File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
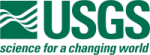 |
Isis 3 Programmer Reference
|
This is free and unencumbered software released into the public domain.
double positiveWest(Angle::Units units=Angle::Radians) const
Get the longitude in the PositiveWest coordinate system.
double positiveEast(Angle::Units units=Angle::Radians) const
Get the longitude in the PositiveEast coordinate system.
Longitude & operator=(const Longitude &longitudeToCopy)
Same as positiveEast.
@ Domain180
As the longitude increases the actual position is more west.
@ Domain360
As the longitude increases the actual position is more east.
Longitude force180Domain() const
This returns a longitude that is constricted to -180 to 180 degrees.
void setPositiveEast(double longitude, Angle::Units units=Angle::Radians)
Set the longitude given a value in the PositiveEast longitude system.
This class is designed to encapsulate the concept of a Longitude.
Domain m_currentDomain
This is necessary for converting to PositiveWest and back.
~Longitude()
This cleans up the Longitude class.
Contains multiple PvlContainers.
void setPositiveWest(double longitude, Angle::Units units=Angle::Radians)
Set the longitude given a value in the PositiveWest longitude system.
Units
The set of usable angle measurement units.
Defines an angle and provides unit conversions.
Direction
Possible longitude directions: Is a positive longitude towards east or towards west?
static QList< QPair< Longitude, Longitude > > to360Range(Longitude startLon, Longitude endLon)
Calculates where the longitude range is in 0-360.
@ PositiveWest
As the longitude increases the actual position is more west.
Domain
Use LongitudeDomain360 if 0-360 is the primary range of the longitude values with 180 being the 'cent...
Longitude()
Create a blank Longitude object with 0-360 domain.
Longitude force360Domain() const
This returns a longitude that is constricted to 0-360 degrees.
@ PositiveEast
As the longitude increases the actual position is more east.
@ Radians
Radians are generally used in mathematical equations, 0-2*PI is one circle, however these are more di...
This is free and unencumbered software released into the public domain.
bool inRange(Longitude min, Longitude max) const
Checks if this longitude value is within the given range.