File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
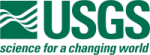 |
Isis 3 Programmer Reference
|
9 #include "TargetBodyList.h"
16 #include <QInputDialog>
18 #include <QProgressDialog>
19 #include <QtConcurrentMap>
20 #include <QXmlStreamWriter>
23 #include "IException.h"
26 #include "TargetBody.h"
27 #include "XmlStackedHandlerReader.h"
121 emit countChanged(count());
134 emit countChanged(count());
144 bool countChanging = count();
147 emit countChanged(count());
161 emit countChanged(count());
180 emit countChanged(count());
196 emit countChanged(count());
213 emit countChanged(count());
227 emit countChanged(count());
240 emit countChanged(count());
253 emit countChanged(count());
270 emit countChanged(count());
286 emit countChanged(count());
297 emit countChanged(count());
308 emit countChanged(count());
325 emit countChanged(count());
342 if (count() != other.count()) {
343 emit countChanged(count());
359 emit countChanged(count());
373 emit countChanged(count());
387 emit countChanged(count());
405 emit countChanged(count());
423 emit countChanged(count());
441 emit countChanged(count());
459 emit countChanged(count());
474 bool countChanging = (rhs.count() != count());
478 emit countChanged(count());
493 bool countChanging = (rhs.count() != count());
500 emit countChanged(count());
880 const QString &localName,
881 const QString &qName,
882 const QXmlAttributes &atts) {
883 if (XmlStackedHandler::startElement(namespaceURI, localName, qName, atts)) {
884 if (localName ==
"TargetBodyList") {
885 QString
name = atts.value(
"name");
886 QString
path = atts.value(
"path");
888 if (!
name.isEmpty()) {
889 m_TargetBodyList->setName(
name);
892 if (!
path.isEmpty()) {
893 m_TargetBodyList->setPath(
path);
896 else if (localName ==
"target") {
921 const QString &localName,
922 const QString &qName) {
923 if (localName ==
"TargetBodyList") {
924 XmlHandler handler(m_TargetBodyList, m_project);
928 reader.setErrorHandler(&handler);
930 QString TargetBodyListXmlPath = m_project->targetBodyRoot() +
"/" +
931 m_TargetBodyList->path() +
"/targets.xml";
932 QFile file(TargetBodyListXmlPath);
934 if (!file.open(QFile::ReadOnly)) {
936 QString(
"Unable to open [%1] with read access")
937 .arg(TargetBodyListXmlPath),
941 QXmlInputSource xmlInputSource(&file);
942 if (!reader.parse(xmlInputSource))
944 tr(
"Failed to open target body list XML [%1]").arg(TargetBodyListXmlPath),
948 return XmlStackedHandler::endElement(namespaceURI, localName, qName);
TargetBodyList & operator=(const QList< TargetBodyQsp > &rhs)
Assignment operator for a QList of TargetBodyQsp.
TargetBodyList(QString name, QString path, QObject *parent=NULL)
Create an target body list from an target body list name and path (does not read TargetBody objects).
@ Io
A type of error that occurred when performing an actual I/O operation.
QString m_name
This functor is used for copying the TargetBody objects between two projects quickly.
TargetBodyQsp takeAt(int i)
Removes and returns the TargetBody at a specific index.
void save(QXmlStreamWriter &stream, const Project *project, FileName newProjectRoot) const
Delete all of the contained TargetBody objects from disk (see TargetBody::deleteFromDisk())
Project * m_project
The project that contains the TargetBodies.
TargetBodyQsp takeLast()
Removes and returns the last TargetBody in the list.
File name manipulation and expansion.
virtual void pushContentHandler(XmlStackedHandler *newHandler)
Push a contentHandler and maybe continue parsing...
TargetBodyList & operator+=(const QList< TargetBodyQsp > &other)
Appends another TargetBodyList to the list.
void push_back(TargetBodyQsp const &value)
Appends a TargetBody to the end of the list.
void setPath(QString newPath)
Set the relative path (from the project root) to this target body list's folder.
Manage a stack of content handlers for reading XML files.
void push_front(TargetBodyQsp const &value)
Insertes a TargetBody at the front of the list.
XmlHandler(TargetBodyList *TargetBodyList, Project *project)
Change the visibility of the display name.
void removeAt(int i)
Removes the TargetBody at a specific index.
The main project for ipce.
void removeLast()
Removes the last TargetBody from the list.
XmlReader for working with TargetBody XML files.
void insert(int i, TargetBodyQsp const &value)
Inserts a TargetBody at a specific index.
TargetBodyList & operator<<(const QList< TargetBodyQsp > &other)
Appends another TargetBodyList to the list.
List for holding TargetBodies.
void removeFirst()
Removes the first TargetBody from the list.
void clear()
clears the list.
virtual bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts)
Handle an XML start element.
~TargetBodyList()
Create an target body list from a list of target body file names.
QString m_path
This stores the directory name that contains the TargetBody objects in this list.
QString name() const
Get the human-readable name of this target body list.
void append(TargetBodyQsp const &value)
Appends a TargetBody to the list.
QString path() const
Get the path to these target body objects in the list (relative to project root).
void setName(QString newName)
Gets a list of pre-connected actions that have to do with display, such as color, alpha,...
int removeAll(TargetBodyQsp const &value)
Removes all occurrences of a TargetBody and returns the number removed.
void swap(QList< TargetBodyQsp > &other)
Swaps the list with another TargetBodyList.
TargetBodyList * m_TargetBodyList
The TargetBodyList to read into/save from.
virtual bool endElement(const QString &namespaceURI, const QString &localName, const QString &qName)
Handle an XML end element.
void prepend(TargetBodyQsp const &value)
Inserts a TargetBody at the front of the list.
iterator erase(iterator pos)
Erases the TargetBody associated with an iterator.
This is free and unencumbered software released into the public domain.
TargetBodyQsp takeFirst()
Removes and returns the first TargetBody in the list.
bool removeOne(TargetBodyQsp const &value)
Removes the first occurrence of a TargetBody from the list.