File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
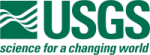 |
Isis 3 Programmer Reference
|
15 #include "IException.h"
66 bool hasField(
const QString &name)
const;
70 QString
getType(
int index)
const;
71 QString
getType(
const QString &name)
const;
73 bool isNull(
const QString &name)
const;
75 QString
getValue(
const QString &name)
const;
SqlRecord()
Default Constructor.
bool hasField(const QString &name) const
Indicates the existance/non-existance of a field in the row.
QString QtTypeField(const char *ctype) const
Returns a generic field type given a Qt QVariant type.
QString getType(int index) const
Returns the type of a field/column at the specified index.
int getFieldIndex(const QString &name) const
Return the index of a named field/column This method will determine the index of the named field afte...
int size() const
Returns the number of fields/columns in query.
QString getValue(int index) const
Returns the value of the field/column at specified index.
bool isNull(const QString &name) const
Determines if the value of the field/column is NULL.
QString getFieldName(int index) const
Returns the name of a field/column at a particular index.
Construct and execute a query on a database and manage result.
This is free and unencumbered software released into the public domain.
Provide simplified access to resulting SQL query row.