Loading [MathJax]/jax/output/NativeMML/config.js
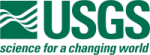 |
Isis 3 Programmer Reference
|
3 #include "SpectralPlotWindow.h"
11 #include "MdiCubeViewport.h"
14 #include "PvlObject.h"
45 foreach (
QAction *menuAction, menuBar()->actions()) {
46 if (menuAction->text() ==
"&Options") {
47 QMenu *optsMenu = qobject_cast<QMenu *>(menuAction->parentWidget());
55 SpectralPlotWindow::~SpectralPlotWindow() {
81 QPen markerPen(color);
82 markerPen.setWidth(1);
84 QwtPlotMarker *newMarker =
new QwtPlotMarker;
85 newMarker->setLineStyle(QwtPlotMarker::LineStyle(2));
86 newMarker->setLinePen(markerPen);
87 newMarker->attach(
plot());
88 newMarker->setVisible(
false);
101 int redBand = 0, greenBand = 0, blueBand = 0, grayBand = 0;
107 if (pvl.
findObject(
"IsisCube").hasGroup(
"BandBin")) {
Cube display widget for certain Isis MDI applications.
A single keyword-value pair.
QwtPlotMarker * m_grayBandLine
The band marker for the gray band.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
Units
These are all the possible units for the x or y data in a plot curve.
void nullify()
This initializes the class member data to NULL.
QwtPlotMarker * m_greenBandLine
The band marker for the green band.
QwtPlotMarker * m_blueBandLine
The band marker for the blue band.
PlotCurve::Units xAxisUnits() const
This is the data-type of the curves' x data in this plot window.
void update(MdiCubeViewport *activeViewport)
This window can show markers for the currently visible bands.
QwtPlotMarker * createMarker(QColor color)
This is a helper method to create new band markers with the same line style and a custom color.
Contains multiple PvlContainers.
MdiCubeViewport * m_cvp
The viewport to be used as a reference for band markers.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
IO Handler for Isis Cubes.
QAction * m_showHideBandMarkers
This action toggles band marker visibility.
@ Wavelength
The data is a wavelength.
void setBandMarkersVisible(bool visible)
QwtPlot * plot()
Get the plot encapsulated by this PlotWindow.
double toDouble(const QString &string)
Global function to convert from a string to a double.
Namespace for the standard library.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
bool m_markersVisible
True if the visibile state of the active markers should be true.
bool bandMarkersVisible() const
PvlKeyword & findKeyword(const QString &name)
Find a keyword with a specified name.
QwtPlotMarker * m_redBandLine
The band marker for the red band.
void setViewport(MdiCubeViewport *cvp)
This class needs to know which viewport the user is looking at so it can appropriately draw in the ba...
void replot()
Reset the scale of the plot, replot it and emit plot changed.
void drawBandMarkers()
This method actually draws in the vertical band line(s) on the plot area.
This is free and unencumbered software released into the public domain.