Loading [MathJax]/jax/output/NativeMML/config.js
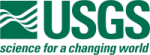 |
Isis 3 Programmer Reference
|
10 #include "PolynomialUnivariate.h"
12 #include "IException.h"
69 double derivative = 0;
72 derivative += i *
Coefficient(i) * pow(value, i - 1);
91 const int coefIndex) {
95 derivative = pow(value, coefIndex);
97 else if(coefIndex == 0) {
101 QString msg =
"Unable to evaluate the derivative of the univariate polynomial for the given "
102 "coefficient index [" +
toString(coefIndex) +
"]. "
103 "Index is negative or exceeds degree of polynomial ["
void SetCoefficients(const std::vector< double > &coefs)
Set the coefficients for the equation.
int p_degree
The order/degree of the polynomial.
PolynomialUnivariate(int degree)
Create a PolynomialUnivariate object.
double DerivativeVar(const double value)
This will take the Derivative with respect to the variable and evaluate at given value.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
double DerivativeCoef(const double value, const int coefIndex)
Evaluate the derivative of the polynomial defined by the given coefficients with respect to the coeff...
Time based linear equation class.
void Expand(const std::vector< double > &vars)
This is the the overriding virtual function that provides the expansion of the two input variables in...
@ Programmer
This error is for when a programmer made an API call that was illegal.
std::vector< double > p_terms
A vector of the terms in the equation.
This is free and unencumbered software released into the public domain.
int Coefficients() const
Returns the number of coefficients for the equation.
double Coefficient(int i) const
Returns the ith coefficient.