File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
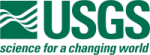 |
Isis 3 Programmer Reference
|
6 #include <QColorDialog>
8 #include <QInputDialog>
11 #include <QXmlStreamWriter>
15 #include "XmlStackedHandlerReader.h"
101 emit supportAdded(prop);
139 while(red + green + blue < 300) {
141 green = rand() % 256;
145 return QColor(red, green, blue, 60);
157 stream.writeStartElement(
"displayProperties");
159 stream.writeAttribute(
"displayName",
displayName());
163 dataBuffer.open(QIODevice::ReadWrite);
164 QDataStream propsStream(&dataBuffer);
168 stream.writeCharacters(dataBuffer.data().toHex());
170 stream.writeEndElement();
213 foreach(display, displays) {
244 const QString &localName,
const QString &qName,
const QXmlAttributes &atts) {
245 if (XmlStackedHandler::startElement(namespaceURI, localName, qName, atts)) {
246 if (localName ==
"displayProperties") {
272 return XmlStackedHandler::characters(ch);
288 const QString &localName,
const QString &qName) {
289 if (localName ==
"displayProperties") {
290 QByteArray hexValues(m_hexData.toLatin1());
291 QDataStream valuesStream(QByteArray::fromHex(hexValues));
292 valuesStream >> *m_displayProperties->m_propertyValues;
295 return XmlStackedHandler::endElement(namespaceURI, localName, qName);
309 (*m_propertyValues)[prop] = value;
312 emit propertyChanged(
this);
329 QVariant callerData = caller->data();
@ ShowLabel
True if the control net should show its display name (bool)
This class is designed to serialize QColor in a human-readable form.
virtual ~GuiCameraDisplayProperties()
destructor
void setShowLabel(bool)
Change the visibility of the display name associated with this target.
Property m_propertiesUsed
This indicates whether any widgets with this DisplayProperties is using a particular property.
void setSelected(bool)
Change the selected state associated with this target.
This is free and unencumbered software released into the public domain.
File name manipulation and expansion.
virtual void pushContentHandler(XmlStackedHandler *newHandler)
Push a contentHandler and maybe continue parsing...
bool supports(Property prop)
Support may come later, please make sure you are connected to the supportAdded signal.
void setValue(Property prop, QVariant value)
This is the generic mutator for properties.
void save(QXmlStreamWriter &stream, const Project *project, FileName newProjectRoot) const
Write the Gui Camera Display Properties out to an XML file.
Property
This is a list of properties and actions that are possible.
static QColor randomColor()
Creates and returns a random color for the intial color of the footprint polygon.
static QList< GuiCameraDisplayProperties * > senderToData(QObject *sender)
This is for the slots that have a list of display properties as associated data.
void toggleShowLabel()
Change the visibility of the display name.
Process a GuiCameraDisplayProperties in a stack-oriented way.
Manage a stack of content handlers for reading XML files.
The main project for ipce.
void addSupport(Property prop)
Call this with every property you support, otherwise they will not communicate properly between widge...
@ Selected
The selection state of this control net (bool)
QVariant getValue(Property prop) const
Get a property's associated data.
virtual bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts)
The XML reader invokes this method at the start of every element in the XML document.
QString displayName() const
Returns the display name.
@ None
Null display property for bit-flag purposes.
GuiCameraDisplayProperties(QString displayName, QObject *parent=NULL)
GuiCameraDisplayProperties constructor.
QMap< int, QVariant > * m_propertyValues
This is a map from Property to value – the reason I use an int is so Qt knows how to serialize this Q...
virtual bool characters(const QString &ch)
This implementation of a virtual function calls QXmlDefaultHandler::characters(QString &ch) which in ...
XmlHandler(GuiCameraDisplayProperties *displayProperties)
Sets the GuiCameraDisplayProperties variable pointer.
void setColor(QColor newColor)
Change the color associated with this target.
virtual bool endElement(const QString &namespaceURI, const QString &localName, const QString &qName)
The XML reader invokes this method at the end of every element in the XML document.
The GUI communication mechanism for target body objects.
GuiCameraDisplayProperties * m_displayProperties
An internal pointer to GuiCameraDisplayProperties object.
This is free and unencumbered software released into the public domain.