Loading [MathJax]/jax/output/NativeMML/config.js
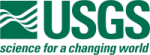 |
Isis 3 Programmer Reference
|
7 #include "jama/jama_svd.h"
8 #include "jama/jama_qr.h"
12 #include "LeastSquares.h"
13 #include "IException.h"
24 int sparseRows,
int sparseCols,
bool jigsaw) {
31 p_sparseRows = sparseRows;
32 p_sparseCols = sparseCols;
38 if (sparseRows == 0 || sparseCols == 0) {
39 QString msg =
"If solving using sparse matrices, you must enter the "
40 "number of rows/columns";
45 p_sparseA.set_size(sparseRows, sparseCols);
46 p_normals.set_size(sparseCols, sparseCols);
47 p_ATb.resize(sparseCols, 1);
58 p_currentFillRow = -1;
99 QString msg =
"Number of elements in data does not match basis [" +
143 int ncolumns = (int)data.size();
145 for(
int c = 0; c < ncolumns; c++) {
159 if((row >=
Rows()) || (row < 0)) {
160 QString msg =
"Index out of bounds [Given = " +
toString(row) +
"]";
174 if((row >=
Rows()) || (row < 0)) {
175 QString msg =
"Index out of bounds [Given = " +
toString(row) +
"]";
207 if((method ==
SPARSE && p_sparseRows == 0) ||
210 QString msg =
"No solution available because no input data was provided";
217 else if(method ==
QRD) {
220 else if(method ==
SPARSE) {
240 for(
int r = 0; r < A.dim1(); r++) {
242 for(
int c = 0; c < A.dim2(); c++) {
253 JAMA::SVD<double> svd(A);
255 TNT::Array2D<double> V;
259 TNT::Array2D<double> invS;
262 for(
int i = 0; i < invS.dim1(); i++) {
263 if(invS[i][i] != 0.0) invS[i][i] = 1.0 / invS[i][i];
267 TNT::Array2D<double> U;
269 TNT::Array2D<double> transU(U.dim2(), U.dim1());
271 for(
int r = 0; r < U.dim1(); r++) {
272 for(
int c = 0; c < U.dim2(); c++) {
273 transU[c][r] = U[r][c];
278 TNT::Array2D<double> VinvS = TNT::matmult(V, invS);
279 TNT::Array2D<double> Aplus = TNT::matmult(VinvS, transU);
284 for(
int r = 0; r < (int)
p_expected.size(); r++) {
288 TNT::Array2D<double> coefs = TNT::matmult(Aplus, b);
293 QString msg =
"Unable to solve least-squares using SVD method. No "
294 "solution available. Not enough knowns or knowns are "
295 "co-linear ... [Unknowns = "
302 std::vector<double> bcoefs;
303 for (
int i = 0; i < coefs.dim1(); i++) bcoefs.push_back(coefs[i][0]);
308 for(
int i = 0; i < (int)
p_input.size(); i++) {
343 for(
int r = 0; r < A.dim1(); r++) {
345 for(
int c = 0; c < A.dim2(); c++) {
355 JAMA::QR<double> qr(A);
359 for(
int r = 0; r < (int)
p_expected.size(); r++) {
366 int full = qr.isFullRank();
368 QString msg =
"Unable to solve-least squares using QR Decomposition. "
369 "The upper triangular R matrix is not full rank";
373 TNT::Array1D<double> coefs = qr.solve(b);
376 std::vector<double> bcoefs;
377 for(
int i = 0; i < coefs.dim1(); i++) {
378 bcoefs.push_back(coefs[i]);
383 for(
int i = 0; i < (int)
p_input.size(); i++) {
434 arma::mat b(p_sparseRows, 1);
437 for (
int r = 0; r < p_sparseRows; r++ )
445 for(
int i = 0; i < p_sparseCols; i++) {
458 if (status ==
false) {
459 QString msg =
"Could not solve sparse least squares problem.";
469 for(
int i = 0; i < p_sparseCols; i++ )
482 for (
int i = 0; i < p_sparseRows; i++ ) {
491 double constrained_vTPv = 0.0;
493 for (
int i = 0; i < p_sparseCols; i++ ) {
523 void LeastSquares::Reset ()
529 p_currentFillRow = -1;
555 QString msg =
"Unable to evaluate until a solution has been computed";
572 QString msg =
"Unable to return residuals until a solution has been computed";
592 QString msg =
"Unable to return residuals until a solution has been computed";
LeastSquares(Isis::BasisFunction &basis, bool sparse=false, int sparseRows=0, int sparseCols=0, bool jigsaw=false)
Creates a LeastSquares Object.
std::vector< double > p_epsilonsSparse
sparse vector of total parameter corrections
int Solve(Isis::LeastSquares::SolveMethod method=SVD)
After all the data has been registered through AddKnown, invoke this method to solve the system of eq...
arma::mat p_xSparse
sparse solution matrix
void SolveSVD()
After all the data has been registered through AddKnown, invoke this method to solve the system of eq...
int p_constrainedParameters
constrained parameters
std::vector< double > GetInput(int row) const
This method returns the data at the given row.
void SetCoefficients(const std::vector< double > &coefs)
Set the coefficients for the equation.
Generic linear equation class.
@ Unknown
A type of error that cannot be classified as any of the other error types.
void FillSparseA(const std::vector< double > &data)
Invoke this method for each set of knowns for sparse solutions.
std::vector< double > p_parameterWeights
vector of parameter weights
std::vector< double > p_expected
A vector of the expected values when solved.
double Evaluate(const std::vector< double > &input)
Invokes the BasisFunction Evaluate method.
double Term(int c) const
Returns the cth term.
QString Name() const
Returns the name of the equation.
int Rows() const
This methods returns the number of rows in the matrix.
double Residual(int i) const
Returns the ith residual.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
std::vector< std::vector< double > > p_input
A vector of the input variables to evaluate.
~LeastSquares()
Destroys the LeastSquares object.
virtual void Expand(const std::vector< double > &vars)
This is the function you should replace depending on your needs.
arma::SpMat< double > p_sparseA
design matrix 'A'
arma::SpMat< double > p_normals
normal equations matrix 'N'
Isis::BasisFunction * p_basis
Pointer to the BasisFunction object.
double GetExpected(int row) const
This method returns the expected value at the given row.
std::vector< double > p_residuals
A vector of the residuals (or difference between expected and solved values).
std::vector< double > p_sqrtWeight
A vector of the square roots of the weights for each known value.
arma::mat p_ATb
right-hand side vector
int Variables() const
Returns the number of variables in the equation.
@ Programmer
This error is for when a programmer made an API call that was illegal.
double p_sigma0
sigma nought - reference variance
bool p_solved
Boolean value indicating solution is complete.
int SolveSparse()
Solve using sparse class.
void AddKnown(const std::vector< double > &input, double expected, double weight=1.0)
Invoke this method for each set of knowns.
@ SVD
Singular Value Decomposition.
double Evaluate(const std::vector< double > &vars)
Compute the equation using the input variables.
int p_degreesOfFreedom
degrees of freedom (redundancy)
This is free and unencumbered software released into the public domain.
int Coefficients() const
Returns the number of coefficients for the equation.
void Weight(int index, double weight)
Reset the weight for the ith known.
void SolveQRD()
After all the data has been registered through AddKnown, invoke this method to solve the system of eq...
std::vector< double > Residuals() const
Returns a vector of residuals (errors).