File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
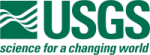 |
Isis 3 Programmer Reference
|
17 #include "SpecialPixel.h"
65 Hillshade::~Hillshade() {
168 QObject::tr(
"Hillshade requires a 3x3x1 portal of data, but a %1x%2x%3 "
169 "portal of data was provided instead")
177 QObject::tr(
"Hillshade requires a valid azimuth angle (sun direction) to "
184 QObject::tr(
"Hillshade azimuth angle [%1] must be between 0 and 360 degrees")
191 QObject::tr(
"Hillshade requires a valid zenith angle (solar elevation) to "
198 QObject::tr(
"Hillshade zenith angle [%1] must be between 0 and 90 degrees")
205 QObject::tr(
"Hillshade requires a pixel resolution (meters/pixel) to "
212 QObject::tr(
"Hillshade requires a non-zero pixel resolution (meters/pixel) "
227 double result =
Null;
230 bool anySpecialPixels =
false;
231 for(
int i = 0; i < input.
size(); ++i) {
233 anySpecialPixels =
true;
238 if (!anySpecialPixels) {
253 double p = ( (-1) * input[0] + (0) * input[1] + (1) * input[2]
254 + (-1) * input[3] + (0) * input[4] + (1) * input[5]
255 + (-1) * input[6] + (0) * input[7] + (1) * input[8]) / (3.0 *
m_pixelResolution);
257 double q = ( (-1) * input[0] + (-1) * input[1] + (-1) * input[2]
258 + (0) * input[3] + (0) * input[4] + (0) * input[5]
280 double numerator = 1.0 + p0 * p + q0 * q;
282 double denominator = sqrt(1 + p * p + q * q) * sqrt(1 + p0 * p0 + q0 * q0);
284 result = numerator / denominator;
315 QString resolution =
"Null";
320 debug <<
"Hillshade[ azimuth =" << hillshade.
azimuth().
toString().toLatin1().data()
322 <<
"resolution =" << resolution.toLatin1().data() <<
"]";
@ Degrees
Degrees are generally considered more human readable, 0-360 is one circle, however most math does not...
Hillshade()
Create a default-constructed Hillshade.
virtual QString toString(bool includeUnits=true) const
Get the angle in human-readable form.
int SampleDimension() const
Returns the number of samples in the shape buffer.
QDebug operator<<(QDebug debug, const Hillshade &hillshade)
Print this class out to a QDebug object.
@ Unknown
A type of error that cannot be classified as any of the other error types.
void setResolution(double resolution)
The resolution is the meters per pixel of the input to shadedValue().
Angle zenith() const
Get the current zenith angle.
void setAzimuth(Angle azimuth)
The azimuth is the direction of the light.
void swap(Hillshade &other)
Swap class data with other; this cannot throw an exception.
Angle * m_zenith
This is the altitide of the light, with 0 directly overhead and 90 at the horizon.
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
bool IsSpecial(const double d)
Returns if the input pixel is special.
double m_pixelResolution
meters per pixel
Buffer for reading and writing cube data.
Angle * m_azimuth
This is direction of the light, with 0 at north.
Angle azimuth() const
Get the current azimuth angle.
void setZenith(Angle zenith)
The zenith is the altitude/solar elevation of the light.
int LineDimension() const
Returns the number of lines in the shape buffer.
double resolution() const
Get the current resolution (meters per pixel).
Defines an angle and provides unit conversions.
const double Null
Value for an Isis Null pixel.
Hillshade & operator=(const Hillshade &rhs)
Assignment operator.
double shadedValue(Buffer &input) const
Calculate the shaded value from a 3x3x1 window.
@ Programmer
This error is for when a programmer made an API call that was illegal.
bool isValid() const
This indicates whether we have a legitimate angle stored or are in an unset, or invalid,...
Calculate light intensity reflected off a local slope of DEM.
static Angle fullRotation()
Makes an angle to represent a full rotation (0-360 or 0-2pi).
int size() const
Returns the total number of pixels in the shape buffer.
int BandDimension() const
Returns the number of bands in the shape buffer.
This is free and unencumbered software released into the public domain.
double radians() const
Convert an angle to a double.