File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
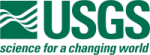 |
Isis 3 Programmer Reference
|
1 #ifndef SpicePosition_h
2 #define SpicePosition_h
17 #include "PolynomialUnivariate.h"
20 #include "ale/States.h"
22 #include <nlohmann/json.hpp>
25 class NumericalApproximation;
201 enum PartialType {WRT_X, WRT_Y, WRT_Z};
216 const std::vector<double> &
Velocity();
223 void LoadCache(
double startTime,
double endTime,
int size);
244 return m_state->getStates().size();
252 const std::vector<double>& YC,
253 const std::vector<double>& ZC,
257 std::vector<double>& YC,
258 std::vector<double>& ZC);
284 std::vector<double>
CoordinatePartial(SpicePosition::PartialType partialVar,
int coeffIndex);
286 std::vector<double>
VelocityPartial(SpicePosition::PartialType partialVar,
int coeffIndex);
287 enum OverrideType {NoOverrides, ScaleOnly, BaseAndScale};
300 SpicePosition(
int targetCode,
int observerCode,
bool swapObserverTarget);
305 const QString &refFrame,
306 const QString &abcorr,
307 double state[6],
bool &hasVelocity,
308 double &lightTime)
const;
309 void setStateVector(
const double state[6],
const bool &hasVelocity);
314 void init(
int targetCode,
int observerCode,
315 const bool &swapObserverTarget =
false);
350 bool m_swapObserverTarget;
bool p_degreeApplied
Flag indicating whether or not a polynomial.
@ HermiteCache
Object is reading from splined table.
void GetPolynomial(std::vector< double > &XC, std::vector< double > &YC, std::vector< double > &ZC)
Return the coefficients of a polynomial fit to each of the three coordinates of the position for the ...
QString p_aberrationCorrection
Light time correction to apply.
Obtain SPICE position information for a body.
std::vector< double > p_coordinate
J2000 position at time et.
void SetEphemerisTimePolyFunction()
This is a protected method that is called by SetEphemerisTime() when Source type is PolyFunction.
void ClearCache()
Removes the entire cache from memory.
double p_overrideBaseTime
Value set by caller to override computed base time.
int p_targetCode
target body code
virtual void SetEphemerisTimeSpice()
This is a protected method that is called by SetEphemerisTime() when Source type is Spice.
void computeStateVector(double et, int target, int observer, const QString &refFrame, const QString &abcorr, double state[6], bool &hasVelocity, double &lightTime) const
Computes the state vector of the target w.r.t observer.
virtual QString GetAberrationCorrection() const
Returns current state of stellar aberration correction.
virtual void SetAberrationCorrection(const QString &correction)
Set the aberration correction (light time)
void SetPolynomial(const Source type=PolyFunction)
Set the coefficients of a polynomial fit to each of the components (X, Y, Z) of the position vector f...
virtual ~SpicePosition()
Destructor.
Source GetSource()
Return the source of the position.
std::vector< double > HermiteCoordinate()
This method returns the Hermite coordinate for the current time for PolyFunctionOverHermiteConstant f...
std::vector< double > CoordinatePartial(SpicePosition::PartialType partialVar, int coeffIndex)
Set the coefficients of a polynomial fit to each of the three coordinates of the position vector for ...
double GetBaseTime()
Return the base time for the position.
std::vector< double > p_velocity
J2000 velocity at time et.
bool p_hasVelocity
Flag to indicate velocity is available.
double getAdjustedEphemerisTime() const
Returns adjusted ephemeris time.
const std::vector< double > & Coordinate()
Return the current J2000 position.
void SetTimeBias(double timeBias)
Apply a time bias when invoking SetEphemerisTime method.
int getTargetCode() const
Returns target code.
void ComputeBaseTime()
Compute the base time using cached times.
double GetTimeBias() const
Returns the value of the time bias added to ET.
double p_et
Current ephemeris time.
void setStateVector(const double state[6], const bool &hasVelocity)
Sets the state of target relative to observer.
void SetEphemerisTimeMemcache()
This is a protected method that is called by SetEphemerisTime() when Source type is Memcache.
SpicePosition(int targetCode, int observerCode)
Construct an empty SpicePosition class using valid body codes.
double p_timeBias
iTime bias when reading kernels
Table LineCache(const QString &tableName)
Return a table with J2000 to reference positions.
const std::vector< double > & SetEphemerisTime(double et)
Return J2000 coordinate at given time.
void SetEphemerisTimePolyFunctionOverHermiteConstant()
This is a protected method that is called by SetEphemerisTime() when Source type is PolyFunctionOverH...
void SetEphemerisTimeHermiteCache()
This is a protected method that is called by SetEphemerisTime() when Source type is HermiteCache.
@ Spice
Object is reading directly from the kernels.
double p_baseTime
Base time used in fit equations.
const std::vector< double > & GetCenterCoordinate()
Compute and return the coordinate at the center time.
bool HasVelocity()
Return the flag indicating whether the velocity exists.
Source p_source
Enumerated value for the location of the SPK information used.
@ PolyFunctionOverHermiteConstant
Object is reading from splined.
int getObserverCode() const
Returns observer code.
int p_degree
Degree of polynomial function fit to the coordinates of the position.
Table LoadHermiteCache(const QString &tableName)
Cache J2000 position over existing cached time range using polynomials stored as Hermite cubic spline...
double p_overrideTimeScale
Value set by caller to override computed time scale.
@ PolyFunction
Object is calculated from nth degree polynomial.
void LoadCache(double startTime, double endTime, int size)
Cache J2000 position over a time range.
double GetLightTime() const
Return the light time coorection value.
int cacheSize() const
Get the size of the current cached positions.
double p_timeScale
Time scale used in fit equations.
Class for storing Table blobs information.
int p_observerCode
observer body code
Source
This enum indicates the status of the object.
void setLightTime(const double &lightTime)
Inheritors can set the light time if indicated.
std::vector< double > Extrapolate(double timeEt)
Extrapolate position for a given time assuming a constant velocity.
double EphemerisTime() const
Return the current ephemeris time.
void CacheLabel(Table &table)
Add labels to a SpicePosition table.
void LoadTimeCache()
Load the time cache.
void Memcache2HermiteCache(double tolerance)
This method reduces the cache for position, time and velocity to the minimum number of values needed ...
std::vector< double > p_coefficients[3]
Coefficients of polynomials fit to 3 coordinates.
double m_lt
!< Swap traditional order
double p_fullCacheSize
Orignial size of the complete cache after spiceinit.
double p_fullCacheStartTime
Original start time of the complete cache after spiceinit.
void SetOverrideBaseTime(double baseTime, double timeScale)
Set an override base time to be used with observations on scanners to allow all images in an observat...
const std::vector< double > & Velocity()
Return the current J2000 velocity.
OverrideType p_override
Time base and scale override options;.
double p_fullCacheEndTime
Original end time of the complete cache after spiceinit.
bool IsCached() const
Is this position cached.
Table Cache(const QString &tableName)
Return a table with J2000 positions.
@ Memcache
Object is reading from cached table.
double ComputeVelocityInTime(PartialType var)
Compute the velocity with respect to time instead of scaled time.
void ReloadCache()
Cache J2000 positions over existing cached time range using polynomials.
std::vector< double > p_cacheTime
iTime for corresponding position
double GetTimeScale()
Return the time scale for the position.
double DPolynomial(const int coeffIndex)
Evaluate the derivative of the fit polynomial (parabola) defined by the given coefficients with respe...
This is free and unencumbered software released into the public domain.
ale::States * m_state
!< Light time correction
void init(int targetCode, int observerCode, const bool &swapObserverTarget=false)
Internal initialization of the object support observer/target swap.
std::vector< double > VelocityPartial(SpicePosition::PartialType partialVar, int coeffIndex)
Compute the derivative of the velocity with respect to the specified variable.
void SetPolynomialDegree(int degree)
Set the polynomial degree.