File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
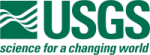 |
Isis 3 Programmer Reference
|
Go to the documentation of this file.
23 #include "GuiCameraList.h"
30 #include <QInputDialog>
32 #include <QProgressDialog>
33 #include <QtConcurrentMap>
34 #include <QXmlStreamWriter>
38 #include "IException.h"
41 #include "XmlStackedHandlerReader.h"
158 bool countChanging = count();
363 if (count() != other.count()) {
496 bool countChanging = (rhs.count() != count());
515 bool countChanging = (rhs.count() != count());
897 const QString &qName,
const QXmlAttributes &atts) {
898 if (XmlStackedHandler::startElement(namespaceURI, localName, qName, atts)) {
899 if (localName ==
"GuiCameraList") {
900 QString
name = atts.value(
"name");
901 QString
path = atts.value(
"path");
903 if (!
name.isEmpty()) {
904 m_GuiCameraList->setName(
name);
907 if (!
path.isEmpty()) {
908 m_GuiCameraList->setPath(
path);
911 else if (localName ==
"target") {
935 const QString &qName) {
936 if (localName ==
"GuiCameraList") {
937 XmlHandler handler(m_GuiCameraList, m_project);
941 reader.setErrorHandler(&handler);
943 QString GuiCameraListXmlPath = m_project->targetBodyRoot() +
"/" + m_GuiCameraList->path() +
945 QFile file(GuiCameraListXmlPath);
947 if (!file.open(QFile::ReadOnly)) {
949 QString(
"Unable to open [%1] with read access")
950 .arg(GuiCameraListXmlPath),
954 QXmlInputSource xmlInputSource(&file);
955 if (!reader.parse(xmlInputSource))
957 tr(
"Failed to open target body list XML [%1]").arg(GuiCameraListXmlPath),
961 return XmlStackedHandler::endElement(namespaceURI, localName, qName);
bool removeOne(GuiCameraQsp const &value)
Removes the first occurance of a GuiCamera from the list.
virtual bool endElement(const QString &namespaceURI, const QString &localName, const QString &qName)
Handle an XML end element.
XmlHandler(GuiCameraList *GuiCameraList, Project *project)
Change the visibility of the display name.
@ Io
A type of error that occurred when performing an actual I/O operation.
int removeAll(GuiCameraQsp const &value)
Removes all occurances of a GuiCamera from the list.
void swap(QList< GuiCameraQsp > &other)
Swaps the list with another GuiCameraList.
iterator erase(iterator pos)
Removes the GuiCamera associated with an iterator.
File name manipulation and expansion.
virtual void pushContentHandler(XmlStackedHandler *newHandler)
Push a contentHandler and maybe continue parsing...
void setPath(QString newPath)
Set the relative path (from the project root) to this gui camera list's folder.
void clear()
Clears the list.
GuiCameraQsp takeAt(int i)
Removes and returns the GuiCamera at a specific index.
Manage a stack of content handlers for reading XML files.
void prepend(GuiCameraQsp const &value)
Inserts a GuiCamera at the beginning of the list.
The main project for ipce.
void push_front(GuiCameraQsp const &value)
Inserts a GuiCamera at the front of the list.
GuiCameraQsp takeFirst()
Removes and returns the first GuiCamera in the list.
GuiCameraList & operator<<(const QList< GuiCameraQsp > &other)
Appends another GuiVameraList to the list and returns a reference to this.
QString m_name
This functor is used for copying the GuiCamera objects between two projects quickly.
GuiCameraList & operator=(const QList< GuiCameraQsp > &rhs)
Assigns a list of GuiCameras to the list.
GuiCameraList(QString name, QString path, QObject *parent=NULL)
Create an gui camera list from a gui camera list name and path (does not read GuiCamera objects).
GuiCameraQsp takeLast()
Removes and returns the last GuiCamera in the list.
virtual bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts)
Handle an XML start element.
QString path() const
Get the path to these gui camera objects in the list (relative to project root).
GuiCameraList & operator+=(const QList< GuiCameraQsp > &other)
Appends another GuiCameraList to the list and returns a reference to this.
~GuiCameraList()
Create a gui camera list from a list of gui camera file names.
void setName(QString newName)
Gets a list of pre-connected actions that have to do with display, such as color, alpha,...
void removeFirst()
Removes the first GuiCamera in the list.
void removeLast()
Removes the last GuiCamera in the list.
void save(QXmlStreamWriter &stream, const Project *project, FileName newProjectRoot) const
Delete all of the contained GuiCamera objects from disk (see GuiCamera::deleteFromDisk())
void removeAt(int i)
Removes the GuiCamera at a specific index.
void countChanged(int newCount)
Emitted when the number of GuiCameras in the list changes.
QString name() const
Get the human-readable name of this gui cameray list.
void push_back(GuiCameraQsp const &value)
Inserts a GuiCamera at the end of the list.
XmlHandler used to save to xml files.
void append(GuiCameraQsp const &value)
Appends a single GuiCamera to the list.
List of GuiCameras saved as QSharedPointers.
void insert(int i, GuiCameraQsp const &value)
Inserts a GuiCamera into the list at a given index.
QString m_path
This stores the directory name that contains the GuiCamera objects in this list.
This is free and unencumbered software released into the public domain.