Loading [MathJax]/jax/output/NativeMML/config.js
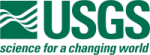 |
Isis 3 Programmer Reference
|
9 #include "SpecialPixel.h"
42 NearestNeighborType = 1,
44 CubicConvolutionType = 4
62 double BiLinear(
const double isamp,
const double iline,
78 double Interpolate(
const double isamp,
const double iline,
~Interpolator()
Destroys the Interpolator object.
double NearestNeighbor(const double isamp, const double iline, const double buf[])
Performs a nearest-neighbor interpolation on the buffer data.
int Lines()
Returns the number of lines needed by the interpolator.
interpType p_type
The type of interpolation to be performed.
double HotSample()
Returns the sample coordinate of the center pixel in the buffer for the interpolator.
interpType
The interpolator type, including: None, Nearest Neighbor, BiLinear or Cubic Convultion.
Interpolator()
Constructs an empty Interpolator object.
void Init()
Initializes the object data members.
double CubicConvolution(const double isamp, const double iline, const double buf[])
Performs a cubic-convulsion interpolation on the buffer data.
double Interpolate(const double isamp, const double iline, const double buf[])
Performs an interpolation on the data according to the parameters set in the constructor.
void SetType(const interpType &type)
Sets the type of interpolation.
int Samples()
Returns the number of samples needed by the interpolator.
double HotLine()
Returns the line coordinate of the center pixel in the buffer for the interpolator.
double BiLinear(const double isamp, const double iline, const double buf[])
Performs a bi-linear interpolation on the buffer data.
This is free and unencumbered software released into the public domain.