File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
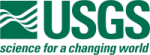 |
Isis 3 Programmer Reference
|
13 #include <QStringList>
15 #include "Constants.h"
20 bool toBool(
const QString &);
21 int toInt(
const QString &);
28 QString
toString(
const unsigned int &);
30 QString
toString(
double,
int precision = 14);
169 IString(
const std::string &str);
173 IString(
const double &num,
const int piPrecision = 14);
180 static std::string
Trim(
const std::string &chars,
const std::string &str);
183 static std::string
TrimHead(
const std::string &chars,
const std::string &str);
186 static std::string
TrimTail(
const std::string &chars,
const std::string &str);
189 static std::string
UpCase(
const std::string &str);
192 static std::string
DownCase(
const std::string &str);
195 static int ToInteger(
const std::string &str);
201 static double ToDouble(
const std::string &str);
203 QString
ToQt()
const;
204 static QString
ToQt(
const std::string &str);
207 static int Split(
const char separator,
const std::string &instr,
208 std::vector<std::string> &tokens,
209 bool allowEmptyEntries =
true);
212 static std::string
Compress(
const std::string &str,
bool force =
false);
215 int maxReplaceCount = 20);
216 static std::string
Replace(
const std::string &str,
217 const std::string &from,
218 const std::string &to,
219 int maxReplacementCount = 20);
221 IString Replace(
const std::string &from,
const std::string &to ,
bool honorquotes);
222 static IString Replace(
const std::string &str,
const std::string &from,
223 const std::string &to ,
bool honorquotes);
226 static std::string
Convert(
const std::string &str,
227 const std::string &listofchars,
234 static std::string
Remove(
const std::string &del,
const std::string &str);
241 operator int()
const {
260 operator double()
const {
291 bool Equal(
const std::string &str)
const;
292 static bool Equal(
const std::string &str1,
const std::string &str2);
294 static std::string
ToStd(
const QString &str);
300 void SetDouble(
const double &value,
const int piPrecision = 14);
303 std::ostream &
operator<<(std::ostream &os,
const QString &
string);
304 std::ostream &
operator<<(std::ostream &os,
const QStringRef &
string);
IString Replace(const std::string &from, const std::string &to, int maxReplaceCount=20)
Replaces all instances of the first input string with the second input string.
double ToDouble() const
Returns the floating point value the IString represents.
QDebug operator<<(QDebug debug, const Hillshade &hillshade)
Print this class out to a QDebug object.
IString DownCase()
Converts all upper case letters in the object IString into lower case characters.
IString TrimTail(const std::string &chars)
Trims the input characters from the end of the object IString.
static std::string ToStd(const QString &str)
Converts a Qt string into a std::string.
IString()
Constructs an empty IString object.
IString Trim(const std::string &chars)
Removes characters from the beginning and end of the IString.
IString ConvertWhiteSpace()
Returns the string with all "new lines", "carriage returns", "tabs", "form feeds",...
int ToInteger() const
Returns the object string as an integer.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
IString Convert(const std::string &listofchars, const char &to)
Returns the string with all occurrences of any character in the "from" argument converted to the "to"...
IString Compress(bool force=false)
Collapses multiple spaces into single spaces.
IString UpCase()
Converst any lower case characters in the object IString with uppercase characters.
BigInt ToBigInteger() const
Returns the BigInt representation of the object IString.
BigInt toBigInt(const QString &string)
Global function to convert from a string to a "big" integer.
int toInt(const QString &string)
Global function to convert from a string to an integer.
IString & operator=(const int &value)
Attempts to convert the stirng to a QStirng (Qt) and return that IString.
long long int BigInt
Big int.
void SetDouble(const double &value, const int piPrecision=14)
Performs the conversion necessary to represent a floating-point value as a string.
double toDouble(const QString &string)
Global function to convert from a string to a double.
bool toBool(const QString &string)
Global function to convert from a string to a boolean.
static int Split(const char separator, const std::string &instr, std::vector< std::string > &tokens, bool allowEmptyEntries=true)
Find separators between characters and split them into strings.
bool Equal(const std::string &str) const
Compare a string to the object IString.
IString Token(const IString &separator)
Returns the first token in the IString.
Adds specific functionality to C++ strings.
IString TrimHead(const std::string &chars)
Trims The input characters from the beginning of the object IString.
IString Remove(const std::string &del)
Remove all instances of any character in the string from the IString.
This is free and unencumbered software released into the public domain.
QString ToQt() const
Retuns the object string as a QString.