File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
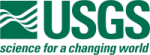 |
Isis 3 Programmer Reference
|
11 template<
class T>
class QVector;
76 virtual bool intersectSurface(std::vector<double> observerPos,
77 std::vector<double> lookDirection)=0;
83 const std::vector<double> &observerPos,
84 const bool &backCheck =
true);
85 virtual bool intersectSurface(
const SurfacePoint &surfpt,
86 const std::vector<double> &observerPos,
87 const bool &backCheck =
true);
98 virtual void calculateDefaultNormal() = 0;
106 virtual void calculateSurfaceNormal() = 0;
112 virtual double emissionAngle(
const std::vector<double> & sB);
118 virtual double phaseAngle(
const std::vector<double> &sB,
119 const std::vector<double> &uB);
135 QString
name()
const;
144 std::vector<double>
normal();
147 virtual bool isVisibleFrom(
const std::vector<double> observerPos,
148 const std::vector<double> lookDirection);
153 void setNormal(
const std::vector<double>);
154 void setNormal(
const double a,
const double b,
const double c);
164 const std::vector<double> &observerLookVectorToTarget);
std::vector< double > m_normal
Local normal of current intersection point.
virtual double emissionAngle(const std::vector< double > &sB)
Computes and returns emission angle, in degrees, given the observer position.
virtual void clearSurfacePoint()
Clears or resets the current surface point.
This class is designed to encapsulate the concept of a Latitude.
SurfacePoint * surfaceIntersection() const
Returns the surface intersection for this ShapeModel.
virtual void setSurfacePoint(const SurfacePoint &surfacePoint)
Set surface intersection point.
std::vector< Distance > targetRadii() const
Returns the radii of the body in km.
void setHasIntersection(bool b)
Sets the flag to indicate whether this ShapeModel has an intersection.
SurfacePoint * m_surfacePoint
< Name of the shape
virtual double incidenceAngle(const std::vector< double > &uB)
Computes and returns incidence angle, in degrees, given the illuminator position.
QString name() const
Gets the shape name.
virtual ~ShapeModel()=0
Virtual destructor to destroy the ShapeModel object.
bool m_hasNormal
indicates normal has been computed
void Initialize()
Initializes the ShapeModel private variables.
void setNormal(const std::vector< double >)
Sets the normal for the currect intersection point.
Distance measurement, usually in meters.
This class is designed to encapsulate the concept of a Longitude.
bool m_hasEllipsoidIntersection
Indicates the ellipsoid was successfully intersected.
void setName(QString name)
Sets the shape name.
double resolution()
Convenience method to get pixel resolution (m/pix) at current intersection point.
virtual bool isDEM() const =0
Indicates whether this shape model is from a DEM.
ShapeModel()
Default constructor creates ShapeModel object, initializing name to an empty string,...
bool intersectEllipsoid(const std::vector< double > observerPosRelativeToTarget, const std::vector< double > &observerLookVectorToTarget)
Finds the intersection point on the ellipsoid model using the given position of the observer (spacecr...
bool m_hasIntersection
indicates good intersection exists
void setHasNormal(bool status)
Sets the flag to indicate whether this ShapeModel has a surface normal.
bool hasEllipsoidIntersection()
Returns the status of the ellipsoid model intersection.
bool hasNormal() const
Returns surface point normal status.
bool hasValidTarget() const
Returns the status of the target.
Define shapes and provide utilities for Isis targets.
virtual double phaseAngle(const std::vector< double > &sB, const std::vector< double > &uB)
Computes and returns phase angle, in degrees, given the positions of the observer and illuminator.
bool hasIntersection()
Returns intersection status.
This is free and unencumbered software released into the public domain.
void calculateEllipsoidalSurfaceNormal()
Calculates the ellipsoidal surface normal.
This class is used to create and store valid Isis targets.
virtual bool isVisibleFrom(const std::vector< double > observerPos, const std::vector< double > lookDirection)
Default occulsion implementation.
This class defines a body-fixed surface point.
This is free and unencumbered software released into the public domain.
std::vector< double > normal()
Returns the local surface normal at the current intersection point.