Loading [MathJax]/jax/output/NativeMML/config.js
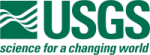 |
Isis 3 Programmer Reference
|
10 #include <QSharedPointer>
80 std::vector<Distance>
radii()
const;
92 std::vector<Angle> poleRaCoefs();
93 std::vector<Angle> poleDecCoefs();
94 std::vector<Angle> pmCoefs();
101 std::vector<double> poleRaNutPrecCoefs();
102 std::vector<double> poleDecNutPrecCoefs();
104 std::vector<double> pmNutPrecCoefs();
106 std::vector<Angle> sysNutPrecConstants();
107 std::vector<Angle> sysNutPrecCoefs();
static PvlGroup radiiGroup(QString target)
Creates a Pvl Group with keywords TargetName, EquitorialRadius, and PolarRadius.
void setShapeEllipsoid()
Set the shape to the ellipsoid and save the original shape.
ShapeModel * shape() const
Return the shape.
SpiceInt * m_systemCode
The NaifBodyCode of the targets planetary system If the target is sky, then what should this be?...
bool isSky() const
Return if our target is the sky.
void setRadii(std::vector< Distance > radii)
Sets the radii of the body.
void setSpice(Spice *spice)
Set the Spice pointer for the Target.
void setName(QString name)
Set the name for the Target.
ShapeModel * m_shape
target shape model
Container for cube-like labels.
SpiceInt naifBodyCode() const
This returns the NAIF body code of the target.
ShapeModel * m_originalShape
target original shape model
Spice * m_spice
parent Spice object, needed to get pixel resolution in ShapeModels
std::vector< Distance > m_radii
target radii
std::vector< Distance > radii() const
Returns the radii of the body in km.
~Target()
Destroys the Target.
Contains multiple PvlContainers.
bool m_sky
flag indicating target is the sky
Target()
Constructs an empty Target object.
void restoreShape()
Restores the shape to the original after setShapeEllipsoid has overridden it.
QString * m_systemName
name of the planetary system of the target
static SpiceInt lookupNaifBodyCode(QString name)
This returns the NAIF body code of the target indicated in the labels.
Obtain SPICE information for a spacecraft.
SpiceInt * m_bodyCode
The NaifBodyCode value, if it exists in the labels.
SpiceInt naifPlanetSystemCode() const
This returns the NAIF planet system body code of the target.
Define shapes and provide utilities for Isis targets.
Spice * spice() const
Return the spice object.
QString systemName() const
Return planet system name.
void init()
Initialize member variables.
QString * m_name
target name
This class is used to create and store valid Isis targets.
This is free and unencumbered software released into the public domain.
QString name() const
Return target name.