Loading [MathJax]/jax/output/NativeMML/config.js
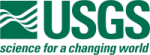 |
Isis 3 Programmer Reference
|
18 class HistogramWidget;
44 const QString &name,
const QColor &color);
77 void deleteFromCube();
78 void stretchChanged();
virtual void setHistogram(const Histogram &)
This should be called when the visible area changes.
virtual void setStretch(Stretch)=0
Children must re-implement this to update their stretch pairs and GUI elements appropriately.
void updateGraph()
This updates the graph with the current stretch object.
HistogramWidget * p_graph
Histogram graph.
StretchType(const Histogram &hist, const Stretch &stretch, const QString &name, const QColor &color)
This constructs a stretch type.
This is the base class for advanced stretches.
Stores stretch information for a cube.
virtual ~StretchType()
Destructor.
void updateTable()
This updates the table with the current stretch pairs.
Histogram * p_cubeHist
Visible area histogram.
virtual CubeStretch getStretch()
Returns the current stretch object.
void savePairs()
This asks the user for a file and saves the current stretch pairs to that file.
QGridLayout * p_mainLayout
Main layout.
void saveToCube()
Emitted when a new Stretch object is available.
QTableWidget * createStretchTable()
This creates the stretch pairs table.
Container of a cube histogram.
Stretch * p_stretch
Current stretch pairs stored here.
QTableWidget * p_table
Pairs table.
This is free and unencumbered software released into the public domain.