File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
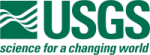 |
Isis 3 Programmer Reference
|
1 #ifndef ShapeDisplayProperties_H
2 #define ShapeDisplayProperties_H
16 #include "DisplayProperties.h"
17 #include "XmlStackedHandler.h"
20 class QXmlStreamWriter;
27 class XmlStackedHandlerReader;
119 virtual bool startElement(
const QString &namespaceURI,
const QString &localName,
120 const QString &qName,
const QXmlAttributes &atts);
124 virtual bool endElement(
const QString &namespaceURI,
const QString &localName,
125 const QString &qName);
void toggleShowLabel()
Change the visibility of the display name.
This class is designed to serialize QColor in a human-readable form.
@ None
Null display property for bit-flag purposes.
This is free and unencumbered software released into the public domain.
File name manipulation and expansion.
void setValue(Property prop, QVariant value)
This is the generic mutator for properties.
void addSupport(Property prop)
Call this with every property you support, otherwise they will not communicate properly between widge...
void setColor(QColor newColor)
Change the color associated with this shape.
void save(QXmlStreamWriter &stream, const Project *project, FileName newProjectRoot) const
Saves this object to an XML file.
Property
This is a list of properties and actions that are possible.
@ Selected
The selection state of this control net (bool)
ShapeDisplayProperties(QString displayName, QObject *parent=NULL)
ShapeDisplayProperties constructor.
Manage a stack of content handlers for reading XML files.
This is the GUI communication mechanism for shape objects.
The main project for ipce.
QMap< int, QVariant > * m_propertyValues
This is a map from Property to value – the reason I use an int is so Qt knows how to serialize this Q...
static QList< ShapeDisplayProperties * > senderToData(QObject *sender)
Get the display properties from a slot.
This class is used for processing an XML file containing information about a WorkOrder.
virtual bool characters(const QString &ch)
This is called when the XML processor has parsed a chunk of character data.
virtual ~ShapeDisplayProperties()
The destructor.
QString displayName() const
Returns the display name.
bool supports(Property prop)
Support for this may come later.
virtual bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts)
This overrides the parent startElement function in XmlStackedHandler so the parser can handle an XML ...
QVariant getValue(Property prop) const
Get a property's associated data.
Property m_propertiesUsed
This indicated whether any widgets with this DisplayProperties is using a particular property.
XmlHandler(ShapeDisplayProperties *displayProperties)
Constructor for the XmlHandler class.
XML Handler that parses XMLs in a stack-oriented way.
virtual bool endElement(const QString &namespaceURI, const QString &localName, const QString &qName)
The XML reader invokes this method at the end of every element in the XML document.
void setSelected(bool)
Change the selected state associated with this shape.
static QColor randomColor()
Creates and returns a random color for the initial color of the footprint polygon.
This is free and unencumbered software released into the public domain.
Q_DECLARE_METATYPE(Isis::PlotWindow *)
We have plot windows as QVariant data types, so here it's enabled.
void setShowLabel(bool)
Change the visibility of the display name associated with this shape.
@ ShowLabel
True if the control net should show its display name (bool)