Loading [MathJax]/jax/output/NativeMML/config.js
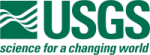 |
Isis 3 Programmer Reference
|
9 #include "ViewportBufferFill.h"
30 ViewportBufferFill::ViewportBufferFill(
const QRect &rect,
31 const int &xCoef,
const double &xScale,
const int &yCoef,
32 const double &yScale,
const QPoint &topLeftPixel)
120 linesToPaint =
p_rect->height();
bool doneReading()
Returns true if read position is past the end of the fill.
QRect * p_rect
Rect this fill represents.
int p_yCoef
viewport to sample/line y coef
static const int STEPSIZE
how many cube lines per paint if painting inbetween gets re-enabled
bool shouldPaint(int &linesToPaint)
Returns true if it is recommended to paint the fill area so far.
int getTopmostPixelPosition()
Returns the top of the X/Y bounding rect for this fill.
bool shouldRequestMore()
Returns true if request position is past the end of the fill.
int p_xCoef
viewport to sample/line x coef
int getLeftmostPixelPosition()
Returns the left of the X/Y bounding rect for this fill.
double p_xScale
viewport to sample/line x scalar
unsigned int p_requestPosition
Position of the cube requests.
Namespace for the standard library.
unsigned int p_readPosition
Position of the cube reads.
void stop()
Cancels the current operation.
QPoint * p_topLeftPixel
Top left of the viewport for this fill.
double p_yScale
viewport to sample/line y scalar
This is free and unencumbered software released into the public domain.
~ViewportBufferFill()
Destructor.