File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
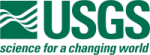 |
Isis 3 Programmer Reference
|
7 #include "LabelTranslationManager.h"
10 #include <QDomDocument>
11 #include <QDomElement>
14 #include "IException.h"
18 #include "PvlContainer.h"
20 #include "PvlKeyword.h"
21 #include "PvlObject.h"
22 #include "PvlToXmlTranslationManager.h"
36 PvlToXmlTranslationManager::PvlToXmlTranslationManager(
const QString &transFile)
50 const QString &transFile)
82 validKeywords.push_back(pair<QString, int>(
"OutputAttributes", -1));
83 validKeywords.push_back(pair<QString, int>(
"OutputSiblings", -1));
110 while((grp =
InputGroup(transGroupName, inst++)).name() !=
"") {
134 QDomElement &parentElement) {
137 QString transGroupName = transGroup.
name();
140 while (grp.
name() !=
"") {
152 QString untranslatedValue = inputKeyword[0];
155 QString units = inputKeyword.
unit();
156 if (outputName.size() == 2 && outputName[0] ==
"att") {
157 parentElement.setAttribute(outputName[1], translatedValue);
158 if (transGroup.
hasKeyword(
"OutputAttributes")) {
163 QDomElement newElement = parentElement.ownerDocument().createElement(outputName[0]);
165 parentElement.appendChild(newElement);
166 if (transGroup.
hasKeyword(
"OutputAttributes")) {
171 if (transGroup.
hasKeyword(
"OutputSiblings")) {
183 QDomElement newElement = parentElement.ownerDocument().createElement(
OutputName(transGroupName));
185 parentElement.appendChild(newElement);
186 if (transGroup.
hasKeyword(
"OutputAttributes")) {
189 if (transGroup.
hasKeyword(
"OutputSiblings")) {
208 QDomDocument &outputLabel) {
228 QDomElement element = outputLabel.documentElement();
256 int instanceNumber = 0;
258 bool anInputGroupFound =
false;
260 while(inputGroupKeyword.
name() !=
"") {
262 if(containingGroup != NULL) {
263 anInputGroupFound =
true;
271 inputGroupKeyword =
InputGroup(transGroupName, instanceNumber);
274 if(anInputGroupFound) {
275 QString msg =
"Unable to find input keyword [" +
InputKeywordName(transGroupName) +
276 "] for output name [" + transGroupName +
"] in file [" +
281 QString container =
"";
284 if(i > 0) container +=
",";
289 QString msg =
"Unable to find input group [" + container +
"] for output name [" +
312 while((grp =
InputGroup(transGroupName, inst++)).name() !=
"") {
334 if(inputGroup.
size() == 1 &&
344 objectIndex < inputGroup.
size() - 1;
346 if(currentObject->
hasObject(inputGroup[objectIndex])) {
347 currentObject = ¤tObject->
findObject(inputGroup[objectIndex]);
355 if(currentObject->
hasObject(inputGroup[objectIndex])) {
356 return ¤tObject->
findObject(inputGroup[objectIndex]);
358 else if(currentObject->
hasGroup(inputGroup[objectIndex])) {
359 return ¤tObject->
findGroup(inputGroup[objectIndex]);
379 QDomElement &xmlRootElement) {
384 QDomElement *currentElement = &xmlRootElement;
389 if (containers.
size() > 0 && currentElement->tagName() == containers[0]) {
394 while (i < containers.
size()) {
400 bool addNewElement =
false;
402 if (specifications.size() == 2 && specifications[0] ==
"new") {
403 addNewElement =
true;
409 QDomElement childElement = xmlRootElement.ownerDocument().createElement(specifications[1]);
410 *currentElement = currentElement->appendChild(childElement).toElement();
414 else if (currentElement->namedItem(containers[i]).isNull()) {
415 QDomElement childElement = xmlRootElement.ownerDocument().createElement(specifications[0]);
416 *currentElement = currentElement->appendChild(childElement).toElement();
421 *currentElement = currentElement->firstChildElement(containers[i]);
425 return currentElement;
439 QDomElement &parent) {
441 for (
int i = 0; i < outputSiblings.
size(); i++) {
443 parsedSibling.reserve(5);
445 if (parsedSibling.size() != 2) {
447 QString msg =
"Malformed OutputSibling [" + outputSiblings[i] +
"]. OutputSiblings must" +
448 " be in the form of tag|value";
452 if (parent.namedItem(parsedSibling[0]).isNull()) {
454 QDomElement childElement = parent.ownerDocument().createElement(parsedSibling[0]);
456 parent.appendChild(childElement).toElement();;
472 QDomElement &element) {
475 for (
int i = 0; i < outputAttributes.
size(); i++) {
478 if (parsedAttribute.size() != 2) {
479 QString msg =
"Malformed output attribute [" + outputAttributes[i] +
480 "]. OutputAttributes must be in the form of att@attribute_name|value";
483 element.setAttribute(parsedAttribute[0], parsedAttribute[1]);
502 QDomElement newElement = parent.ownerDocument().createElement(name);
505 parent.appendChild(newElement).toElement();;
519 QDomText valueText = element.ownerDocument().createTextNode(value);
521 element.appendChild(valueText);
524 element.setAttribute(
"unit", units);
539 element.firstChild().setNodeValue(value);
541 element.setAttribute(
"unit", units);
virtual QStringList parseSpecification(QString specification) const
Parses and validates a dependency specification.
void addSiblings(PvlKeyword outputSiblings, QDomElement &parent)
Take in outputSiblings PvlKeyword and turn each sibling into its corresponding QDomElement.
QString name() const
Returns the keyword name.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
PvlGroup & group(const int index)
Return the group at the specified index.
virtual std::vector< std::pair< QString, int > > validKeywords() const
Returns a vector of valid keyword names and their sizes.
Contains Pvl Groups and Pvl Objects.
A single keyword-value pair.
void addAttributes(PvlKeyword something, QDomElement &parent)
Take in the outputAttributes PvlKeyword and add each attribute to the appropriate element given as an...
virtual QString Translate(QString translationGroupName, int inputIndex=0)
Returns a translated value.
void doTranslation(PvlGroup transGroup, QDomElement &parent)
Translate the requested output name to output values using the input name and values or default value...
int groups() const
Returns the number of groups contained.
QString OutputName(const QString translationGroupName)
Retrieves a string containing the value of the OutputName keyword for the translation group with the ...
bool hasGroup(const QString &name) const
Returns a boolean value based on whether the object has the specified group or not.
static void addElement(QDomElement &parent, QString name, QString value, QString units="")
Add a QDomElement to the given parent with the indicated value and units.
virtual std::vector< std::pair< QString, int > > validKeywords() const
Returns a vector of valid keyword names and their sizes.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
virtual ~PvlToXmlTranslationManager()
Destroys the TranslationManager object.
Container for cube-like labels.
QDomElement * createParentElements(const QString translationGroupName, QDomElement &xml)
Read the OutputPosition for the translation group name passed and create any parent elements specifie...
static void setElementValue(QDomElement &element, QString value, QString units="")
Set the QDomElement's value, and units, if units != "".
Contains multiple PvlContainers.
virtual bool InputHasKeyword(const QString translationGroupName)
Indicates if the input keyword corresponding to the output name exists in the label.
void SetLabel(Pvl &inputLabel)
Internalizes a Pvl formatted label for translation.
static void resetElementValue(QDomElement &element, QString value, QString units="")
Reset the QDomElement's value, and units, if units != "".
PvlKeyword OutputPosition(const QString translationGroupName)
Retrieves the OutputPosition PvlKeyword for the translation group with the given name.
Allows applications to translate simple text files.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
PvlToXmlTranslationManager(const QString &transFile)
Constructs and initializes a TranslationManager object from given the Pvl translation file.
QString fileName() const
Returns the filename used to initialise the Pvl object.
QString name() const
Returns the container name.
QString Translate(const QString translationGroupName, const QString inputKeyValue="") const
Translates a single output value from the given translation group name and input value.
bool hasObject(const QString &name) const
Returns a boolean value based on whether the object exists in the current PvlObject or not.
virtual QString InputKeywordName(const QString translationGroupName) const
Returns the input keyword name from the translation table corresponding to the output name argument.
virtual const PvlContainer * GetContainer(const PvlKeyword &inputGroup) const
Return a container from the input label with the path given by the "InputPosition" keyword of the tra...
virtual PvlKeyword InputGroup(const QString translationGroupName, const int inst=0) const
Returns the input group name from the translation table corresponding to the output name argument.
bool IsAuto(const QString translationGroupName)
Determines whether the given group should be automatically translated.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
int size() const
Returns the number of values stored in this keyword.
bool IsOptional(const QString translationGroupName)
Determines whether the translation group is optional.
QString unit(const int index=0) const
Returns the units of measurement of the element of the array of values for the object at the specifie...
PvlKeyword & findKeyword(const QString &name)
Find a keyword with a specified name.
void Auto(QDomDocument &outputLabel)
Automatically translate all the output names found in the translation table.
Contains more than one keyword-value pair.
static bool stringEqual(const QString &string1, const QString &string2)
Checks to see if two QStrings are equal.
virtual const PvlKeyword & InputKeyword(const QString translationGroupName) const
Uses the translation file group name to find the input label's PvlKeyword that corresponds to the Inp...
Pvl m_inputLabel
A Pvl object for the input label file.
This is free and unencumbered software released into the public domain.
Pvl & TranslationTable()
Protected accessor for pvl translation table passed into class.