File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
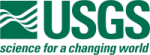 |
Isis 3 Programmer Reference
|
7 #include "QtImporter.h"
12 #include "IException.h"
30 "The file [" + inputName.
expanded() +
31 "] does not contain a recognized image format",
139 return qGreen(pixel);
163 return qAlpha(pixel);
virtual ~QtImporter()
Destruct the importer.
void setSamples(int s)
Set the sample dimension (width) of the output image.
virtual bool isArgb() const
Tests to see if the input image is has an alpha channel, implying RGBA.
File name manipulation and expansion.
virtual int getAlpha(int pixel) const
Retrieves the alpha component of the given pixel.
Imports images with standard formats into Isis as cubes.
virtual bool isGrayscale() const
Tests to see if the input image is grayscale (no RGB/A).
virtual int getBlue(int pixel) const
Retrieves the blue component of the given pixel.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
QImage * m_qimage
Structure holding all input image data in memory.
QtImporter(FileName inputName)
Construct the importer.
void setDefaultBands()
Set the number of bands to be created for the output cube based on the number of color channels in th...
virtual int getGray(int pixel) const
Retrieves the gray component of the given pixel.
void setLines(int l)
Set the line dimension (height) of the output image.
virtual bool isRgb() const
Tests to see if the input image is neither grayscale nor has an alpha channel, implying RGB (no alpha...
virtual int getPixel(int s, int l) const
Returns a representation of a pixel for the input format that can then be broken down into specific g...
virtual int getGreen(int pixel) const
Retrieves the green component of the given pixel.
virtual void updateRawBuffer(int line, int band) const
Does nothing as Qt reads the entire input image into memory, and therefore does not need to be update...
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
virtual int getRed(int pixel) const
Retrieves the red component of the given pixel.