 return (geom.take());
342 return (1 == GEOSisEmpty(this->
m_geom));
365 double gisArea = 0.0;
366 result = GEOSArea(this->
m_geom, &gisArea);
388 double gisLength = 0.0;
389 result = GEOSLength(this->
m_geom, &gisLength);
430 if (!
isValid() ) {
return (0); }
432 int ngeoms = GEOSGetNumGeometries(
m_geom);
434 for (
int i = 0 ; i < ngeoms ; i++) {
435 npoints += GEOSGetNumCoordinates(GEOSGetGeometryN(
m_geom, i));
467 return (1 == result);
494 return (1 == result);
520 return (1 == result);
547 return (1 == result);
567 return ( 1 == result );
596 if (this->
area() == 0) {
600 QScopedPointer<GisGeometry> inCommon(
intersection(target));
601 double ratio = inCommon->area() / this->
area();
624 GEOSGeometry *geom = GEOSEnvelope(
m_geom);
641 GEOSGeometry *geom = GEOSConvexHull(
m_geom);
663 GEOSGeometry *geom = GEOSTopologyPreserveSimplify(
m_geom, tolerance);
724 xlongitude = ylatitude =
Null;
729 GEOSGeometry *center = GEOSGetCentroid(
m_geom);
731 GEOSGeomGetX(center, &xlongitude);
732 GEOSGeomGetY(center, &ylatitude);
751 GEOSGeometry *center = GEOSGetCentroid(
m_geom);
764 #if defined(DISABLE_PREPARED_GEOMETRY)
781 GEOSCoordSequence *point = GEOSCoordSeq_create(1, 2);
782 GEOSCoordSeq_setX(point, 0, x);
783 GEOSCoordSeq_setY(point, 0, y);
785 return (GEOSGeom_createPoint(point));
798 QString polyStr = QString::fromStdString(myGis.
polyStr());
int points() const
Get number of points in geometry.
@ IsisCube
An ISIS Cube is used to create the geometry.
const GEOSPreparedGeometry * preparedGeometry(const GEOSGeometry *geom) const
Gets a GEOSPreparedGeometry from the given GEOSGeometry.
bool overlaps(const GisGeometry &target) const
Test for overlapping geometries.
GisGeometry * envelope() const
Computes the envelope or bounding box of this geometry.
This class models GIS topology.
double area() const
Computes the area of a geometry.
GisGeometry * centroid() const
Computes the centroid of the geometry and returns it as a new geometry.
Type m_type
Geometry type of GIS source.
GisGeometry()
Fundamental constructor of an empty object.
@ WKT
The GEOS library WKT reader is used to create the geometry.
ImagePolygon readFootprint() const
Read the footprint polygon for the Cube.
std::string polyStr() const
Return a geos Multipolygon.
QString isValidReason() const
Returns a string describing reason for invalid geometry.
GisGeometry * intersection(const GisGeometry &geom) const
Computes the intersection of two geometries.
Type type() const
Returns the type (origin) of the geometry.
const GEOSGeometry * geometry() const
Returns the GEOSGeometry object to extend functionality.
bool isEmpty() const
Tests for a defined but empty geometry.
GEOSPreparedGeometry const * makePrepared(const GEOSGeometry *geom) const
Creates a prepared geometry of current geometry.
Create cube polygons, read/write polygons to blobs.
void setGeometry(GEOSGeometry *geom)
Set the geometry directly taking ownership.
GEOSGeometry * clone(const GEOSGeometry *geom) const
Clones the given GEOSGeometry pointer.
Encapsulation class provides support for GEOS-C API.
@ GeosGis
GEOS GIS. A geometry object cannot be created with this geometry type.
bool isDefined() const
Determines if the current geometry is valid.
GisGeometry * clone() const
Clones the contents of this geometry to a new instance.
bool equals(const GisGeometry &target) const
Test if target and this geometry are equal.
GEOSGeometry * geomFromWKT(const QString &wkt)
Reads in the geometry from the given well-known text formatted string.
static GisTopology * instance()
Gets the singleton instance of this class.
GEOSGeometry * geomFromWKB(const QString &wkb)
Reads in the geometry from the given well-known binary formatted string.
bool contains(const GisGeometry &target) const
Test if the target geometry is contained within this geometry.
const GEOSPreparedGeometry * preparedGeometry() const
Returns special GEOS prepared geometry if it exists.
void destroy(GEOSGeometry *geom) const
Destroys the given GEOS geometry.
GisGeometry * convexHull() const
Computes the convex hull of the geometry.
GisGeometry * g_union(const GisGeometry &geom) const
Computes the union of two geometries.
GisGeometry * simplify(const double &tolerance) const
Simplify complex or overdetermined geoemtry.
@ None
No geometry. A geometry object cannot be created with this geometry type.
IO Handler for Isis Cubes.
GEOSPreparedGeometry const * m_preparedGeom
A prepared geometry from the GEOS library.
GEOSGeometry * makePoint(const double x, const double y) const
Create a point geometry.
@ WKB
The GEOS library WKB reader is used to create the geometry.
const double Null
Value for an Isis Null pixel.
bool intersects(const GisGeometry &target) const
Computes a new geometry from the intersection of the two geomtries.
static QString typeToString(const Type &type)
Returns the type of the Geometry as a QString.
GEOSGeometry * fromCube(Cube &cube) const
Reads Polygon from ISIS Cube and returns geometry from contents.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
double intersectRatio(const GisGeometry &geom) const
Computes intersect ratio between two geometries.
GEOSGeometry * m_geom
Pointer to GEOS-C opaque structure.
double length() const
Computes the length of a geometry.
void destroy()
Destroys the GEOS elements of this geometry object.
double distance(const GisGeometry &target) const
Computes the distance between two geometries.
bool disjoint(const GisGeometry &target) const
Tests for disjoint geometries.
bool isValid() const
Determines validity of the geometry contained in this object.
void open(const QString &cfile, QString access="r")
This method will open an isis cube for reading or reading/writing.
Type
Source type of the geometry.
GisGeometry & operator=(GisGeometry const &geom)
Assignment operator for GISGeomtries.
virtual ~GisGeometry()
Destructor.
This is free and unencumbered software released into the public domain.