File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
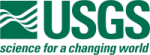 |
Isis 3 Programmer Reference
|
8 #include "SpectralDefinition2D.h"
13 #include "ProcessBySample.h"
36 QString msg = QObject::tr(
"Input calibration file [%1] must have 2 lines: "
37 "one containing wavelength centers and one containing widths").
65 QString msg = QObject::tr(
"Unable to open input cube [%1] and read it into a spectral "
66 "definition.").arg(smileDefFilename.
toString());
86 if ( (b >
m_sectionList->at(i)) && (b < m_sectionList->at(i+1)) ) {
114 const int band)
const {
124 Spectel SpectralDefinition2D::findSpectelByWavelength(
const double wavelength,
125 const int sectionNumber)
const {
136 const int sectionNumber)
const {
138 double bestdiff = DBL_MAX;
147 end = spectrum->length();
153 for (
int i=start; i<end; i++) {
154 diff = spectrum->at(i).centerWavelength() - wavelength;
155 if (std::abs(diff) < std::abs(bestdiff)) {
162 return spectrum->at(bestband);
175 for(
int band=0; band<
m_spectelList->at(samp)->size(); band++){
177 temp+=
"Spectel at (s,b) (";
178 temp+= QString::number(samp);
180 temp+= QString::number(band);
181 temp+=
") : Wavelength=";
198 if (in.
Band() == 1) {
213 int index = in.
Band() - 1;
217 (((spectrum->at(index-2).centerWavelength() <
218 spectrum->at(index-1).centerWavelength()) &&
219 (spectrum->at(index-1).centerWavelength() >
220 spectrum->at(index).centerWavelength())) ||
221 ((spectrum->at(index-2).centerWavelength() >
222 spectrum->at(index-1).centerWavelength()) &&
223 (spectrum->at(index-1).centerWavelength() <
224 spectrum->at(index).centerWavelength())))) {
QList< int > * m_sectionList
The list of sections.
int sectionNumber(int s, int l, int b) const
returns section number given (s,l,b)
void ProcessCubeInPlace(const Functor &funct, bool threaded=true)
Stores information about a "Spectral pixel" or spectel.
This is free and unencumbered software released into the public domain.
File name manipulation and expansion.
@ Unknown
A type of error that cannot be classified as any of the other error types.
double centerWavelength() const
Gets central wavelength of spectel.
QString toString()
Returns QString representation of SpectralDefinition2D.
int m_nb
Number of bands in input Cube.
double filterWidth() const
Gets wavelength width associated with spectel.
QList< QList< Spectel > * > * m_spectelList
Internally represent the samples x 2 lines x n bands calibration file Outside list is the sample inde...
void operator()(Buffer &in) const
Internal function used to help read-in a calibration cube.
Buffer for reading and writing cube data.
int m_numSections
The number of sections of this Spectral Definition.
QString expanded() const
Returns a QString of the full file name including the file path, excluding the attributes.
~SpectralDefinition2D()
destructor
SpectralDefinition2D(FileName smileDefFilename)
Construct a SpectralDefinition2D object using a filename.
int m_nl
Number of lines in input Cube.
void SetText(const QString &text)
Changes the value of the text string reported just before 0% processed.
Isis::Progress * Progress()
This method returns a pointer to a Progress object.
IO Handler for Isis Cubes.
virtual int bandCount() const
Returns the number of virtual bands for the cube.
QString toString() const
Returns a QString of the full file name including the file path, excluding the attributes with any Is...
int Band(const int index=0) const
Returns the band position associated with a shape buffer index.
void Finalize()
Cleans up by closing cubes and freeing memory.
void SetProcessingDirection(ProcessingDirection direction)
Set the direction the data will be read, either all lines in a single band proceeding to the next ban...
virtual int sectionCount() const
Returns the number of sections in the calibration image.
int Sample(const int index=0) const
Returns the sample position associated with a shape buffer index.
int m_ns
Number of samples in input Cube.
Spectel findSpectel(const int sample, const int line, const int band) const
Get the Spectel at some sample, line, band (associated with your input/calibration file)
Isis::Cube * SetInputCube(const QString ¶meter, int requirements=0)
Opens an input cube specified by the user and verifies requirements are met.
This is free and unencumbered software released into the public domain.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....