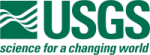 |
Isis 3 Programmer Reference
|
14 #include "Constants.h"
15 #include "IException.h"
18 #include "SpecialPixel.h"
41 Angle(longitude, longitudeUnits) {
42 if(mapping[
"LongitudeDomain"][0] ==
"360") {
45 else if(mapping[
"LongitudeDomain"][0] ==
"180") {
49 IString msg =
"Longitude domain [" +
50 IString(mapping[
"LongitudeDomain"][0]) +
"] not recognized";
54 if(mapping[
"LongitudeDirection"][0] ==
"PositiveEast") {
57 else if(mapping[
"LongitudeDirection"][0] ==
"PositiveWest") {
61 IString msg =
"Longitude direction [" +
62 IString(mapping[
"LongitudeDirection"][0]) +
"] not recognized";
88 IString msg =
"Longitude direction [" +
IString(lonDir) +
"] not valid";
105 Angle(longitude, longitudeUnits) {
115 IString msg =
"Longitude direction [" +
IString(lonDir) +
"] not valid";
127 :
Angle(longitudeToCopy) {
159 double longitude =
angle(units);
164 double halfWrap = wrapPoint / 2.0;
166 int numPlanetWraps = qFloor(longitude / wrapPoint);
171 if (numPlanetWraps != 0 && qFuzzyCompare(numPlanetWraps * wrapPoint, longitude)) {
172 if (numPlanetWraps > 0)
178 longitude -= numPlanetWraps * wrapPoint;
179 longitude = -(longitude - halfWrap) + halfWrap;
180 longitude -= numPlanetWraps * wrapPoint;
185 longitude = -1 * longitude;
215 double halfWrap = wrapPoint / 2.0;
217 int numPlanetWraps = qFloor(longitude / wrapPoint);
222 if (numPlanetWraps != 0 && qFuzzyCompare(numPlanetWraps * wrapPoint, longitude)) {
223 if (numPlanetWraps > 0)
230 longitude -= numPlanetWraps * wrapPoint;
231 longitude = -(longitude - halfWrap) + halfWrap;
232 longitude -= numPlanetWraps * wrapPoint;
237 longitude = -1 * longitude;
253 if(
this == &longitudeToCopy)
return *
this;
273 if (qFuzzyCompare(
degrees(), 360.0)) {
274 resultantLongitude = 360.0;
277 resultantLongitude -= 360 * qFloor(resultantLongitude / 360);
327 foreach (range, ranges) {
328 if (thisLon >= range.first && thisLon <= range.second) {
332 double thisLonRadians = thisLon.
radians();
333 double rangeStartRadians = range.first.radians();
334 double rangeEndRadians = range.second.radians();
336 if (qFuzzyCompare(thisLonRadians, rangeStartRadians) ||
337 qFuzzyCompare(thisLonRadians, rangeEndRadians)) {
342 if ((qFuzzyCompare(thisLonRadians, 0.0) || qFuzzyCompare(thisLonRadians, 2.0 *
PI)) &&
343 (qFuzzyCompare(rangeStartRadians, 0.0) ||
344 qFuzzyCompare(rangeEndRadians, 2.0 *
PI))) {
368 if (startLon.
isValid() && endLon.
isValid() && startLon < endLon) {
380 if (endLon2 < startLon) {
381 range.append(qMakePair(startLon2, endLon2));
391 range.append(qMakePair(startLon, endLon));
@ Degrees
Degrees are generally considered more human readable, 0-360 is one circle, however most math does not...
const double PI
The mathematical constant PI.
This is free and unencumbered software released into the public domain.
double positiveWest(Angle::Units units=Angle::Radians) const
Get the longitude in the PositiveWest coordinate system.
double positiveEast(Angle::Units units=Angle::Radians) const
Get the longitude in the PositiveEast coordinate system.
Longitude & operator=(const Longitude &longitudeToCopy)
Same as positiveEast.
@ Domain180
As the longitude increases the actual position is more west.
@ Domain360
As the longitude increases the actual position is more east.
double unitWrapValue(const Units &unit) const
Return wrap value in desired units.
Longitude force180Domain() const
This returns a longitude that is constricted to -180 to 180 degrees.
bool IsSpecial(const double d)
Returns if the input pixel is special.
void setPositiveEast(double longitude, Angle::Units units=Angle::Radians)
Set the longitude given a value in the PositiveEast longitude system.
This class is designed to encapsulate the concept of a Longitude.
Domain m_currentDomain
This is necessary for converting to PositiveWest and back.
~Longitude()
This cleans up the Longitude class.
Contains multiple PvlContainers.
void setPositiveWest(double longitude, Angle::Units units=Angle::Radians)
Set the longitude given a value in the PositiveWest longitude system.
Units
The set of usable angle measurement units.
Defines an angle and provides unit conversions.
Direction
Possible longitude directions: Is a positive longitude towards east or towards west?
@ Programmer
This error is for when a programmer made an API call that was illegal.
static QList< QPair< Longitude, Longitude > > to360Range(Longitude startLon, Longitude endLon)
Calculates where the longitude range is in 0-360.
bool isValid() const
This indicates whether we have a legitimate angle stored or are in an unset, or invalid,...
Angle()
Constructs a blank angle object which needs a value to be set in order to do any calculations.
This is free and unencumbered software released into the public domain.
static Angle fullRotation()
Makes an angle to represent a full rotation (0-360 or 0-2pi).
double degrees() const
Get the angle in units of Degrees.
virtual void setAngle(const double &angle, const Units &unit)
Set angle value in desired units.
@ PositiveWest
As the longitude increases the actual position is more west.
Adds specific functionality to C++ strings.
Domain
Use LongitudeDomain360 if 0-360 is the primary range of the longitude values with 180 being the 'cent...
Longitude()
Create a blank Longitude object with 0-360 domain.
Longitude force360Domain() const
This returns a longitude that is constricted to 0-360 degrees.
@ PositiveEast
As the longitude increases the actual position is more east.
virtual double angle(const Units &unit) const
Return angle value in desired units.
@ Radians
Radians are generally used in mathematical equations, 0-2*PI is one circle, however these are more di...
This is free and unencumbered software released into the public domain.
bool inRange(Longitude min, Longitude max) const
Checks if this longitude value is within the given range.
double radians() const
Convert an angle to a double.