Loading [MathJax]/jax/output/NativeMML/config.js
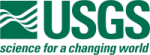 |
Isis 3 Programmer Reference
|
69 iTime(
const QString &time);
97 iTime operator +(
const double &secondsToAdd)
const;
98 void operator +=(
const double &secondsToAdd);
99 friend iTime operator +(
const double &secondsToAdd,
iTime time);
101 iTime operator -(
const double &secondsToSubtract)
const;
102 double operator -(
const iTime &iTimeToSubtract)
const;
103 void operator -=(
const double &secondsToSubtract);
104 friend iTime operator -(
const double &secondsToSubtract,
iTime time);
130 QString
UTC(
int precision=8)
const;
134 void setEt(
double et);
135 void setUtc(QString utcString);
143 static bool p_lpInitialized;
iTime()
Constructs an empty iTime object.
bool operator>(const iTime &time)
Compare two iTime objects for greater than.
bool operator!=(const iTime &time)
Compare two iTime objects for inequality.
Parse and return pieces of a time string.
bool operator<=(const iTime &time)
Compare two iTime objects for less than or equal.
QString EtString() const
Returns the ephemeris time (TDB) representation of the time as a string.
bool operator<(const iTime &time)
Compare two iTime objects for less than.
int Minute() const
Returns the minute portion of the time as an int.
static QString CurrentLocalTime()
Returns the current local time This time is taken directly from the system clock, so if the system cl...
bool operator==(const iTime &time)
Compare two iTime objects for equality.
int Month() const
Returns the month portion of the time as an int.
QString SecondString(int precision=8) const
Returns the second portion of the time as a string.
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
iTime(const double time)
Constructs a iTime object and initializes it to the time from the argument.
int Year() const
Returns the year portion of the time as an int.
static QString CurrentGMT()
Returns the current Greenwich Mean iTime The time is based on the system time, so it is only as accur...
QString MonthString() const
Returns the month portion of the time as a string.
void LoadLeapSecondKernel()
Uses the Naif routines to load the most current leap second kernel.
QString MinuteString() const
Returns the minute portion of the time as a string.
QString DayString() const
Returns the dat portion of the time as a string.
int Hour() const
Returns the hour portion of the time as an int.
double Second() const
Returns the second portion of the time as a double.
double p_et
The ephemeris representaion of the original string passed into the constructor or the operator= membe...
int Day() const
Returns the day portion of the time as an int.
QString DayOfYearString() const
Returns the day of year portion of the time as a string.
QString HourString() const
Returns the hour portion of the time as a string.
void operator=(const QString &time)
Changes the value of the iTime object.
QString YearString() const
Returns the year portion of the time as a string.
QString UTC(int precision=8) const
Returns the internally stored time, formatted as a UTC time.
bool operator>=(const iTime &time)
Compare two iTime objects for greater than or equal.
This is free and unencumbered software released into the public domain.
int DayOfYear() const
Returns the day of year portion of the time as an int.