File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
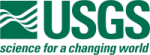 |
Isis 3 Programmer Reference
|
7 #include "PolygonSeeder.h"
9 #include "IException.h"
10 #include "LeastSquares.h"
12 #include "PolygonTools.h"
13 #include "PolynomialBivariate.h"
14 #include "LeastSquares.h"
16 #include "ProjectionFactory.h"
91 errorSpot =
"Algorithm";
106 errorSpot =
"MinimumThickness";
116 errorSpot =
"MinimumArea";
126 QString msg =
"Improper format for PolygonSeeder PVL [";
127 msg += pvl.
fileName() +
"]. Location [" + errorSpot +
"]";
147 const geos::geom::Envelope *xyBoundBox) {
149 QString msg =
"Polygon did not meet the minimum area of [";
156 pow(std::max(xyBoundBox->getWidth(), xyBoundBox->getHeight()), 2.0);
158 QString msg =
"Polygon did not meet the minimum thickness ratio of [";
double p_minimumArea
The value for the 'MinimumArea' Keyword in the PolygonSeederAlgorithm group of the Pvl that is passed...
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
QString p_algorithmName
The value for the 'Name' Keyword in the PolygonSeederAlgorithm group of the Pvl that is passed into t...
A single keyword-value pair.
void addKeyword(const PvlKeyword &keyword, const InsertMode mode=Append)
Add a keyword to the container.
virtual PvlGroup PluginParameters(QString grpName)
Plugin parameters.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
double MinimumArea()
Return the minimum allowed area of the polygon.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
@ Traverse
Search child objects.
double MinimumThickness()
Return the minimum allowed thickness of the polygon.
Contains multiple PvlContainers.
Pvl * invalidInput
The Pvl passed in by the constructor minus what was used.
const PolygonSeeder & operator=(const PolygonSeeder &other)
Assignment operator.
QString fileName() const
Returns the filename used to initialise the Pvl object.
virtual ~PolygonSeeder()
Destroys the PolygonSeeder object.
virtual void Parse(Pvl &pvl)
Initialize parameters in the PolygonSeeder class using a PVL specification.
void deleteKeyword(const QString &name)
Remove a specified keyword.
QString Algorithm() const
The name of the algorithm, read from the Name Keyword in the PolygonSeeder Pvl passed into the constr...
QString StandardTests(const geos::geom::MultiPolygon *multiPoly, const geos::geom::Envelope *polyBoundBox)
Check the polygon to see if it meets standard criteria.
PolygonSeeder(Pvl &pvl)
Create PolygonSeeder object.
double p_minimumThickness
The value for the 'MinimumThickness' Keyword in the PolygonSeederAlgorithm group of the Pvl that is p...
Pvl InvalidInput()
This method returns a copy of the Pvl passed in by the constructor (from a def file probably) minus w...
This class is used as the base class for all PolygonSeeder objects.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....