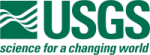 |
Isis 3 Programmer Reference
|
24 #include "Constants.h"
25 #include "CameraDetectorMap.h"
26 #include "CameraFocalPlaneMap.h"
27 #include "CameraDistortionMap.h"
28 #include "CameraGroundMap.h"
29 #include "CameraSkyMap.h"
31 #include "IException.h"
35 #include "Longitude.h"
36 #include "NaifStatus.h"
37 #include "Projection.h"
38 #include "ProjectionFactory.h"
39 #include "RingPlaneProjection.h"
40 #include "ShapeModel.h"
41 #include "SpecialPixel.h"
42 #include "SurfacePoint.h"
44 #include "TProjection.h"
57 PvlObject::FindOptions::Traverse).findKeyword(
"InstrumentId")[0];
75 if (lab.
findObject(
"IsisCube").hasGroup(
"Mapping")) {
169 bool success =
false;
243 bool success =
false;
298 if (shape->
name() !=
"Plane") {
357 if (tproj->
SetWorld(sample, line)) {
405 if (shape->
name() !=
"Plane") {
433 if (!surfacePt.Valid()) {
543 const double radius) {
614 double a = sB[0] - pB[0];
615 double b = sB[1] - pB[1];
616 double c = sB[2] - pB[2];
617 double dist = sqrt(a * a + b * b + c * c) * 1000.0;
675 return (lineRes + sampRes) / 2.0;
690 return (lineRes + sampRes) / 2.0;
743 if (
target()->shape()->name() ==
"Plane") {
744 IString msg =
"Images with plane targets should use Camera method RingRangeResolution ";
745 msg +=
"instead of GroundRangeResolution";
754 double originalSample =
Sample();
755 double originalLine =
Line();
756 int originalBand =
Band();
773 for (
int band = 1; band <= eband; band++) {
777 for (
int line = 1; line <=
p_lines + 1; line++) {
781 for (samp = 1; samp <=
p_samples + 1; samp++) {
783 if (
SetImage((
double)samp - 0.5, (
double)line - 0.5)) {
791 if (lon > 180.0) lon -= 360.0;
802 if (obliqueres > 0.0) {
807 if ((line != 1) && (line !=
p_lines + 1))
break;
812 if (line == 1)
continue;
813 if (line ==
p_lines + 1)
continue;
817 for (samp =
p_samples + 1; samp >= 1; samp--) {
818 if (
SetImage((
double)samp - 0.5, (
double)line - 0.5)) {
826 if (lon > 180.0) lon -= 360.0;
838 if (obliqueres > 0.0) {
874 if (obliqueres > 0.0) {
956 SetImage(originalSample, originalLine);
963 string message =
"Camera missed planet or SPICE data off.";
991 double originalSample =
Sample();
992 double originalLine =
Line();
993 int originalBand =
Band();
1010 for (
int band = 1; band <= eband; band++) {
1014 for (
int line = 1; line <=
p_lines + 1; line++) {
1019 for (samp = 1; samp <=
p_samples + 1; samp++) {
1021 if (
SetImage((
double)samp - 0.5, (
double)line - 0.5)) {
1029 if (azimuth > 180.0) azimuth -= 360.0;
1038 if ((line != 1) && (line !=
p_lines + 1))
break;
1042 if (line == 1)
continue;
1043 if (line ==
p_lines + 1)
continue;
1047 for(samp =
p_samples + 1; samp >= 1; samp--) {
1048 if (
SetImage((
double)samp - 0.5, (
double)line - 0.5)) {
1056 if (azimuth > 180.0) azimuth -= 360.0;
1142 SetImage(originalSample, originalLine);
1151 string message =
"RingPlane ShapeModel - Camera missed plane or SPICE data off.";
1166 double minlat, minlon, maxlat, maxlon;
1167 return GroundRange(minlat, maxlat, minlon, maxlon, pvl);
1183 double &minlon,
double &maxlon,
1207 QString latType = (QString) map[
"LatitudeType"];
1208 if (latType.toUpper() ==
"PLANETOGRAPHIC") {
1209 if (abs(minlat) < 90.0) {
1210 minlat *=
PI / 180.0;
1211 minlat = atan(tan(minlat) * (a / b) * (a / b));
1212 minlat *= 180.0 /
PI;
1215 if(abs(maxlat) < 90.0) {
1216 maxlat *=
PI / 180.0;
1217 maxlat = atan(tan(maxlat) * (a / b) * (a / b));
1218 maxlat *= 180.0 /
PI;
1226 bool domain360 =
true;
1228 QString lonDomain = (QString) map[
"LongitudeDomain"];
1229 if(lonDomain.toUpper() ==
"180") {
1238 QString lonDirection = (QString) map[
"LongitudeDirection"];
1239 if(lonDirection.toUpper() ==
"POSITIVEWEST") {
1240 double swap = minlon;
1248 while(minlon < 0.0) {
1252 while(minlon > 360.0) {
1258 while(minlon < -180.0) {
1262 while(minlon > 180.0) {
1269 if((maxlon - minlon) > 359.0)
return true;
1287 double &minRingLongitude,
double &maxRingLongitude,
Pvl &pvl) {
1301 bool domain360 =
true;
1303 QString ringLongitudeDomain = (QString) map[
"RingLongitudeDomain"];
1304 if (ringLongitudeDomain ==
"180") {
1312 if (map.
hasKeyword(
"RingLongitudeDirection")) {
1313 QString ringLongitudeDirection = (QString) map[
"RingLongitudeDirection"];
1314 if (ringLongitudeDirection.toUpper() ==
"Clockwise") {
1315 double swap = minRingLongitude;
1316 minRingLongitude = -maxRingLongitude;
1317 maxRingLongitude = -swap;
1323 while (minRingLongitude < 0.0) {
1324 minRingLongitude += 360.0;
1325 maxRingLongitude += 360.0;
1327 while (minRingLongitude > 360.0) {
1328 minRingLongitude -= 360.0;
1329 maxRingLongitude -= 360.0;
1333 while (minRingLongitude < -180.0) {
1334 minRingLongitude += 360.0;
1335 maxRingLongitude += 360.0;
1337 while (minRingLongitude > 180.0) {
1338 minRingLongitude -= 360.0;
1339 maxRingLongitude -= 360.0;
1344 if ((maxRingLongitude - minRingLongitude) > 359.0) {
1364 map +=
PvlKeyword(
"LatitudeType",
"Planetocentric");
1365 map +=
PvlKeyword(
"LongitudeDirection",
"PositiveEast");
1375 map +=
PvlKeyword(
"ProjectionName",
"Sinusoidal");
1386 if (
target()->shape()->name() !=
"Plane") {
1388 IString msg =
"A ring plane projection has been requested on an image whose shape is not a ring plane. ";
1389 msg +=
"Rerun spiceinit with shape=RINGPLANE. ";
1396 map +=
PvlKeyword(
"RingLongitudeDirection",
"CounterClockwise");
1397 map +=
PvlKeyword(
"RingLongitudeDomain",
"360");
1406 map +=
PvlKeyword(
"ProjectionName",
"Planar");
1413 QString key =
"INS" +
toString(code) +
"_FOCAL_LENGTH";
1420 QString key =
"INS" +
toString(code) +
"_PIXEL_PITCH";
1463 else if (
target()->shape()->hasIntersection()) {
1493 normal[0] = normal[1] = normal[2] = 0.0;
1502 if (!shapeModel->
isDEM()) {
1509 double origin[3] = {0, 0, 0};
1510 unusedNeighborPoints.fill(origin);
1511 shapeModel->calculateLocalNormal(unusedNeighborPoints);
1519 double line =
Line();
1523 surroundingPoints.append(qMakePair(samp, std::nexttoward(line - 0.5, line)));
1524 surroundingPoints.append(qMakePair(samp, std::nexttoward(line + 0.5, line)));
1525 surroundingPoints.append(qMakePair(std::nexttoward(samp - 0.5, samp), line));
1526 surroundingPoints.append(qMakePair(std::nexttoward(samp + 0.5, samp), line));
1529 double originalSample = samp;
1530 double originalLine = line;
1533 for (
int i = 0; i < cornerNeighborPoints.size(); i++) {
1534 cornerNeighborPoints[i] =
new double[3];
1542 for (
int i = 0; i < cornerNeighborPoints.size(); i++) {
1544 if (!(
SetImage(surroundingPoints[i].first, surroundingPoints[i].second))) {
1545 surroundingPoints[i].first = samp;
1546 surroundingPoints[i].second = line;
1549 if (!(
SetImage(surroundingPoints[i].first, surroundingPoints[i].second))) {
1551 normal[0] = normal[1] = normal[2] = 0.0;
1555 SetImage(originalSample, originalLine);
1562 for (
int i = 0; i < cornerNeighborPoints.size(); i++) {
1563 delete [] cornerNeighborPoints[i];
1580 if ((surroundingPoints[0].first == surroundingPoints[1].first &&
1581 surroundingPoints[0].second == surroundingPoints[1].second) ||
1582 (surroundingPoints[2].first == surroundingPoints[3].first &&
1583 surroundingPoints[2].second == surroundingPoints[3].second)) {
1585 normal[0] = normal[1] = normal[2] = 0.0;
1589 SetImage(originalSample, originalLine);
1596 for (
int i = 0; i < cornerNeighborPoints.size(); i++)
1597 delete [] cornerNeighborPoints[i];
1603 SetImage(originalSample, originalLine);
1604 shapeModel->calculateLocalNormal(cornerNeighborPoints);
1607 for (
int i = 0; i < cornerNeighborPoints.size(); i++) {
1608 delete [] cornerNeighborPoints[i];
1625 std::vector<double> localNormal(3);
1626 localNormal = shapeModel->
normal();
1627 memcpy(normal, (
double *) &localNormal[0],
sizeof(
double) * 3);
1643 Angle & emission,
bool &success) {
1652 unorm_c(normal,normal,&mag);
1659 SpiceDouble surfSpaceVect[3], unitizedSurfSpaceVect[3], dist;
1669 vsub_c((SpiceDouble *) &sB[0], pB, surfSpaceVect);
1670 unorm_c(surfSpaceVect, unitizedSurfSpaceVect, &dist);
1673 SpiceDouble surfaceSunVect[3];
1674 vsub_c(
m_uB, pB, surfaceSunVect);
1675 SpiceDouble unitizedSurfSunVect[3];
1676 unorm_c(surfaceSunVect, unitizedSurfSunVect, &dist);
1680 phase =
Angle(vsep_c(unitizedSurfSpaceVect, unitizedSurfSunVect),
1685 emission =
Angle(vsep_c(unitizedSurfSpaceVect, normal),
1690 incidence =
Angle(vsep_c(unitizedSurfSunVect, normal),
1708 double &mindec,
double &maxdec) {
1712 double originalSample =
Sample();
1713 double originalLine =
Line();
1714 int originalBand =
Band();
1731 for (
int band = 1; band <= eband; band++) {
1734 for (
int line = 1; line <=
p_lines; line++) {
1737 for (samp = 1; samp <=
p_samples; samp++) {
1738 SetImage((
double)samp, (
double)line);
1746 if (ra > 180.0) ra -= 360.0;
1750 if ((line != 1) && (line !=
p_lines))
break;
1755 for (samp =
p_samples; samp >= 1; samp--) {
1756 SetImage((
double)samp, (
double)line);
1764 if (ra > 180.0) ra -= 360.0;
1834 SetImage(originalSample, originalLine);
1852 double originalSample =
Sample();
1853 double originalLine =
Line();
1854 int originalBand =
Band();
1864 double dist = (ra1 - ra2) * (ra1 - ra2) + (dec1 - dec2) * (dec1 - dec2);
1866 double lineRes = dist / (
p_lines - 1);
1872 dist = (ra1 - ra2) * (ra1 - ra2) + (dec1 - dec2) * (dec1 - dec2);
1874 double sampRes = dist / (
p_samples - 1);
1879 SetImage(originalSample, originalLine);
1885 return (sampRes < lineRes) ? sampRes : lineRes;
1894 if (
target()->shape()->name() ==
"Plane") {
1895 QString msg =
"North Azimuth is not available for plane target shapes.";
1908 if (azimuth > 360.0) azimuth = azimuth - 360.0;
2051 SpiceDouble azimuthOrigin[3];
2054 if (!originRadius.
isValid()) {
2061 SpiceDouble pointOfInterestFromBodyCenter[3];
2063 lat *
PI / 180.0, pointOfInterestFromBodyCenter);
2071 SpiceDouble pointOfInterest[3];
2072 vsub_c(pointOfInterestFromBodyCenter, azimuthOrigin, pointOfInterest);
2078 SpiceDouble pointOfInterestProj[3];
2079 vperp_c(pointOfInterest, azimuthOrigin, pointOfInterestProj);
2082 SpiceDouble pointOfInterestProjUnit[3];
2083 vhat_c(pointOfInterestProj, pointOfInterestProjUnit);
2089 SpiceDouble pointOfInterestProjUnitScaled[3];
2090 vscl_c(scale, pointOfInterestProjUnit, pointOfInterestProjUnitScaled);
2095 SpiceDouble adjustedPointOfInterestFromBodyCenter[3];
2096 vadd_c(azimuthOrigin, pointOfInterestProjUnitScaled, adjustedPointOfInterestFromBodyCenter);
2099 double azimuthOriginSample =
Sample();
2100 double azimuthOriginLine =
Line();
2103 double adjustedPointOfInterestRad, adjustedPointOfInterestLon, adjustedPointOfInterestLat;
2104 reclat_c(adjustedPointOfInterestFromBodyCenter,
2105 &adjustedPointOfInterestRad,
2106 &adjustedPointOfInterestLon,
2107 &adjustedPointOfInterestLat);
2108 adjustedPointOfInterestLat = adjustedPointOfInterestLat * 180.0 /
PI;
2109 adjustedPointOfInterestLon = adjustedPointOfInterestLon * 180.0 /
PI;
2110 if (adjustedPointOfInterestLon < 0) adjustedPointOfInterestLon += 360.0;
2116 adjustedPointOfInterestLon,
2121 SetImage(azimuthOriginSample, azimuthOriginLine);
2125 double adjustedPointOfInterestSample =
Sample();
2126 double adjustedPointOfInterestLine =
Line();
2154 double deltaSample = adjustedPointOfInterestSample - azimuthOriginSample;
2155 double deltaLine = adjustedPointOfInterestLine - azimuthOriginLine;
2164 double azimuth = 0.0;
2165 if (deltaSample != 0.0 || deltaLine != 0.0) {
2166 azimuth = atan2(deltaLine, deltaSample);
2167 azimuth *= 180.0 /
PI;
2171 if (azimuth < 0.0) azimuth += 360.0;
2172 if (azimuth > 360.0) azimuth -= 360.0;
2180 SetImage(azimuthOriginSample, azimuthOriginLine);
2199 double coord[3], spCoord[3];
2204 double a = vsep_c(coord, spCoord) * 180.0 /
PI;
2208 double c = 180.0 - (a + b);
2238 double slat,
double slon) {
2242 a = (90.0 - slat) *
PI / 180.0;
2243 b = (90.0 - glat) *
PI / 180.0;
2246 a = (90.0 + slat) *
PI / 180.0;
2247 b = (90.0 + glat) *
PI / 180.0;
2250 double cslon = slon;
2251 double cglon = glon;
2252 if (cslon > cglon) {
2253 if ((cslon-cglon) > 180.0) {
2254 while ((cslon-cglon) > 180.0) cslon = cslon - 360.0;
2257 if (cglon > cslon) {
2258 if ((cglon-cslon) > 180.0) {
2259 while ((cglon-cslon) > 180.0) cglon = cglon - 360.0;
2266 if (cslon > cglon) {
2269 else if (cslon < cglon) {
2276 else if (slat < glat) {
2277 if (cslon > cglon) {
2280 else if (cslon < cglon) {
2288 if (cslon > cglon) {
2291 else if (cslon < cglon) {
2299 double C = (cglon - cslon) *
PI / 180.0;
2301 double c = acos(cos(a)*cos(b) + sin(a)*sin(b)*cos(C));
2302 double azimuth = 0.0;
2303 if (sin(b) == 0.0 || sin(c) == 0.0) {
2306 double intermediate = (cos(a) - cos(b)*cos(c))/(sin(b)*sin(c));
2307 if (intermediate < -1.0) {
2308 intermediate = -1.0;
2310 else if (intermediate > 1.0) {
2313 double A = acos(intermediate) * 180.0 /
PI;
2316 if (quad == 1 || quad == 4) {
2319 else if (quad == 2 || quad == 3) {
2320 azimuth = 360.0 - A;
2324 if (quad == 1 || quad == 4) {
2325 azimuth = 180.0 - A;
2327 else if (quad == 2 || quad == 3) {
2328 azimuth = 180.0 + A;
2427 int cacheSize =
CacheSize(ephemerisTimes.first, ephemerisTimes.second);
2435 double altitudeMeters;
2437 altitudeMeters = 1.0;
2450 setTime(ephemerisTimes.first);
2480 pair<double,double> ephemerisTimes;
2481 double startTime = -DBL_MAX;
2482 double endTime = -DBL_MAX;
2484 for (
int band = 1; band <=
Bands(); band++) {
2487 double etStart =
time().
Et();
2490 double etEnd =
time().
Et();
2492 startTime = min(etStart, etEnd);
2493 endTime = max(etStart, etEnd);
2495 startTime = min(startTime, min(etStart, etEnd));
2496 endTime = max(endTime, max(etStart, etEnd));
2498 if (startTime == -DBL_MAX || endTime == -DBL_MAX) {
2499 string msg =
"Unable to find time range for the spice kernels";
2502 ephemerisTimes.first = startTime;
2503 ephemerisTimes.second = endTime;
2504 return ephemerisTimes;
2531 if (startTime == endTime) {
2559 if (startSize == 2 && endSize == 2) {
2565 if (endSize > startSize) {
2566 IString message =
"Camera::SetGeometricTilingHint End size must be smaller than the start size";
2570 if (startSize < 4) {
2571 IString message =
"Camera::SetGeometricTilingHint Start size must be at least 4";
2575 bool foundEnd =
false;
2576 while (powerOf2 > 0 && startSize != powerOf2) {
2577 if (powerOf2 == endSize) foundEnd =
true;
2584 IString message =
"Camera::SetGeometricTilingHint Start size must be a power of 2";
2589 IString message =
"Camera::SetGeometricTilingHint End size must be a power of 2 less than the start size, but greater than 2";
3038 double orgLine =
Line();
3039 double orgSample =
Sample();
3044 double y =
Line() - orgLine;
3045 double x =
Sample() - orgSample;
3046 double celestialNorthClockAngle = atan2(-y, x) * 180.0 /
Isis::PI;
3047 celestialNorthClockAngle = 90.0 - celestialNorthClockAngle;
3049 if (celestialNorthClockAngle < 0.0) {
3050 celestialNorthClockAngle += 360.0;
3054 return celestialNorthClockAngle;
double kilometers() const
Get the distance in kilometers.
void radii(Distance r[3]) const
Returns the radii of the body in km.
virtual void SetBand(const int band)
Virtual method that sets the band number.
int CacheSize(double startTime, double endTime)
This method calculates the spice cache size.
@ Degrees
Degrees are generally considered more human readable, 0-360 is one circle, however most math does not...
virtual ~Camera()
Destroys the Camera Object.
void IgnoreProjection(bool ignore)
Set whether or not the camera should ignore the Projection.
bool SetRightAscensionDeclination(const double ra, const double dec)
Sets the right ascension declination.
const double HALFPI
The mathematical constant PI/2.
Projection * p_projection
A pointer to the Projection.
virtual double exposureDuration() const
Return the exposure duration for the pixel that the camera is set to.
double p_minRingRadius
The minimum ring radius.
double FocalPlaneX() const
double p_mindec
The minimum declination.
iTime time() const
Returns the ephemeris time in seconds which was used to obtain the spacecraft and sun positions.
double p_minlon
The minimum longitude.
void SetGeometricTilingHint(int startSize=128, int endSize=8)
This method sets the best geometric tiling size for projecting from this camera model.
bool SetImageSkyMapProjection(const double sample, const double line, ShapeModel *shape)
Sets the sample/line values of the image to get the lat/lon values for a Skymap Projected image.
virtual bool SetSky(const double ra, const double dec)
Compute undistorted focal plane coordinate from ra/dec.
CameraSkyMap * p_skyMap
A pointer to the SkyMap.
double p_maxdec
The maximum declination.
ShapeModel * shape() const
Return the shape.
PvlGroupIterator findGroup(const QString &name, PvlGroupIterator beg, PvlGroupIterator end)
Find a group with the specified name, within these indexes.
virtual void subSolarPoint(double &lat, double &lon)
Returns the sub-solar latitude/longitude in universal coordinates (0-360 positive east,...
int Band() const
Returns the current band.
virtual void clearSurfacePoint()
Clears or resets the current surface point.
double FocalPlaneY() const
virtual void subSpacecraftPoint(double &lat, double &lon)
Returns the sub-spacecraft latitude/longitude in universal coordinates (0-360 positive east,...
double UniversalRingLongitude()
This returns a universal ring longitude (clockwise in 0 to 360 domain).
virtual SpicePosition * instrumentPosition() const
Accessor method for the instrument position.
Convert between parent image coordinates and detector coordinates.
const double PI
The mathematical constant PI.
double SpacecraftAltitude()
Returns the distance from the spacecraft to the subspacecraft point in km.
int ParentLines() const
Returns the number of lines in the parent alphacube.
SpiceInt naifBodyCode() const
This returns the NAIF body code of the target indicated in the labels.
virtual double ObliquePixelResolution()
Returns the oblique pixel resolution at the current position in meters/pixel.
CameraSkyMap * SkyMap()
Returns a pointer to the CameraSkyMap object.
A single keyword-value pair.
virtual int SpkTargetId() const
Provides target code for instruments SPK NAIF kernel.
This is free and unencumbered software released into the public domain.
double p_childLine
Line value for child.
This class is designed to encapsulate the concept of a Latitude.
void SetSkyMap(CameraSkyMap *map)
Sets the Sky Map.
SpiceInt naifIkCode() const
This returns the NAIF IK code to use when reading from instrument kernels.
virtual double UniversalLatitude()
This returns a universal latitude (planetocentric).
bool HasSurfaceIntersection() const
Returns if the last call to either SetLookDirection or SetUniversalGround had a valid intersection wi...
virtual bool SetImage(const double sample, const double line)
Sets the sample/line values of the image to get the lat/lon values.
double p_maxra180
The maximum right ascension in the 180 domain.
int p_lines
The number of lines in the image.
double p_minRingLongitude180
The minimum ring longitude in the 180 domain.
bool IntersectsLongitudeDomain(Pvl &pvl)
Checks whether the ground range intersects the longitude domain or not.
const double DEG2RAD
Multiplier for converting from degrees to radians.
void SetDetectorMap(CameraDetectorMap *map)
Sets the Detector Map.
double UndistortedFocalPlaneX() const
Gets the x-value in the undistorted focal plane coordinate system.
double DetectorSample() const
Return detector sample.
void SetGroundMap(CameraGroundMap *map)
Sets the Ground Map.
virtual double Sample() const
Returns the current sample number.
@ Unknown
A type of error that cannot be classified as any of the other error types.
void setHasIntersection(bool b)
Sets the flag to indicate whether this ShapeModel has an intersection.
double p_maxlon180
The maximum longitude in the 180 domain.
QString m_instrumentNameLong
Full instrument name.
virtual double SampleScaleFactor() const
Return scaling factor for computing sample resolution.
virtual bool SetUndistortedFocalPlane(double ux, double uy)
Compute distorted focal plane x/y.
virtual bool SetParent(const double sample, const double line)
Compute detector position from a parent image coordinate.
virtual double ObliqueDetectorResolution()
This method returns the Oblique Detector Resolution if the Look Vector intersects the target and if t...
double AlphaLine(double betaLine)
Returns an alpha line given a beta line.
double p_maxRingLongitude
The maximum ring longitude (azimuth)
virtual double Declination()
Returns the declination angle (sky latitude).
int p_referenceBand
The reference band.
double FocalPlaneX() const
Gets the x-value in the focal plane coordinate system.
virtual double ObliqueSampleResolution()
Returns the oblique sample resolution at the current position in m.
virtual double resolution()
Returns the resolution of the camera.
void GroundRangeResolution()
Computes the ground range and min/max resolution.
virtual double Longitude() const
This returns a longitude with correct longitude direction and domain as specified in the label object...
double DetectorLine() const
QString name() const
Gets the shape name.
ProjectionType projectionType() const
Returns an enum value for the projection type.
QString instrumentNameLong() const
This method returns the full instrument name.
double FocalPlaneY() const
Gets the y-value in the focal plane coordinate system.
bool hasKeyword(const QString &name) const
Check to see if a keyword exists.
bool RaDecRange(double &minra, double &maxra, double &mindec, double &maxdec)
Computes the RaDec range.
Container for cube-like labels.
CameraDistortionMap * DistortionMap()
Returns a pointer to the CameraDistortionMap object.
double p_minlat
The minimum latitude.
double AlphaSample(double betaSample)
Returns an alpha sample given a beta sample.
Latitude GetLatitude() const
Return the body-fixed latitude for the surface point.
double UndistortedFocalPlaneZ() const
Gets the z-value in the undistorted focal plane coordinate system.
double p_maxobliqueres
The maximum oblique resolution.
virtual void createCache(iTime startTime, iTime endTime, const int size, double tol)
This method creates an internal cache of spacecraft and sun positions over a specified time range.
bool HasReferenceBand() const
Checks to see if the Camera object has a reference band.
virtual bool SetUniversalGround(const double coord1, const double coord2)
This method is used to set the lat/lon or radius/azimuth (i.e.
static void CheckErrors(bool resetNaif=true)
This method looks for any naif errors that might have occurred.
virtual bool SetGround(Latitude latitude, Longitude longitude)
Sets the lat/lon values to get the sample/line values.
SurfacePoint GetSurfacePoint() const
Returns the surface point (most efficient accessor).
int p_bands
The number of bands in the image.
void basicRingMapping(Pvl &map)
Writes the basic mapping group for ring plane to the specified Pvl.
double DetectorSample() const
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
virtual double RightAscension()
Returns the right ascension angle (sky longitude).
bool RawFocalPlanetoImage()
Computes the image coordinate for the current universal ground point.
Distance measurement, usually in meters.
double BetaLine(double alphaLine)
Returns a beta line given an alpha line.
This class is designed to encapsulate the concept of a Longitude.
QString m_instrumentId
The InstrumentId as it appears on the cube.
Convert between undistorted focal plane and ground coordinates.
virtual Target * target() const
Returns a pointer to the target object.
double FocalPlaneY() const
double p_maxres
The maximum resolution.
std::pair< double, double > StartEndEphemerisTimes()
Calculates the start and end ephemeris times.
std::vector< Distance > radii() const
Returns the radii of the body in km.
@ Traverse
Search child objects.
double FocalPlaneX() const
@ Kilometers
The distance is being specified in kilometers.
double p_focalLength
The focal length, in units of millimeters.
double SpacecraftAzimuth()
Return the Spacecraft Azimuth.
virtual double exposureDuration(const double sample, const double line, const int band) const
This virtual method is for returning the exposure duration of a given pixel.
double p_minRingLongitude
The minimum ring longitude (azimuth)
int ParentSamples() const
Returns the number of samples in the parent alphacube.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
double OffNadirAngle()
Return the off nadir angle in degrees.
virtual double WorldY() const
This returns the world Y coordinate provided SetGround, SetCoordinate, SetUniversalGround,...
void ringRangeResolution()
Analogous to above GroundRangeResolution method.
bool p_ignoreProjection
Whether or no to ignore the Projection.
@ Meters
The distance is being specified in meters.
double Et() const
Returns the ephemeris time (TDB) representation of the time as a double.
bool InCube()
This returns true if the current Sample() or Line() value is outside of the cube (meaning the point m...
Base class for Map Projections of plane shapes.
virtual bool isDEM() const =0
Indicates whether this shape model is from a DEM.
void SetPixelPitch()
Reads the Pixel Pitch from the instrument kernel.
Contains multiple PvlContainers.
virtual double UniversalLongitude() const
Returns the positive east, 0-360 domain longitude, in degrees, at the surface intersection point in t...
CameraDetectorMap * p_detectorMap
A pointer to the DetectorMap.
CameraFocalPlaneMap * p_focalPlaneMap
A pointer to the FocalPlaneMap.
static double GroundAzimuth(double glat, double glon, double slat, double slon)
Computes and returns the ground azimuth between the ground point and another point of interest,...
double ComputeAzimuth(const double lat, const double lon)
Computes the image azimuth value from your current position (origin) to a point of interest specified...
virtual bool SetFocalPlane(const double dx, const double dy)
Compute detector position (sample,line) from focal plane coordinates.
SpiceDouble m_uB[3]
This contains the sun position (u) in the bodyfixed reference frame (B).
double LowestObliqueImageResolution()
Returns the lowest/worst oblique resolution in the entire image.
int BetaLines() const
Returns the number of lines in the beta cube.
double HighestImageResolution()
Returns the highest/best resolution in the entire image.
Base class for Map TProjections.
QString instrumentId()
This method returns the InstrumentId as it appears in the cube.
int p_childBand
Band value for child.
bool p_ringRangeComputed
Flag showing if ring range was computed successfully.
virtual double LineResolution()
Returns the line resolution at the current position in meters.
CameraDistortionMap * p_distortionMap
A pointer to the DistortionMap.
double p_childSample
Sample value for child.
virtual bool SetUniversalGround(const double latitude, const double longitude)
Sets the lat/lon values to get the sample/line values.
double p_pixelPitch
The pixel pitch, in millimeters per pixel.
PvlObjectIterator findObject(const QString &name, PvlObjectIterator beg, PvlObjectIterator end)
Find the index of object with a specified name, between two indexes.
bool isValid() const
Test if this distance has been initialized or not.
virtual QList< QPointF > PixelIfovOffsets()
Returns the pixel ifov offsets from center of pixel, which defaults to the (pixel pitch * summing mod...
virtual double WorldX() const
This returns the world X coordinate provided SetGround, SetCoordinate, SetUniversalGround,...
double ParentSample() const
Return parent sample.
double CelestialNorthClockAngle()
Computes the celestial north clock angle at the current line/sample or ra/dec.
double p_minlon180
The minimum longitude in the 180 domain.
AlphaCube * p_alphaCube
A pointer to the AlphaCube.
Distort/undistort focal plane coordinates.
double p_minres
The minimum resolution.
void SetFocalPlaneMap(CameraFocalPlaneMap *map)
Sets the Focal Plane Map.
double NorthAzimuth()
Returns the North Azimuth.
IO Handler for Isis Cubes.
This class is used to rewrite the "alpha" keywords out of the AlphaCube group or Instrument group.
int AlphaLines() const
Returns the number of lines in the alpha cube.
QString m_spacecraftNameLong
Full spacecraft name.
virtual bool SetDetector(const double sample, const double line)
Compute parent position from a detector coordinate.
virtual int bandCount() const
Returns the number of virtual bands for the cube.
Defines an angle and provides unit conversions.
virtual bool SetWorld(const double x, const double y)
This method is used to set a world coordinate.
int p_samples
The number of samples in the image.
bool SetImageMapProjection(const double sample, const double line, ShapeModel *shape)
Sets the sample/line values of the image to get the lat/lon values for a Map Projected image.
virtual double LineScaleFactor() const
Return scaling factor for computing line resolution.
double DetectorLine() const
Return detector line.
double p_maxRingRadius
The maximum ring radius.
Longitude GetLongitude() const
Return the body-fixed longitude for the surface point.
double p_maxlon
The maximum longitude.
int AlphaSamples() const
Returns the number of samples in the alpha cube.
double BetaSample(double alphaSample)
Returns a beta sample given an alpha sample.
const double Null
Value for an Isis Null pixel.
int Bands() const
Returns the number of bands in the image.
static Isis::Projection * CreateFromCube(Isis::Cube &cube)
This method is a helper method.
bool p_raDecRangeComputed
Flag showing if the raDec range has been computed successfully.
double p_maxRingLongitude180
The maximum ring longitude in the 180 domain.
void addGroup(const Isis::PvlGroup &group)
Add a group to the object.
virtual bool IsBandIndependent()
Virtual method that checks if the band is independent.
double kilometers() const
Get the displacement in kilometers.
int Samples() const
Returns the number of samples in the image.
bool p_pointComputed
Flag showing if Sample/Line has been computed.
void BasicMapping(Pvl &map)
Writes the basic mapping group to the specified Pvl.
bool p_groundRangeComputed
Flag showing if ground range was computed successfully.
double RaDecResolution()
Returns the RaDec resolution.
Class for computing sensor ground coordinates.
double p_maxra
The maxumum right ascension.
int p_geometricTilingStartSize
The ideal geometric tile size to start with when projecting.
bool hasNormal() const
Returns surface point normal status.
double toDouble(const QString &string)
Global function to convert from a string to a double.
CameraFocalPlaneMap * FocalPlaneMap()
Returns a pointer to the CameraFocalPlaneMap object.
int BetaSamples() const
Returns the number of samples in the beta cube.
@ Programmer
This error is for when a programmer made an API call that was illegal.
double FocalLength() const
Returns the focal length.
Namespace for the standard library.
void LoadCache()
This loads the spice cache big enough for this image.
double FocalPlaneX() const
Define shapes and provide utilities for Isis targets.
QString m_instrumentNameShort
Shortened instrument name.
Convert between distorted focal plane and detector coordinates.
CameraGroundMap * GroundMap()
Returns a pointer to the CameraGroundMap object.
CameraDetectorMap * DetectorMap()
Returns a pointer to the CameraDetectorMap object.
double PixelPitch() const
Returns the pixel pitch.
virtual double PixelResolution()
Returns the pixel resolution at the current position in meters/pixel.
Pvl * label() const
Returns a pointer to the IsisLabel object associated with the cube.
virtual bool SetDetector(const double sample, const double line)
Compute distorted focal plane coordinate from detector position (sampel,line)
Convert between undistorted focal plane and ra/dec coordinates.
virtual int SpkCenterId() const
Provides the center of motion body for SPK NAIF kernel.
virtual bool SetFocalPlane(double dx, double dy)
Compute undistorted focal plane x/y.
virtual double UniversalLongitude()
This returns a universal longitude (positive east in 0 to 360 domain).
bool GroundRange(double &minlat, double &maxlat, double &minlon, double &maxlon, Pvl &pvl)
Computes the Ground Range.
double meters() const
Get the distance in meters.
double p_minobliqueres
The minimum oblique resolution.
virtual void instrumentBodyFixedPosition(double p[3]) const
Returns the spacecraft position in body-fixed frame km units.
CameraGroundMap * p_groundMap
A pointer to the GroundMap.
double degrees() const
Get the angle in units of Degrees.
void GetLocalNormal(double normal[3])
This method will find the local normal at the current (sample, line) and set it to the passed in arra...
@ Triaxial
These projections are used to map triaxial and irregular-shaped bodies.
virtual bool SetFocalPlane(const double ux, const double uy, const double uz)
Compute ground position from focal plane coordinate.
double HighestObliqueImageResolution()
Returns the highest/best oblique resolution in the entire image.
int Lines() const
Returns the number of lines in the image.
void LocalPhotometricAngles(Angle &phase, Angle &incidence, Angle &emission, bool &success)
Calculates LOCAL photometric angles using the DEM (not ellipsoid).
bool hasIntersection()
Returns intersection status.
void Coordinate(double p[3]) const
Returns the x,y,z of the surface intersection in BodyFixed km.
bool IsSky() const
Returns true if projection is sky and false if it is land.
Adds specific functionality to C++ strings.
virtual double EmissionAngle() const
Returns the emission angle in degrees.
QString instrumentNameShort() const
This method returns the shortened instrument name.
virtual double SampleResolution()
Returns the sample resolution at the current position in meters.
virtual double Line() const
Returns the current line number.
QString spacecraftNameLong() const
This method returns the full spacecraft name.
SpiceDouble getDouble(const QString &key, int index=0)
This returns a value from the NAIF text pool.
This is free and unencumbered software released into the public domain.
SpiceInt naifSpkCode() const
This returns the NAIF SPK code to use when reading from SPK kernels.
double ParentLine() const
Return parent line.
virtual double ObliqueLineResolution()
Returns the oblique line resolution at the current position in meters.
int ReferenceBand() const
Returns the reference band.
QString m_spacecraftNameShort
Shortened spacecraft name.
double FocalPlaneY() const
double UndistortedFocalPlaneY() const
Gets the y-value in the undistorted focal plane coordinate system.
double p_minra180
The minimum right ascension in the 180 domain.
double LowestImageResolution()
Returns the lowest/worst resolution in the entire image.
bool HasProjection()
Checks to see if the camera object has a projection.
double SunAzimuth()
Returns the Sun Azimuth.
QString spacecraftNameShort() const
This method returns the shortened spacecraft name.
bool ringRange(double &minRingRadius, double &maxRingRadius, double &minRingLongitude, double &maxRingLongitude, Pvl &pvl)
Analogous to the above Ground Range method.
double p_maxlat
The maximum latitude.
virtual double DetectorResolution()
Returns the detector resolution at the current position in meters.
void GetGeometricTilingHint(int &startSize, int &endSize)
This will get the geometric tiling hint; these values are typically used for ProcessRubberSheet::SetT...
This class defines a body-fixed surface point.
int p_geometricTilingEndSize
The ideal geometric tile size to end with when projecting.
void SetFocalLength()
Reads the focal length from the instrument kernel.
@ Radians
Radians are generally used in mathematical equations, 0-2*PI is one circle, however these are more di...
This is free and unencumbered software released into the public domain.
Distance LocalRadius() const
Returns the local radius at the intersection point.
double p_minra
The minimum right ascension.
@ User
A type of error that could only have occurred due to a mistake on the user's part (e....
std::vector< double > normal()
Returns the local surface normal at the current intersection point.
double UniversalRingRadius()
This returns a universal radius, which is just the radius in meters.
virtual double UniversalLatitude() const
Returns the planetocentric latitude, in degrees, at the surface intersection point in the body fixed ...
virtual bool SetGround(const Latitude &lat, const Longitude &lon)
Compute undistorted focal plane coordinate from ground position.
double radians() const
Convert an angle to a double.
void SetDistortionMap(CameraDistortionMap *map, bool deleteExisting=true)
Sets the Distortion Map.