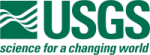 |
Isis 3 Programmer Reference
|
9 #include "SpecialPixel.h"
63 static unsigned char To8Bit(
const double d);
65 static short int To16Bit(
const double d);
67 static short unsigned int To16UBit(
const double d);
69 static float To32Bit(
const double d);
72 static double ToDouble(
const unsigned char t);
73 static double ToDouble(
const short int t);
74 static double ToDouble(
const short unsigned int t);
75 static double ToDouble(
const float t);
78 static float ToFloat(
const unsigned char d);
79 static float ToFloat(
const short int d);
80 static float ToFloat(
const short unsigned int d);
81 static float ToFloat(
const double d);
84 static std::string
ToString(
double d);
96 return(d < VALID_MIN8);
119 return(f < VALID_MIN4);
131 return(d >= VALID_MIN8);
151 static inline bool IsNull(
const double d) {
171 static inline bool IsHigh(
const double d) {
172 return(d == HIGH_REPR_SAT8) || (d == HIGH_INSTR_SAT8);
191 static inline bool IsLow(
const double d) {
192 return(d == LOW_REPR_SAT8) || (d == LOW_INSTR_SAT8);
211 static inline bool IsHrs(
const double d) {
212 return(d == HIGH_REPR_SAT8);
231 static inline bool IsHis(
const double d) {
232 return(d == HIGH_INSTR_SAT8);
251 static inline bool IsLis(
const double d) {
252 return(d == LOW_INSTR_SAT8);
271 static inline bool IsLrs(
const double d) {
272 return(d == LOW_REPR_SAT8);
int m_band
band coordinate of pixel
bool IsLrs()
Returns true if the input pixel is low representation saturation.
static short unsigned int To16UBit(const double d)
Converts double pixels to short unsigned int pixels with special pixel translations.
double ToDouble()
Converts stored pixel value to a double.
Pixel()
Constructs an empty Pixel.
Pixel & operator=(const Pixel &other)
Copy assignment operator.
bool IsLis()
Returns true if the input pixel is low instrument saturation.
int m_sample
sample coordinate of pixel
static bool IsHis(const double d)
Returns true if the input pixel is high instrument saturation.
virtual ~Pixel()
Default destructor.
static bool IsHrs(const double d)
Returns true if the input pixel is high representation saturation.
static bool IsLis(const double d)
Returns true if the input pixel is low instrument saturation.
static bool IsSpecial(const double d)
Returns true if the input pixel is special.
float ToFloat()
Converts internal pixel value to float with pixel translations and care for overflows (underflows are...
static bool IsSpecial(const float f)
Returns true if the input pixel is special.
short unsigned int To16Ubit()
Converts internal pixel value to a short int pixel with special pixel translations.
bool IsSpecial()
Returns true if the input pixel is special.
static bool IsHigh(const double d)
Returns true if the input pixel is one of the high saturation types.
static bool IsLow(const double d)
Returns true if the input pixel is one of the low saturation types.
bool IsLow()
Returns true if the input pixel is one of the low saturation types.
std::string ToString()
Returns the name of the pixel type as a string.
unsigned char To8Bit()
Converts internal pixel value to an unsigned char pixel with special pixel translations.
float To32Bit()
Converts internal pixel value to float with special pixel translations.
int m_line
line coordinate of pixel
Store and/or manipulate pixel values.
static bool IsNull(const double d)
Returns true if the input pixel is null.
static bool IsLrs(const double d)
Returns true if the input pixel is low representation saturation.
short int To16Bit()
Converts internal pixel value to a short int pixel with special pixel translations.
bool IsValid()
Returns true if the input pixel is valid.
bool IsHis()
Returns true if the input pixel is high instrument saturation.
bool IsNull()
Returns true if the input pixel is null.
static bool IsValid(const double d)
Returns true if the input pixel is valid.
This is free and unencumbered software released into the public domain.
bool IsHigh()
Returns true if the input pixel is one of the high saturation types.
bool IsHrs()
Returns true if the input pixel is high representation saturation.