Loading [MathJax]/jax/output/NativeMML/config.js
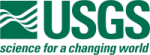 |
Isis 3 Programmer Reference
|
76 operator double()
const;
77 operator float()
const;
78 operator QString()
const;
79 operator std::vector<int>()
const;
80 operator std::vector<double>()
const;
81 operator std::vector<float>()
const;
87 void operator=(
const std::vector<int> &values);
88 void operator=(
const std::vector<double> &values);
89 void operator=(
const std::vector<float> &value);
95 static QString toString(
const TableField &field, QString delimiter =
",");
Type type() const
Returns the enumerated value of the TableField value's type.
Type m_type
Field value type.
bool isInteger() const
Determines whether the field type is Integer.
TableField(const QString &name, Type type, int size=1)
Constructs a TableField object with the given field name, field value type, and field size.
Contains multiple PvlContainers.
bool isReal() const
Determines whether the field type is Text.
int bytes() const
Returns the number of bytes in the field value.
@ Integer
The values in the field are 4 byte integers.
QString m_name
Field name.
std::vector< int > m_ivalues
Vector containing integer field values.
@ Double
The values in the field are 8 byte doubles.
~TableField()
Destroys the TableField object.
QString m_svalue
String containing text value of field.
bool isDouble() const
Determines whether the field type is Double.
int size() const
Returns the number of values stored for the field at each record.
QString name() const
Returns the name of the TableField.
PvlGroup pvlGroup()
Creates and returns a PvlGroup named "Field" containing the following keywords and their respective v...
std::vector< double > m_dvalues
Vector containing double field values.
bool isText() const
Determines whether the field type is Text.
std::vector< float > m_rvalues
Vector containing Real field values.
@ Real
The values in the field are 4 byte reals or floats.
@ Text
The values in the field are text strings with 1 byte per character.
void operator=(const int value)
Sets field value equal to input if TableField::Type is Integer and size is 1.
Type
This enum describes the value type for the TableField.
int m_bytes
Number of bytes in field.
This is free and unencumbered software released into the public domain.
Class for storing an Isis::Table's field information.