File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
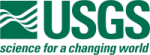 |
Isis 3 Programmer Reference
|
1 #ifndef NumericalApproximation_h
2 #define NumericalApproximation_h
13 #include <gsl/gsl_errno.h>
14 #include <gsl/gsl_spline.h>
15 #include "IException.h"
788 void AddData(
const double x,
const double y);
789 void AddData(
unsigned int n,
double *x,
double *y);
790 void AddData(
const vector <double> &x,
const vector <double> &y);
791 void SetCubicClampedEndptDeriv(
const double yp1,
const double ypn);
792 void AddCubicHermiteDeriv(
unsigned int n,
double *fprimeOfx);
793 void AddCubicHermiteDeriv(
const vector <double> &fprimeOfx);
794 void AddCubicHermiteDeriv(
const double fprimeOfx);
797 double DomainMinimum();
798 double DomainMaximum();
799 bool Contains(
double x);
820 double Evaluate(
const double a,
const ExtrapType &etype = ThrowError);
821 vector <double> Evaluate(
const vector <double> &a,
const ExtrapType &etype = ThrowError);
822 vector <double> PolynomialNevilleErrorEstimate();
823 vector <double> CubicClampedSecondDerivatives();
824 double EvaluateCubicHermiteFirstDeriv(
const double a);
825 double EvaluateCubicHermiteSecDeriv(
const double a);
828 double GslFirstDerivative(
const double a);
829 double BackwardFirstDifference(
const double a,
const unsigned int n = 3,
830 const double h = 0.1);
831 double ForwardFirstDifference(
const double a,
const unsigned int n = 3,
832 const double h = 0.1);
833 double CenterFirstDifference(
const double a,
const unsigned int n = 5,
834 const double h = 0.1);
836 double GslSecondDerivative(
const double a);
837 double BackwardSecondDifference(
const double a,
const unsigned int n = 3,
838 const double h = 0.1);
839 double ForwardSecondDifference(
const double a,
const unsigned int n = 3,
840 const double h = 0.1);
841 double CenterSecondDifference(
const double a,
const unsigned int n = 5,
842 const double h = 0.1);
845 double GslIntegral(
const double a,
const double b);
846 double TrapezoidalRule(
const double a,
const double b);
847 double Simpsons3PointRule(
const double a,
const double b);
848 double Simpsons4PointRule(
const double a,
const double b);
849 double BoolesRule(
const double a,
const double b);
850 double RefineExtendedTrap(
double a,
double b,
double s,
unsigned int n);
851 double RombergsMethod(
double a,
double b);
888 void GslAllocation(
unsigned int npoints);
889 void GslDeallocation();
892 void GslIntegrityCheck(
int gsl_status,
const char *src,
894 void ValidateDataSet();
895 bool InsideDomain(
const double a);
897 bool GslComputed()
const;
899 void ComputeCubicClamped();
900 double ValueToExtrapolate(
const double a,
const ExtrapType &etype);
901 double EvaluateCubicNeighborhood(
const double a);
902 vector <double> EvaluateCubicNeighborhood(
const vector <double> &a,
const ExtrapType &etype);
903 double EvaluateCubicClamped(
const double a);
904 double EvaluateCubicHermite(
const double a);
905 double EvaluatePolynomialNeville(
const double a);
906 vector <double> EvaluateForIntegration(
const double a,
const double b,
907 const unsigned int n);
908 int FindIntervalLowerIndex(
const double a);
911 const string &message,
const char *filesrc,
vector< double > p_fprimeOfx
List of first derivatives corresponding to each x value in the data set (i.e. each value in p_x)
@ CubicNatPeriodic
Cubic Spline interpolation with periodic boundary conditions.
@ Linear
Linear interpolation.
ExtrapType
This enum defines the manner in which a value outside of the domain should be handled if passed to th...
const gsl_interp_type * InterpFunctor
GSL Interpolation specs.
bool p_clampedComputed
Flag variable to determine whether ComputeCubicClamped() has been called.
vector< double > p_y
List of Y values.
double p_clampedDerivFirstPt
First derivative of first x-value, p_x[0]. This is only used for the CubicClamped interpolation type.
@ Polynomial
Polynomial interpolation.
double p_clampedDerivLastPt
First derivative of last x-value, p_x[n-1]. This is only used for the CubicClamped interpolation type...
unsigned int Size()
Returns the number of the coordinates added to the data set.
@ Akima
Non-rounded Akima Spline interpolation with natural boundary conditions.
@ CubicClamped
Cubic Spline interpolation with clamped boundary conditions.
gsl_interp_accel * p_acc
Lookup accelorator.
bool p_clampedEndptsSet
Flag variable to determine whether SetCubicClampedEndptDeriv() has been called after all data was add...
InterpType InterpolationType()
Returns the enumerated type of interpolation chosen.
bool p_dataValidated
Flag variable to determine whether ValidateDataSet() has been called.
@ ThrowError
Evaluate() throws an error if a is outside of the domain.
@ CubicNeighborhood
Cubic Spline interpolation using 4-pt Neighborhoods with natural boundary conditions.
vector< double > p_polyNevError
Estimate of error for interpolation evaluated at x. This is only used for the PolynomialNeville inter...
@ PolynomialNeville
Polynomial interpolation using Neville's algorithm.
vector< double > p_clampedSecondDerivs
List of second derivatives evaluated at p_x values. This is only used for the CubicClamped interpolat...
Namespace for the standard library.
map< InterpType, InterpFunctor > FunctorList
Set up a std::map of GSL interpolator functors. List of function types.
static FunctorList p_interpFunctors
Maintains list of interpolator options.
vector< double > p_x
List of X values.
@ CubicHermite
Cubic Spline interpolation using the Hermite cubic polynomial.
InterpType p_itype
Interpolation type.
InterpType
This enum defines the types of interpolation supported in this class.
FunctorList::const_iterator FunctorConstIter
GSL Iterator.
ErrorType
Contains a set of exception error types.
NumericalApproximation provides various numerical analysis methods of interpolation,...
This is free and unencumbered software released into the public domain.
@ CubicNatural
Cubic Spline interpolation with natural boundary conditions.
gsl_spline * p_interp
Currently active interpolator.