File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
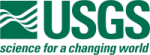 |
Isis 3 Programmer Reference
|
10 #include "CubeViewport.h"
14 #include <QApplication>
16 #include <QCloseEvent>
23 #include <QMessageBox>
24 #include <QMouseEvent>
32 #include "CubeDataThread.h"
33 #include "IException.h"
36 #include "Histogram.h"
39 #include "PvlKeyword.h"
40 #include "PvlObject.h"
42 #include "CubeStretch.h"
43 #include "StretchTool.h"
45 #include "UniversalGroundMap.h"
46 #include "ViewportBuffer.h"
65 "Can not view NULL cube pointer",
70 "Can not view unopened cube",
94 p_trackingCube = NULL;
108 setAttribute(Qt::WA_DeleteOnClose);
109 setHorizontalScrollBarPolicy(Qt::ScrollBarAlwaysOn);
110 setVerticalScrollBarPolicy(Qt::ScrollBarAlwaysOn);
111 viewport()->setObjectName(
"viewport");
112 viewport()->setCursor(QCursor(Qt::CrossCursor));
113 viewport()->installEventFilter(
this);
114 viewport()->setAttribute(Qt::WA_OpaquePaintEvent);
116 setAttribute(Qt::WA_NoSystemBackground);
117 setFrameShadow(QFrame::Plain);
118 setFrameShape(QFrame::NoFrame);
119 setAutoFillBackground(
false);
176 p_whatsThisText = QString(
"<b>Function: </b>Viewport to ") + cubeFileName;
179 "<p><b>Cube Dimensions:</b> \
180 <blockQuote>Samples = " +
221 p_image =
new QImage(viewport()->size(), QImage::Format_RGB32);
251 QAbstractScrollArea::show();
264 double progress = 0.0;
265 bool completed =
false;
287 int realProgress = (int)(progress * 100.0);
295 else if(realProgress == 100) {
370 delete p_trackingCube;
371 p_trackingCube = NULL;
385 delete(*p_pixmapPaintRects)[rect];
395 delete(*p_knownStretches)[stretch];
396 (*p_knownStretches)[stretch] = NULL;
409 delete(*p_globalStretches)[stretch];
410 (*p_globalStretches)[stretch] = NULL;
459 double ss, sl, es, el;
470 if(es >
cube()->sampleCount() + 0.5){
473 if(el >
cube()->lineCount() + 0.5){
487 if(ex > viewport()->width()){
488 ex = viewport()->width();
490 if(ey > viewport()->height()){
491 ey = viewport()->height();
493 QRect vpRect(sx, sy, ex - sx + 1, ey - sy + 1);
526 bool canClose =
true;
529 switch(QMessageBox::information(
this, tr(
"Confirm Save"),
530 tr(
"The cube [<font color='red'>%1</font>] contains unsaved changes. "
531 "Do you want to save the changes before exiting?").arg(
cube()->fileName()),
532 QMessageBox::Save | QMessageBox::Discard | QMessageBox::Cancel)) {
534 case QMessageBox::Save:
539 case QMessageBox::Discard:
544 case QMessageBox::Cancel:
594 if (viewport()->width() && viewport()->height()) {
596 double maxScale = max(viewport()->width(),viewport()->height());
597 if (
scale > maxScale) {
602 if (
scale < minScale) {
609 contentsToCube(horizontalScrollBar()->value(), verticalScrollBar()->value(),
643 viewport()->repaint();
672 viewport()->setUpdatesEnabled(
false);
674 bool wasEnabled =
false;
717 viewport()->setUpdatesEnabled(
true);
718 viewport()->update();
747 int panX = horizontalScrollBar()->value() - x;
748 int panY = verticalScrollBar()->value() - y;
799 double &sample,
double &line)
const {
816 double &sample,
double &line)
const {
817 x += horizontalScrollBar()->value();
818 x -= viewport()->width() / 2;
819 y += verticalScrollBar()->value();
820 y -= viewport()->height() / 2;
836 int &x,
int &y)
const {
837 x = (int)(sample *
p_scale + 0.5);
838 y = (int)(line *
p_scale + 0.5);
853 int &x,
int &y)
const {
855 x -= horizontalScrollBar()->value();
856 x += viewport()->width() / 2;
857 y -= verticalScrollBar()->value();
858 y += viewport()->height() / 2;
871 int x = horizontalScrollBar()->value() + dx;
873 dx = 1 - horizontalScrollBar()->value();
875 else if(x >= horizontalScrollBar()->maximum()) {
876 dx = horizontalScrollBar()->maximum() - horizontalScrollBar()->value();
880 int y = verticalScrollBar()->value() + dy;
882 dy = 1 - verticalScrollBar()->value();
884 else if(y >= verticalScrollBar()->maximum()) {
885 dy = verticalScrollBar()->maximum() - verticalScrollBar()->value();
889 if((dx == 0) && (dy == 0)){
895 verticalScrollBar()->value() + dy);
912 if(viewport()->signalsBlocked()) {
924 bool panQueued =
false;
963 viewport()->update();
987 QString str = QFileInfo(cubeFileName).fileName();
988 str += QString(
" @ ");
989 str += QString::number(
p_scale * 100.0);
990 str += QString(
"% ");
993 str += QString(
"(RGB = ");
1002 str += QString(
"(Gray = ");
1004 str += QString(
")");
1012 parentWidget()->setWindowTitle(str);
1054 p_image =
new QImage(viewport()->size(), QImage::Format_RGB32);
1055 p_pixmap = QPixmap(viewport()->size());
1061 verticalScrollBar()->value());
1064 "<p><b>Viewport Dimensions:</b> \
1065 <blockQuote>Samples = " +
1066 QString::number(viewport()->width()) +
"<br>" +
1068 QString::number(viewport()->height()) +
"</blockquote></p>";
1074 viewport()->update();
1125 viewport()->repaint(rect);
1168 for(
int y = dataArea.top();
1169 !dataArea.isNull() && y <= dataArea.bottom();
1171 const vector< double > & line =
1174 if(line.size() == 0) {
1182 QRgb *rgb = (QRgb *)
p_image->scanLine(y);
1188 for(
int x = dataArea.left(); x <= dataArea.right(); x++) {
1190 int bufferX = x - bufferLeft;
1192 if(bufferX >= (
int)line.size()){
1204 double bufferVal = line.at(bufferX);
1211 rgb[x] = qRgb(redPix, greenPix, bluePix);
1228 "Buffer rects mismatched",
1237 "Buffer rects mismatched",
1243 for(
int y = dataArea.top();
1244 !dataArea.isNull() && y <= dataArea.bottom();
1252 if((
int)redLine.size() < dataArea.width() ||
1253 (
int)greenLine.size() < dataArea.width() ||
1254 (
int)blueLine.size() < dataArea.width()) {
1256 "Empty buffer line",
1260 QRgb *rgb = (QRgb *)
p_image->scanLine(y);
1266 for(
int x = dataArea.left(); x <= dataArea.right(); x++) {
1271 rgb[x] = qRgb(redPix, greenPix, bluePix);
1277 if(!dataArea.isNull()){
1278 p.drawImage(dataArea.topLeft(), *
p_image, dataArea);
1298 int drawStartX = dx;
1299 int pixmapStartX = 0;
1300 if(drawStartX < 0) {
1305 int drawStartY = dy;
1306 int pixmapStartY = 0;
1313 int pixmapDrawWidth =
p_pixmap.width() - pixmapStartX + 1;
1314 int pixmapDrawHeight =
p_pixmap.height() - pixmapStartY + 1;
1317 QPixmap pixmapCopy =
p_pixmap.copy();
1320 painter.fillRect(rect, QBrush(
p_bgColor));
1321 painter.drawPixmap(drawStartX, drawStartY,
1323 pixmapStartX, pixmapStartY,
1324 pixmapDrawWidth, pixmapDrawHeight);
1332 xFillRect = QRect(QPoint(0, 0),
1337 xFillRect = QRect(QPoint(
p_pixmap.width() + dx, 0),
1344 yFillRect = QRect(QPoint(0, 0),
1349 yFillRect = QRect(QPoint(0,
p_pixmap.height() + dy),
1362 viewport()->update();
1378 PvlGroup cubeGrp(
"CubeDimensions");
1382 whatsThisObj += cubeGrp;
1385 PvlGroup viewportGrp(
"ViewportDimensions");
1388 whatsThisObj += viewportGrp;
1396 int iFilterSize = filterName.
size();
1400 PvlKeyword virtualKey(
"Virtual"), physicalKey(
"Physical"), filterNameKey;
1408 virtualKey +=
toString(iGreenBand);
1410 bandGrp += virtualKey;
1415 bandGrp += physicalKey;
1418 if(iRedBand <= iFilterSize) {
1419 filterNameKey += filterName[iRedBand-1];
1422 filterNameKey +=
"None";
1425 if(iGreenBand <= iFilterSize) {
1426 filterNameKey += filterName[iGreenBand-1];
1429 filterNameKey +=
"None";
1432 if(iBlueBand <= iFilterSize) {
1433 filterNameKey += filterName[iBlueBand-1];
1436 filterNameKey +=
"None";
1438 bandGrp += filterNameKey;
1449 if(iFilterSize && iGrayBand <= iFilterSize) {
1450 bandGrp +=
PvlKeyword(
"FilterName", filterName[iGrayBand-1]);
1455 double sl, ss, es, el;
1461 cubeAreaPvl += bandGrp;
1462 whatsThisObj += cubeAreaPvl;
1463 pWhatsThisPvl += whatsThisObj;
1482 pFilterNameKey =bandBinGrp.
findKeyword(
"FilterName") ;
1496 double & pdStartLine,
double & pdEndLine)
1499 if(pdStartSample < 1.0){
1500 pdStartSample = 1.0;
1502 if(pdStartLine < 1.0){
1507 viewportToCube(viewport()->width() - 1, viewport()->height() - 1, pdEndSample, pdEndLine);
1522 double sl, ss, es, el;
1528 int iFilterSize = filterNameKey.
size();
1536 sBandInfo =
"Bands(RGB) Virtual = " +
1537 QString::number(iRedBand) +
", ";
1538 sBandInfo += QString::number(iGreenBand) +
", ";
1539 sBandInfo += QString::number(iBlueBand) +
" ";
1541 sBandInfo +=
"Physical = " +
1547 sBandInfo +=
"<br>FilterName = ";
1548 if(iRedBand <= iFilterSize) {
1549 sBandInfo += QString(filterNameKey[iRedBand-1]);
1552 sBandInfo +=
"None";
1556 if(iGreenBand <= iFilterSize) {
1557 sBandInfo += QString(filterNameKey[iGreenBand-1]);
1560 sBandInfo +=
"None";
1564 if(iBlueBand <= iFilterSize) {
1565 sBandInfo += QString(filterNameKey[iBlueBand-1]);
1568 sBandInfo +=
"None";
1575 sBandInfo =
"Band(Gray) Virtual = " + QString::number(iGrayBand) +
" ";
1579 if(iFilterSize && iGrayBand <= iFilterSize) {
1580 sBandInfo +=
"<br>FilterName = " + QString(filterNameKey[iGrayBand-1]);
1585 "<p><b>Visible Cube Area:</b><blockQuote> \
1586 Samples = " + QString::number(
int(ss + 0.5)) +
"-" +
1587 QString::number(
int(es + 0.5)) +
"<br> \
1588 Lines = " + QString::number(
int(sl + 0.5)) +
"-" +
1589 QString::number(
int(el + 0.5)) +
"<br> " +
1590 sBandInfo +
"</blockQuote></p>";
1593 setWhatsThis(fullWhatsThis);
1594 viewport()->setWhatsThis(fullWhatsThis);
1696 if(o == viewport()) {
1698 case QEvent::Enter: {
1699 viewport()->setMouseTracking(
true);
1704 case QEvent::MouseMove: {
1705 QMouseEvent *m = (QMouseEvent *) e;
1707 emit
mouseMove(m->pos(), (Qt::MouseButton)(m->button() +
1712 case QEvent::Leave: {
1713 viewport()->setMouseTracking(
false);
1718 case QEvent::MouseButtonPress: {
1719 QMouseEvent *m = (QMouseEvent *) e;
1721 (Qt::MouseButton)(m->button() + m->modifiers()));
1725 case QEvent::MouseButtonRelease: {
1726 QMouseEvent *m = (QMouseEvent *) e;
1728 (Qt::MouseButton)(m->button() + m->modifiers()));
1732 case QEvent::MouseButtonDblClick: {
1733 QMouseEvent *m = (QMouseEvent *) e;
1744 return QAbstractScrollArea::eventFilter(o, e);
1760 if(e->key() == Qt::Key_Plus) {
1765 else if(e->key() == Qt::Key_Minus) {
1770 else if(e->key() == Qt::Key_Up) {
1774 else if(e->key() == Qt::Key_Down) {
1778 else if(e->key() == Qt::Key_Left) {
1782 else if(e->key() == Qt::Key_Right) {
1786 else if ((e->key() == Qt::Key_C) &&
1787 QApplication::keyboardModifiers() &
1788 Qt::ControlModifier) {
1794 QClipboard *clipboard = QApplication::clipboard();
1795 clipboard->setText(fileName.absoluteFilePath());
1798 QAbstractScrollArea::keyPressEvent(e);
1810 QPoint g = QCursor::pos();
1811 QPoint v = viewport()->mapFromGlobal(g);
1818 if(v.x() >= viewport()->width()){
1821 if(v.y() >= viewport()->height()){
1835 QPoint g = QCursor::pos();
1836 return viewport()->mapFromGlobal(g);
1848 QPoint g = QCursor::pos();
1850 QPoint v = viewport()->mapFromGlobal(g);
1857 if(v.x() >= viewport()->width()){
1860 if(v.y() >= viewport()->height()){
1876 QPoint v = viewport()->mapToGlobal(g);
1889 viewport()->blockSignals(
true);
1891 verticalScrollBar()->setValue(1);
1892 verticalScrollBar()->setMinimum(1);
1894 verticalScrollBar()->setPageStep(viewport()->height() / 2);
1896 horizontalScrollBar()->setValue(1);
1897 horizontalScrollBar()->setMinimum(1);
1899 horizontalScrollBar()->setPageStep(viewport()->width() / 2);
1901 if(horizontalScrollBar()->value() != x || verticalScrollBar()->value() != y) {
1902 horizontalScrollBar()->setValue(x);
1903 verticalScrollBar()->setValue(y);
1907 QApplication::sendPostedEvents(viewport(), 0);
1908 viewport()->blockSignals(
false);
1948 delete(*p_knownStretches)[oldBand - 1];
1969 viewport()->repaint();
1976 delete(*p_knownStretches)[stretch];
1977 (*p_knownStretches)[stretch] = NULL;
1986 delete(*p_knownStretches)[index];
1989 (*p_knownStretches)[index] =
new Stretch(stretch);
2027 delete(*p_knownStretches)[oldBand - 1];
2050 delete(*p_knownStretches)[oldBand - 1];
2073 delete(*p_knownStretches)[oldBand - 1];
2109 stretch.
Parse(
string);
2121 stretch.
Parse(
string);
2133 stretch.
Parse(
string);
2145 stretch.
Parse(
string);
2205 viewport()->update();
2223 viewport()->update();
2241 viewport()->update();
2259 viewport()->update();
2271 double sampScale = (double) viewport()->width() / (double)
cubeSamples();
2272 double lineScale = (double) viewport()->height() / (double)
cubeLines();
2273 double scale = sampScale < lineScale ? sampScale : lineScale;
2299 double scale = (double) viewport()->height() / (double)
cubeLines();
2314 double ss, sl, es, el;
2315 ss = (double)(rect.left()) - 1.;
2316 sl = (double)(rect.top()) - 1.;
2317 es = (double)(rect.right()) + 1.;
2318 el = (double)(rect.bottom()) + 1.;
2328 if(el >
cube()->lineCount()){
2343 if(ex > viewport()->width()){
2344 ex = viewport()->width();
2346 if(ey > viewport()->height()){
2347 ey = viewport()->height();
2349 QRect vpRect(sx, sy, ex - sx + 1, ey - sy + 1);
2379 QString trackingCubeName = trackingGroup.
findKeyword(
"Filename")[0];
2380 FileName trackingCubeFileName(cubeName.
path() +
"/" + trackingCubeName);
2393 viewport()->setCursor(cursor);
2397 CubeViewport::BandInfo::BandInfo() : band(1), stretch(NULL) {
2400 stretch->SetLis(0.0);
2401 stretch->SetLrs(0.0);
2402 stretch->SetHis(255.0);
2403 stretch->SetHrs(255.0);
2404 stretch->SetMinimum(0.0);
2405 stretch->SetMaximum(255.0);
2425 ASSERT_PTR(stretch);
2432 *stretch = newStretch;
BandInfo p_green
Green band info.
int cubeLines() const
Return the number of lines in the cube.
void SetBasePosition(const int start_sample, const int start_line, const int start_band)
This method is used to set the base position of the shape buffer.
virtual void SetBand(const int band)
Virtual method that sets the band number.
void setCaption()
Change the caption on the viewport title bar.
void scaleChanged()
Emitted when zoom factor changed just before the repaint event.
virtual void keyPressEvent(QKeyEvent *e)
Process arrow keystrokes on cube.
Brick * p_gryBrick
Bricks for every color.
void scrollContentsBy(int dx, int dy)
Scroll the viewport contents by dx/dy screen pixels.
Brick * p_pntBrick
Bricks for every color.
Reads and stores visible DN values.
QImage * p_image
The qimage.
QTimer * p_progressTimer
Activated to update progress bar.
Camera * p_camera
The camera from the cube.
void setAllBandStretches(Stretch stretch)
Sets a stretch for all bands.
bool p_updatingBuffers
Changing RGB and need to not repaint pixmap?
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
virtual QString fileName() const
Returns the opened cube's filename.
int SampleDimension() const
Returns the number of samples in the shape buffer.
const std::vector< double > & getLine(int line)
Retrieves a line from the buffer.
Contains Pvl Groups and Pvl Objects.
void resizedViewport()
Call this when the viewport is resized (not zoomed).
QList< QRect * > * p_pixmapPaintRects
A list of rects that the viewport buffers have requested painted.
A single keyword-value pair.
void cubeContentsChanged(QRect rect)
Calle dhwen the contents of the cube changes.
virtual bool eventFilter(QObject *o, QEvent *e)
Event filter to watch for mouse events on viewport.
bool p_saveEnabled
Has the cube changed?
ViewportBuffer * p_greenBuffer
Viewport Buffer to manage green band.
PvlGroup & group(const QString &group) const
Read a group from the cube into a Label.
double fitScale() const
Determine the scale that causes the full cube to fit in the viewport.
void saveChanges(CubeViewport *)
Emitted when changes should be saved.
void getAllWhatsThisInfo(Pvl &pWhatsThisPvl)
Get All WhatsThis info - viewport, cube, area in PVL format.
void paintPixmapRects()
Goes through the list of requested paints, from the viewport buffer, and paints them.
void read(Blob &blob, const std::vector< PvlKeyword > keywords=std::vector< PvlKeyword >()) const
This method will read data from the specified Blob object.
QVector< Stretch * > * p_knownStretches
Stretches for each previously stretched band.
File name manipulation and expansion.
void center(int x, int y)
Bring the cube pixel under viewport x/y to the center.
void setScale(double scale)
Change the scale of the cube to the given parameter value.
~BandInfo()
Deconstructor.
QPixmap p_pixmap
The qpixmap.
BandInfo p_blue
Blue band info.
void setCursorPosition(int x, int y)
Set the cursor position to x/y in the viewport.
CubeStretch getStretch() const
ViewportBuffer * p_grayBuffer
Viewport Buffer to manage gray band.
virtual int physicalBand(const int &virtualBand) const
This method will return the physical band number given a virtual band number.
Brick * p_redBrick
Bricks for every color.
bool hasGroup(const QString &name) const
Returns a boolean value based on whether the object has the specified group or not.
void mouseLeave()
Emitted when the mouse leaves the viewport.
double grayPixel(int sample, int line)
Gets the gray pixel.
BandInfo p_red
Red band info.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
Container for cube-like labels.
void forgetStretches()
Resets all remembered stretches.
ViewportBuffer * p_blueBuffer
Viewport Buffer to manage blue band.
int Pairs() const
Returns the number of stretch pairs.
Stores stretch information for a cube.
Isis::Camera * Camera() const
Return the camera associated with the ground map (NULL implies none)
void setBand(int band)
Sets the band to read from, the buffer will be re-read if the band changes.
Brick * p_bluBrick
Bricks for every color.
void contentsToCube(int x, int y, double &sample, double &line) const
Turns contents to a cube.
CubeStretch greenStretch() const
Return the green band stretch.
int getBand()
Return the band associated with this viewport buffer.
void moveCursor(int x, int y)
Move the cursor by x,y if possible.
void discardChanges(CubeViewport *)
Emitted when changes should be discarded.
virtual void viewRGB(int redBand, int greenBand, int blueBand)
View cube as color.
void cubeToContents(double sample, double line, int &x, int &y) const
Turns a cube into contents.
Buffer for containing a three dimensional section of an image.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
QString p_whatsThisText
The text for What's this.
void getBandFilterName(PvlKeyword &pFilterNameKey)
Get Band Filter name from the Isis cube label.
void updateScrollBars(int x, int y)
Update the scroll bar.
Encapsulation of Cube I/O with Change Notifications.
int FindCubeId(const Cube *) const
Given a Cube pointer, return the cube ID associated with it.
CubeStretch redStretch() const
Return the red band stretch.
void AddChangeListener()
You must call this method after connecting to the BrickChanged signal, otherwise you are not guarante...
double fitScaleWidth() const
Determine the scale of cube in the width to fit in the viewport.
void stretchKnownGlobal()
List<Tool *> p This stretches to the global stretch.
bool p_thisOwnsCubeData
if true then this owns the CubeDataThread, and should thus delete it
void mouseEnter()
Emitted when the mouse enters the viewport.
void paintPixmap()
Paint the whole pixmap.
bool p_paintPixmap
Paint the pixmap?
bool enabled()
Returns whether the buffer is enabled (reading data) or not.
void mouseDoubleClick(QPoint)
Emitted when double click happens.
CubeStretch blueStretch() const
Return the blue band stretch.
void cubeToViewport(double sample, double line, int &x, int &y) const
Turns a cube into a viewport.
void mouseButtonPress(QPoint, Qt::MouseButton)
Emitted when mouse button pressed.
double currentProgress()
Returns the viewport buffer's loading progress.
int AddCube(const FileName &fileName, bool mustOpenReadWrite=false)
This method is designed to be callable from any thread before data is requested, though no known side...
void setStretch(const Stretch &newStretch)
const BandInfo & operator=(BandInfo other)
The BandInfo for the Cube.
Contains multiple PvlContainers.
virtual void resizeEvent(QResizeEvent *e)
The viewport is being resized.
void enableProgress()
This restarts the progress bar.
void screenPixelsChanged()
Emitted when cube pixels that should be on the screen change.
CubeStretch grayStretch() const
Return the gray band stretch.
void CopyPairs(const Stretch &other)
Copies the stretch pairs from another Stretch object, but maintains special pixel values.
bool hasGroup(const QString &group) const
Return if the cube has a specified group in the labels.
void Parse(const QString &pairs)
Parses a string of the form "i1:o1 i2:o2...iN:oN" where each i:o represents an input:output pair.
bool p_color
Is the viewport in color?
double redPixel(int sample, int line)
Gets the red pixel.
double greenPixel(int sample, int line)
Gets the green pixel.
bool confirmClose()
This method should be called during a close event that would cause this viewport to close.
Projection * p_projection
The projection from the cube.
int p_comboCount
Number of elements in band bin combo box.
void windowTitleChanged()
Emitted when window title changes.
int LineDimension() const
Returns the number of lines in the shape buffer.
Cube * trackingCube() const
void mouseMove(QPoint)
Emitted when the mouse moves.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
void getCubeArea(double &pdStartSample, double &pdEndSample, double &pdStartLine, double &pdEndLine)
Get Cube area corresponding to the viewport's dimension.
QSize sizeHint() const
Make viewports show up as 512 by 512.
void bufferUpdated(QRect rect)
This method is called by ViewportBuffer upon successful completion of all operations and gives the ap...
bool isOpen() const
Test if a cube file has been opened/created.
IO Handler for Isis Cubes.
void doneWithData(int, const Isis::Brick *)
Emitted when a brick is no longer needed, should only be sent to cube data thread.
int cubeSamples() const
Return the number of samples in the cube.
virtual int bandCount() const
Returns the number of virtual bands for the cube.
CubeDataThread * p_cubeData
Does all the cube I/O.
QRect bufferXYRect()
Returns a rect, in screen pixels, of the area this buffer covers.
QPoint cursorPosition() const
Return the cursor position in the viewport.
int p_cubeId
Cube ID given from cube data thread for I/O.
Isis::Projection * Projection() const
Return the projection associated with the ground map (NULL implies none)
CubeStretch * stretch
The Stretch.
void scrollBy(int dx, int dy)
Move the scrollbars by dx/dy screen pixels.
BandInfo p_gray
Gray band info.
void SetNull(const double value)
Sets the mapping for NULL pixels.
double bluePixel(int sample, int line)
Gets the blue pixel.
@ Programmer
This error is for when a programmer made an API call that was illegal.
void RemoveChangeListener()
You must call this method after disconnecting from the BrickChanged signal, otherwise bricks cannot b...
Namespace for the standard library.
void changeCursor(QCursor cursor)
Allows users to change the cursor type on the viewport.
void stretchGreen(const QString &string)
Apply stretch pairs to green bands.
void addStretchAction()
When all current operations finish the cube viewport will be asked to do a stretch if you call this.
PixelType pixelType() const
ViewportBuffer * p_redBuffer
Viewport Buffer to manage red band.
void scaleChanged()
Call this when zoomed, re-reads visible area.
QVector< Stretch * > * p_globalStretches
Global stretches for each stretched band.
void setTrackingCube()
Finds the Tracking group from p_cube and stores the tracking cube name so that we can grab it in Adva...
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
void enable(bool enabled)
This turns on or off reading from the cube.
virtual ~CubeViewport()
Deconstructor for the Cubeviewport.
void cubeChanged(bool changed)
This method is called when the cube has changed or changes have been finalized.
int p_comboIndex
Current element chosen from combo box.
QString p_viewportWhatsThisText
The text for the viewport's what's this.
void pan(int deltaX, int deltaY)
Call this when the viewport is panned.
void setCube(Cube *cube)
This method sets the viewports cube.
void mouseButtonRelease(QPoint, Qt::MouseButton)
Emitted when mouse button released.
int size() const
Returns the number of values stored in this keyword.
virtual void viewGray(int band)
View cube as gray.
double fitScaleHeight() const
Determine the scale of cube in heighth to fit in the viewport.
Brick * p_grnBrick
Bricks for every color.
void onProgressTimer()
This updates the progress bar visually.
void viewportToCube(int x, int y, double &sample, double &line) const
Turns a viewport into a cube.
virtual void paintEvent(QPaintEvent *e)
Repaint the viewport.
PvlKeyword & findKeyword(const QString &name)
Find a keyword with a specified name.
This is free and unencumbered software released into the public domain.
void shiftPixmap(int dx, int dy)
Shifts the pixels on the pixmap without reading new data.
void progressChanged(int)
Emitted with current progress (0 to 100) when working.
Cube * p_cube
The cube associated with the viewport.
int Sample(const int index=0) const
Returns the sample position associated with a shape buffer index.
void showEvent(QShowEvent *)
This method is called to initially show the viewport.
void fillBuffer(QRect rect)
This method will convert the rect to sample/line positions and read from the cube into the buffer.
UniversalGroundMap * p_groundMap
The universal ground map from the cube.
bool HasProjection()
Checks to see if the camera object has a projection.
bool cursorInside() const
Is cursor inside viewport.
bool p_cubeShown
Is the cube visible?
Projection * projection()
int Line(const int index=0) const
Returns the line position associated with a shape buffer index.
virtual void cubeDataChanged(int cubeId, const Isis::Brick *)
This method updates the internal viewport buffer based on changes in cube DN values.
int cubeBands() const
Return the number of bands in the cube.
void stretchGray(const QString &string)
Apply stretch pairs to gray band.
QString path() const
Returns the path of the file name.
void stretchBlue(const QString &string)
Apply stretch pairs to blue bands.
This is free and unencumbered software released into the public domain.
QColor p_bgColor
The color to paint the background of the viewport.
void progressComplete()
Emitted when the current progress is complete (100)
QString p_cubeWhatsThisText
The text for the cube's What's this.
double p_scale
The scale number.
void updateWhatsThis()
Update the What's This text.
bool working()
This tests if queued actions exist in the viewport buffer.
double Map(const double value) const
Maps an input value to an output value based on the stretch pairs and/or special pixel mappings.
void stretchRed(const QString &string)
Apply stretch pairs to red bands.