File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
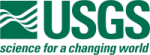 |
Isis 3 Programmer Reference
|
1 #ifndef UserInterface_h
2 #define UserInterface_h
12 #include "PvlTokenizer.h"
142 UserInterface(
const QString &xmlfile,
int &argc,
char *argv[]);
172 return p_gui != NULL;
208 QString &unresolvedParam,
209 std::vector<QString> &value);
210 void preProcess(QString fullReservedName, std::vector<QString> &reservedParams);
211 std::vector<QString>
readArray(QString arrayString);
213 std::vector<QString> &reservedParams,
214 bool handleNoMatches =
true);
bool IsInteractive()
Indicates if the Isis Graphical User Interface is operating.
QString GetInfoFileName()
This method returns the filename where the debugging info is stored when the "-info" tag is used.
void evaluateOption(const QString name, const QString value)
This interprets the "-" options for reserved parameters.
QString p_errList
FileName to write batchlist line that caused error on.
bool p_info
Boolean value representing if it's in debug mode.
void SetErrorList(int i)
This method adds the line specified in the BatchList that the error occured on.
std::vector< std::vector< QString > > p_batchList
Vector of batchlist data.
bool p_interactive
Boolean value representing whether the program is interactive or not.
int ParentId()
Returns the parent id.
bool GetInfoFlag()
This method returns the flag state of info.
Gui for Isis Applications.
QString p_infoFileName
FileName to save debugging info.
std::vector< QString > readArray(QString arrayString)
This interprets an array value from the command line.
void loadBatchList(const QString file)
Loads the user entered batchlist file into a private variable for later use.
int BatchListSize()
Returns the size of the batchlist.
int p_parentId
This is a status to indicate if the GUI is running or not.
~UserInterface()
Destroys the UserInterface object.
Gui * p_gui
Pointer to the gui object.
void loadCommandLine(QVector< QString > &args, bool ignoreAppName=true)
This is used to load the command line into p_cmdline and the Aml object using information contained i...
QString p_progName
Name of program to run.
Application program XML file parameter manager.
void SetBatchList(int i)
Clears the gui parameters and sets the batch list information at line i as the new parameters.
std::vector< char * > p_cmdline
This variable will contain argv.
bool AbortOnError()
Returns true if the program should abort on error, and false if it should continue.
void getNextParameter(unsigned int &curPos, QString &unresolvedParam, std::vector< QString > &value)
This gets the next parameter in the list of arguments.
void preProcess(QString fullReservedName, std::vector< QString > &reservedParams)
This parses the command line and looks for the specified reserved parameter name passed.
QString p_saveFile
FileName to save last history to.
void SaveHistory()
Saves the user parameter information in the history of the program for later use.
Command Line and Xml loader, validation, and access.
QString resolveParameter(QString &name, std::vector< QString > &reservedParams, bool handleNoMatches=true)
This resolves a reserved parameter token on the command line to its fullname.
bool p_abortOnError
Boolean value representing whether to abort or continue on error.
UserInterface(const QString &xmlfile, int &argc, char *argv[])
Constructs an UserInterface object.
This is free and unencumbered software released into the public domain.
void loadHistory(const QString file)
Loads the previous history for the program.