File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
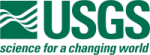 |
Isis 3 Programmer Reference
|
14 #include "PvlKeyword.h"
41 t = t.remove(QRegExp(
"[\\w_-\"'")).toUpper();
43 if(t ==
"STRING")
return StringKeyword;
44 else if(t ==
"BOOL")
return BoolKeyword;
45 else if(t ==
"INTEGER")
return IntegerKeyword;
46 else if(t ==
"REAL")
return RealKeyword;
47 else if(t ==
"OCTAL")
return OctalKeyword;
48 else if(t ==
"HEX")
return HexKeyword;
49 else if(t ==
"BINARY")
return BinaryKeyword;
50 else if(t ==
"ENUM")
return EnumKeyword;
117 void add(
const QString &file);
118 void add(
Pvl &keymap);
142 virtual QString formatValue(
const PvlKeyword &keyword,
144 virtual QString formatName(
const PvlKeyword &keyword);
145 virtual QString formatEnd(
const QString name,
147 virtual QString formatEOL() {
151 virtual KeywordType type(
const PvlKeyword &keyword);
152 virtual int accuracy(
const PvlKeyword &keyword);
156 virtual QString addQuotes(
const QString value);
159 QString m_keywordMapFile;
A single keyword-value pair.
Container for cube-like labels.
KeywordType
The different types of keywords that can be formatted.
KeywordType toKeywordType(const QString type)
Convert a string representing a type of keyword to the corresponding enumeration.
This is free and unencumbered software released into the public domain.