File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
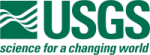 |
Isis 3 Programmer Reference
|
1 #include "AdvancedStretchDialog.h"
7 #include "CubeViewport.h"
9 #include "CubeStretch.h"
10 #include "AdvancedStretch.h"
26 setWindowTitle(
"Advanced Stretch Tool");
28 QVBoxLayout *layout =
new QVBoxLayout();
58 QHBoxLayout* rgbLayout =
new QHBoxLayout();
61 "Red", QColor(Qt::red));
65 "Green", QColor(Qt::green));
69 "Blue", QColor(Qt::blue));
72 ((QVBoxLayout*)layout())->addLayout(rgbLayout);
75 line->setFrameShape(QFrame::HLine);
76 line->setFrameShadow(QFrame::Sunken);
77 ((QVBoxLayout*)layout())->addWidget(line);
89 QPushButton *saveToCubeButton =
new QPushButton(
"Save Stretch Pairs to Cube...");
90 saveToCubeButton->setSizePolicy(QSizePolicy::Fixed, QSizePolicy::Fixed);
91 connect(saveToCubeButton, SIGNAL(clicked(
bool)),
this, SIGNAL(saveToCube()));
93 QPushButton *deleteFromCubeButton =
new QPushButton(
"Delete Stretch Pairs from Cube...");
94 deleteFromCubeButton->setSizePolicy(QSizePolicy::Fixed, QSizePolicy::Fixed);
95 connect(deleteFromCubeButton, SIGNAL(clicked(
bool)),
this, SIGNAL(deleteFromCube()));
97 QPushButton *loadStretchButton =
new QPushButton(
"Restore Saved Stretch from Cube...");
98 loadStretchButton->setSizePolicy(QSizePolicy::Fixed, QSizePolicy::Fixed);
99 connect(loadStretchButton, SIGNAL(clicked(
bool)),
this, SIGNAL(loadStretch()));
101 QHBoxLayout* buttonLayout =
new QHBoxLayout();
103 buttonLayout->addWidget(saveToCubeButton);
104 buttonLayout->addWidget(deleteFromCubeButton);
105 buttonLayout->addWidget(loadStretchButton);
106 ((QBoxLayout*)layout())->addLayout(buttonLayout);
156 "Gray", QColor(Qt::gray));
163 this, SIGNAL(saveToCube()));
165 this, SIGNAL(deleteFromCube()));
167 this, SIGNAL(loadStretch()));
181 QString msg =
"Gray mode not enabled, cannot restore gray stretch";
209 QString msg =
"RGB mode not enabled, cannot restore RGB stretch";
318 QDialog::showEvent(event);
329 QDialog::hideEvent(event);
355 QString msg =
"Gray mode not enabled, cannot get gray stretch";
371 QString msg =
"RGB mode not enabled, cannot get red stretch";
387 QString msg =
"RGB mode not enabled, cannot get green stretch";
403 QString msg =
"RGB mode not enabled, cannot get blue stretch";
bool isRgbMode() const
Returns true if the dialog is displaying the RGB advanced stretches.
void restoreRgbStretch(CubeStretch red, CubeStretch green, CubeStretch blue)
Restores a saved RGB stretch from the cube.
void updateHistogram(const Histogram &grayHist)
This calls setHistogram on the gray advanced stretches.
void hideEvent(QHideEvent *)
This is implemented to send a signal when visibility changes.
CubeStretch getGrayStretch()
This returns the advanced stretch's stretch for gray.
void updateStretch(CubeViewport *)
This calls setStretch on all applicable advanced stretches.
CubeStretch getStretch()
This returns the current stretch type's stretch.
void setHistogram(const Histogram &newHist)
This is called when the visible area changes, so that the histogram can be updated.
void restoreSavedStretch(CubeStretch newStretch)
Used to restore a saved Stretch from a cube.
void visibilityChanged()
Emitted when this dialog is shown or hidden.
Stores stretch information for a cube.
CubeStretch greenStretch() const
Return the green band stretch.
AdvancedStretch * p_grnStretch
Green stretch pane.
CubeStretch redStretch() const
Return the red band stretch.
void stretchChanged()
Emitted when an advanced stretch has changed.
CubeStretch getBluStretch()
This returns the advanced stretch's stretch for blue.
void restoreGrayStretch(CubeStretch stretch)
Restores a saved grayscale stretch from the cube.
CubeStretch blueStretch() const
Return the blue band stretch.
void updateHistograms(const Histogram &redHist, const Histogram &grnHist, const Histogram &bluHist)
This calls setHistogram on all of the advanced stretches.
bool p_enabled
True if advanced stretch should be used.
CubeStretch grayStretch() const
Return the gray band stretch.
AdvancedStretch * p_bluStretch
Blue stretch pane.
CubeStretch getRedStretch()
This returns the advanced stretch's stretch for red.
AdvancedStretch * p_redStretch
Red stretch pane.
AdvancedStretchDialog(QWidget *parent)
This constructs an advanced stretch.
Widget to display Isis cubes for qt apps.
void showEvent(QShowEvent *)
This is implemented to send a signal when visibility changes.
@ Programmer
This error is for when a programmer made an API call that was illegal.
void enableGrayMode(Stretch &grayStretch, Histogram &grayHist)
This displays a gray advanced stretch.
void updateForRGBMode(Stretch &redStretch, Histogram &redHist, Stretch &grnStretch, Histogram &grnHist, Stretch &bluStretch, Histogram &bluHist)
Update the stretch and histogram for all the bands for All BandId option.
Container of a cube histogram.
~AdvancedStretchDialog()
This destroys the advanced stretch dialog.
AdvancedStretch * p_grayStretch
Gray stretch pane.
void setStretch(Stretch newStretch)
This is called when the user creates a stretch outside of the advanced stretch.
CubeStretch getGrnStretch()
This returns the advanced stretch's stretch for green.
void destroyCurrentStretches()
This cleans up memory from currently displayed advanced stretches.
This is free and unencumbered software released into the public domain.
void enableRgbMode(Stretch &redStretch, Histogram &redHist, Stretch &grnStretch, Histogram &grnHist, Stretch &bluStretch, Histogram &bluHist)
This displays RGB advanced stretches.