File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
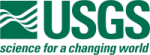 |
Isis 3 Programmer Reference
|
7 #include "LabelTranslationManager.h"
9 #include "IException.h"
13 #include "PvlContainer.h"
15 #include "PvlKeyword.h"
16 #include "PvlObject.h"
17 #include "PvlToPvlTranslationManager.h"
31 PvlToPvlTranslationManager::PvlToPvlTranslationManager(
const QString &transFile)
58 const QString &transFile)
73 std::istream &transStrm)
84 void PvlToPvlTranslationManager::SetLabel(
Pvl &inputLabel) {
112 while((grp =
InputGroup(translationGroupName, inst++)).name() !=
"") {
150 while((grp =
InputGroup(translationGroupName, inst++)).name() !=
"") {
158 while(it != transGroup.
end()) {
163 for(
int v = 0; v < (*con)[(result[0])].size(); v++) {
165 (*con)[result[0]][v]),
166 (*con)[result[0]].unit(v));
171 it = transGroup.
findKeyword(
"InputKey", it + 1, transGroup.
end());
233 int instanceNumber = 0;
235 bool anInputGroupFound =
false;
237 while(inputGroupKeyword.
name() !=
"") {
239 if(containingGroup != NULL) {
240 anInputGroupFound =
true;
248 inputGroupKeyword =
InputGroup(translationGroupName, instanceNumber);
251 if(anInputGroupFound) {
252 QString msg =
"Unable to find input keyword [" +
InputKeywordName(translationGroupName) +
257 QString container =
"";
259 for(
int i = 0; i <
InputGroup(translationGroupName).
size(); i++) {
260 if(i > 0) container +=
",";
262 container +=
InputGroup(translationGroupName)[i];
265 QString msg =
"Unable to find input group [" + container +
292 while((grp =
InputGroup(translationGroupName, inst++)).name() !=
"") {
307 if(inputGroup.
size() == 1 &&
317 objectIndex < inputGroup.
size() - 1;
319 if(currentObject->
hasObject(inputGroup[objectIndex])) {
320 currentObject = ¤tObject->
findObject(inputGroup[objectIndex]);
328 if(currentObject->
hasObject(inputGroup[objectIndex])) {
329 return ¤tObject->
findObject(inputGroup[objectIndex]);
331 else if(currentObject->
hasGroup(inputGroup[objectIndex])) {
332 return ¤tObject->
findGroup(inputGroup[objectIndex]);
QString name() const
Returns the keyword name.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
PvlGroup & group(const int index)
Return the group at the specified index.
Contains Pvl Groups and Pvl Objects.
A single keyword-value pair.
QList< PvlKeyword >::const_iterator ConstPvlKeywordIterator
The const keyword iterator.
void addValue(QString value, QString unit="")
Adds a value with units.
int groups() const
Returns the number of groups contained.
QString OutputName(const QString translationGroupName)
Retrieves a string containing the value of the OutputName keyword for the translation group with the ...
virtual QString Translate(QString translationGroupName, int findex=0)
Returns a translated value.
bool hasGroup(const QString &name) const
Returns a boolean value based on whether the object has the specified group or not.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
virtual PvlKeyword DoTranslation(const QString translationGroupName)
Translate the requested output name to output values using the input name and values or default value...
Container for cube-like labels.
virtual PvlContainer * CreateContainer(const QString translationGroupName, Pvl &pvl)
Create the requsted container and any containers above it and return a reference to the container.
void setName(QString name)
Sets the keyword name.
Contains multiple PvlContainers.
PvlToPvlTranslationManager(const QString &transFile)
Constructs and initializes a TranslationManager object from given the Pvl translation file.
virtual const PvlKeyword & InputKeyword(const QString translationGroupName) const
Returns the ith input value associated with the output name argument.
virtual PvlContainer * CreateContainer(const QString translationGroupName, Pvl &pvl)
Creates all parent PVL containers for an output keyword.
virtual ~PvlToPvlTranslationManager()
Destroys the TranslationManager object.
Allows applications to translate simple text files.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
QString fileName() const
Returns the filename used to initialise the Pvl object.
QString name() const
Returns the container name.
QString Translate(const QString translationGroupName, const QString inputKeyValue="") const
Translates a single output value from the given translation group name and input value.
Pvl p_fLabel
A Pvl object for the input label file.
bool hasObject(const QString &name) const
Returns a boolean value based on whether the object exists in the current PvlObject or not.
virtual QString InputKeywordName(const QString translationGroupName) const
Returns the input keyword name from the translation table corresponding to the output name argument.
virtual PvlKeyword InputGroup(const QString translationGroupName, const int inst=0) const
Returns the input group name from the translation table corresponding to the output name argument.
bool IsAuto(const QString translationGroupName)
Determines whether the given group should be automatically translated.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
int size() const
Returns the number of values stored in this keyword.
bool IsOptional(const QString translationGroupName)
Determines whether the translation group is optional.
virtual const PvlContainer * GetContainer(const PvlKeyword &inputGroup) const
Return a container from the input label according tund.
virtual bool InputHasKeyword(const QString translationGroupName)
Indicates if the input keyword corresponding to the output name exists in the label.
PvlKeyword & findKeyword(const QString &name)
Find a keyword with a specified name.
PvlKeywordIterator end()
Return the ending iterator.
Contains more than one keyword-value pair.
static bool stringEqual(const QString &string1, const QString &string2)
Checks to see if two QStrings are equal.
void Auto(Pvl &outputLabel)
Automatically translate all the output names found in the translation table If a output name does not...
PvlKeywordIterator begin()
Return the beginning iterator.
This is free and unencumbered software released into the public domain.
Pvl & TranslationTable()
Protected accessor for pvl translation table passed into class.