Loading [MathJax]/jax/output/NativeMML/config.js
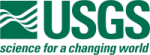 |
Isis 3 Programmer Reference
|
9 #include "TemplateList.h"
13 #include "IException.h"
15 #include "XmlStackedHandlerReader.h"
49 m_path = other.m_path;
50 m_name = other.m_name;
51 m_type = other.m_type;
144 foreach (
Template *currentTemplate, *
this) {
148 if (!m_path.isEmpty()) {
149 QFile::remove(project->
templateRoot() +
"/" + m_path +
"/templates.xml");
183 if (m_type ==
"maps") {
184 stream.writeStartElement(
"mapTemplateList");
186 else if (m_type ==
"registrations") {
187 stream.writeStartElement(
"regTemplateList");
191 QString(
"Attempting to save unsupported template file type: [%1]").arg(m_type),
194 stream.writeAttribute(
"name", m_name);
195 stream.writeAttribute(
"type", m_type);
196 stream.writeAttribute(
"path", m_path);
199 +
"/" + m_type +
"/" + m_name +
"/templates.xml");
201 if (!settingsFileName.
dir().mkpath(settingsFileName.
path())) {
203 QString(
"Failed to create directory [%1]").arg(settingsFileName.
path()),
207 QFile templateListContentsFile(settingsFileName.
toString());
209 if (!templateListContentsFile.open(QIODevice::ReadWrite | QIODevice::Truncate)) {
211 QString(
"Unable to save template information for [%1] because [%2] could not be opened "
213 .arg(m_name).arg(settingsFileName.
original()),
217 QXmlStreamWriter templateDetailsWriter(&templateListContentsFile);
218 templateDetailsWriter.setAutoFormatting(
true);
219 templateDetailsWriter.writeStartDocument();
220 templateDetailsWriter.writeStartElement(
"templates");
222 foreach (
Template *currentTemplate, *
this) {
223 currentTemplate->
save(templateDetailsWriter, project, newProjectRoot);
225 QString newPath = newProjectRoot.
toString() +
"/templates/" + m_type +
"/" + m_name;
229 QFile::copy(currentTemplate->
fileName(),
234 templateDetailsWriter.writeEndElement();
235 templateDetailsWriter.writeEndDocument();
237 stream.writeEndElement();
266 const QString &qName,
const QXmlAttributes &atts) {
267 if (XmlStackedHandler::startElement(namespaceURI, localName, qName, atts)) {
268 if (localName ==
"mapTemplateList" || localName ==
"regTemplateList") {
269 QString
name = atts.value(
"name");
270 QString
type = atts.value(
"type");
271 QString
path = atts.value(
"path");
273 if (!
name.isEmpty()) {
274 m_templateList->setName(
name);
277 if (!
type.isEmpty()) {
278 m_templateList->setType(
type);
281 if (!
path.isEmpty()) {
282 m_templateList->setPath(
path);
285 else if (localName ==
"template") {
286 m_templateList->append(
new Template(m_project->templateRoot(), reader()));
308 const QString &qName) {
309 if (localName ==
"mapTemplateList" || localName ==
"regTemplateList") {
310 XmlHandler handler(m_templateList, m_project);
314 reader.setErrorHandler(&handler);
316 QString templateListXmlPath = m_project->templateRoot() +
"/" + m_templateList->type() +
"/"
317 + m_templateList->name() +
"/templates.xml";
318 templateListXmlPath = QDir::cleanPath(templateListXmlPath);
320 QFile file(templateListXmlPath);
322 if (!file.open(QFile::ReadOnly)) {
324 QString(
"Unable to open [%1] with read access")
325 .arg(templateListXmlPath),
329 QXmlInputSource xmlInputSource(&file);
330 if (!reader.parse(xmlInputSource))
332 tr(
"Failed to open TemplateList XML [%1]").arg(templateListXmlPath),
336 return XmlStackedHandler::endElement(namespaceURI, localName, qName);
void save(QXmlStreamWriter &stream, const Project *project, FileName newProjectRoot) const
Convert this TemplateList into XML format for saving/restoring capabilities.
static QString templateRoot(QString projectRoot)
Appends the root directory name 'templates' to the project .
@ Io
A type of error that occurred when performing an actual I/O operation.
This is free and unencumbered software released into the public domain.
virtual bool startElement(const QString &namespaceURI, const QString &localName, const QString &qName, const QXmlAttributes &atts)
Handle an XML start element.
QString name() const
Returns the name of the file excluding the path and the attributes in the file name.
File name manipulation and expansion.
virtual void pushContentHandler(XmlStackedHandler *newHandler)
Push a contentHandler and maybe continue parsing...
virtual bool endElement(const QString &namespaceURI, const QString &localName, const QString &qName)
Handle an XML end element.
QString path() const
Get the path to these Templates in the TemplateList (relative to project root).
void deleteFromDisk()
Delete the template from disk.
Manage a stack of content handlers for reading XML files.
The main project for ipce.
void save(QXmlStreamWriter &stream, const Project *project, FileName newProjectRoot) const
Method to write this Template object's member data to an XML stream.
QDir dir() const
Returns the path of the file's parent directory as a QDir object.
QString fileName() const
Get the file name that this Template represents.
void deleteFromDisk(Project *project)
Delete all of the contained Templates from disk.
~TemplateList()
Destructor.
TemplateList(QString name, QString type, QString path, QObject *parent=NULL)
Create a template from a file name, type, and path.
QString toString() const
Returns a QString of the full file name including the file path, excluding the attributes with any Is...
TemplateList * m_templateList
TemplateList to be read or written.
QString name() const
Get the human-readable name of this TemplateList.
QString templateRoot() const
Accessor for the root directory of the template data.
QString type() const
Get the type of template in this TemplateList.
XmlHandler(TemplateList *templateList, Project *project)
Create an XML Handler (reader/writer) that can populate the TemplateList class data.
void setType(QString newType)
Set the type of template for of this TemplateList.
void setPath(QString newPath)
Set the relative path (from the project root) to this TemplateList's folder.
QString original() const
Returns the full file name including the file path.
Project * m_project
Project that contains the template list.
void setName(QString newName)
Set the human-readable name of this TemplateList.
QString path() const
Returns the path of the file name.
This is free and unencumbered software released into the public domain.