File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
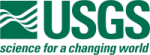 |
Isis 3 Programmer Reference
|
15 #include "Constants.h"
16 #include "CubeManager.h"
18 #include "EllipsoidShape.h"
19 #include "IException.h"
23 #include "Longitude.h"
24 #include "NaifStatus.h"
25 #include "Projection.h"
26 #include "ShapeModel.h"
27 #include "SpecialPixel.h"
28 #include "SurfacePoint.h"
30 #include "UniqueIOCachingAlgorithm.h"
32 #define MAX(x,y) (((x) > (y)) ? (x) : (y))
83 QString message =
"Pixel Ifov offsets not implemented for this camera.";
149 vector<double> lookC(v, v + 3);
159 memcpy(
m_lookB, &lookB[0],
sizeof(
double) * 3);
174 return target()->
shape()->intersectSurface(sB, lookB);
313 std::vector<double> sunB(
m_uB,
m_uB+3);
336 std::vector<double> sunB(
m_uB,
m_uB+3);
360 const double longitude,
375 shape->intersectSurface(lat, lon,
402 const double longitude,
453 shape->intersectSurface(surfacePt,
489 const vector<double> &sB =
536 vector<double> lookB(3);
587 vector<double> lookB(3);
594 recrad_c((SpiceDouble *)&lookJ[0], &range, &
m_ra, &
m_dec);
609 vector<double> lookJ(3);
610 radrec_c(1.0, ra *
PI / 180.0, dec *
PI / 180.0, (SpiceDouble *)&lookJ[0]);
626 scSurfaceVector[0] =
m_lookB[0];
627 scSurfaceVector[1] =
m_lookB[1];
628 scSurfaceVector[2] =
m_lookB[2];
638 SpiceDouble psB[3], upsB[3];
649 vsub_c(pB, (SpiceDouble *) &sB[0], psB);
650 unorm_c(psB, upsB, &dist);
666 if (lst < 0.0) lst += 24.0;
667 if (lst > 24.0) lst -= 24.0;
689 double dist = sqrt(xChange*xChange + yChange*yChange + zChange*zChange);
690 dist /= 149597870.691;
709 double rlat = lat *
PI / 180.0;
710 double rlon = lon *
PI / 180.0;
720 double xChange = spB[0] - ssB[0];
721 double yChange = spB[1] - ssB[1];
722 double zChange = spB[2] - ssB[2];
725 double dist = sqrt(xChange*xChange + yChange*yChange + zChange*zChange);
double kilometers() const
Get the distance in kilometers.
@ Degrees
Degrees are generally considered more human readable, 0-360 is one circle, however most math does not...
virtual void setTime(const iTime &time)
Sets the ephemeris time and reads the spacecraft and sun position from the kernels at that instant in...
iTime time() const
Returns the ephemeris time in seconds which was used to obtain the spacecraft and sun positions.
virtual double emissionAngle(const std::vector< double > &sB)
Computes and returns emission angle, in degrees, given the observer position.
void setShapeEllipsoid()
Set the shape to the ellipsoid and save the original shape.
void SpacecraftSurfaceVector(double scSurfaceVector[3]) const
Sets the vector between the spacecraft and surface point in body-fixed.
ShapeModel * shape() const
Return the shape.
virtual void subSolarPoint(double &lat, double &lon)
Returns the sub-solar latitude/longitude in universal coordinates (0-360 positive east,...
virtual void clearSurfacePoint()
Clears or resets the current surface point.
virtual void subSpacecraftPoint(double &lat, double &lon)
Returns the sub-spacecraft latitude/longitude in universal coordinates (0-360 positive east,...
virtual SpicePosition * instrumentPosition() const
Accessor method for the instrument position.
virtual ~Sensor()
Destroys the Sensor.
const double PI
The mathematical constant PI.
double SpacecraftAltitude()
Returns the distance from the spacecraft to the subspacecraft point in km.
bool SetUniversalGround(const double latitude, const double longitude, bool backCheck=true)
This is the opposite routine for SetLookDirection.
This class is designed to encapsulate the concept of a Latitude.
SurfacePoint * surfaceIntersection() const
Returns the surface intersection for this ShapeModel.
bool HasSurfaceIntersection() const
Returns if the last call to either SetLookDirection or SetUniversalGround had a valid intersection wi...
Parse and return pieces of a time string.
std::vector< double > lookDirectionJ2000() const
Returns the look direction in the camera coordinate system.
void setHasIntersection(bool b)
Sets the flag to indicate whether this ShapeModel has an intersection.
virtual double incidenceAngle(const std::vector< double > &uB)
Computes and returns incidence angle, in degrees, given the illuminator position.
bool SetGroundLocal(bool backCheck)
Computes look vector.
virtual double Declination()
Returns the declination angle (sky latitude).
Longitude GetLongitude() const
Returns a positive east, 0-360 domain longitude object at the surface intersection point in the body ...
Latitude GetLatitude() const
Return the body-fixed latitude for the surface point.
virtual QList< QPointF > PixelIfovOffsets()
This method is implemented in Camera which defaults to the (pixel pitch * summing mode ) / 2.
double LocalSolarTime()
Return the local solar time in hours.
virtual SpicePosition * sunPosition() const
Accessor method for the sun position.
SurfacePoint GetSurfacePoint() const
Returns the surface point (most efficient accessor).
std::vector< double > J2000Vector(const std::vector< double > &rVec)
Given a direction vector in the reference frame, return a J2000 direction.
virtual double RightAscension()
Returns the right ascension angle (sky longitude).
void IgnoreElevationModel(bool ignore)
This allows you to ignore the cube elevation model and use the ellipse.
Distance measurement, usually in meters.
This class is designed to encapsulate the concept of a Longitude.
std::vector< double > lookDirectionBodyFixed() const
Returns the look direction in the body fixed coordinate system.
virtual Target * target() const
Returns a pointer to the target object.
virtual SpiceRotation * instrumentRotation() const
Accessor method for the instrument rotation.
void setTime(const iTime &time)
By setting the time you essential set the position of the spacecraft and body as indicated in the cla...
SpiceDouble m_ra
Right ascension (sky longitude)
@ Meters
The distance is being specified in meters.
virtual double UniversalLongitude() const
Returns the positive east, 0-360 domain longitude, in degrees, at the surface intersection point in t...
void LookDirection(double v[3]) const
Returns the look direction in the camera coordinate system.
SpiceDouble m_uB[3]
This contains the sun position (u) in the bodyfixed reference frame (B).
void restoreShape()
Restores the shape to the original after setShapeEllipsoid has overridden it.
virtual SpiceRotation * bodyRotation() const
Accessor method for the body rotation.
IO Handler for Isis Cubes.
virtual double IncidenceAngle() const
Returns the incidence angle in degrees.
Obtain SPICE information for a spacecraft.
Latitude GetLatitude() const
Returns a planetocentric latitude object at the surface intersection point in body fixed.
Longitude GetLongitude() const
Return the body-fixed longitude for the surface point.
double kilometers() const
Get the displacement in kilometers.
virtual double SolarDistance() const
Returns the distance between the sun and surface point in AU.
Distance GetLocalRadius() const
Return the radius of the surface point.
@ Programmer
This error is for when a programmer made an API call that was illegal.
Namespace for the standard library.
SpiceDouble m_dec
Decliation (sky latitude)
bool m_newLookB
flag to indicate we need to recompute ra/dec
Define shapes and provide utilities for Isis targets.
bool SetLookDirection(const double v[3])
Sets the look direction of the spacecraft.
bool SetRightAscensionDeclination(const double ra, const double dec)
Given the ra/dec compute the look direction.
virtual double phaseAngle(const std::vector< double > &sB, const std::vector< double > &uB)
Computes and returns phase angle, in degrees, given the positions of the observer and illuminator.
double degrees() const
Get the angle in units of Degrees.
virtual double PhaseAngle() const
Returns the phase angle in degrees.
bool hasIntersection()
Returns intersection status.
void Coordinate(double p[3]) const
Returns the x,y,z of the surface intersection in BodyFixed km.
virtual double EmissionAngle() const
Returns the emission angle in degrees.
std::vector< double > ReferenceVector(const std::vector< double > &jVec)
Given a direction vector in J2000, return a reference frame direction.
SpiceDouble m_lookB[3]
Look direction in body fixed.
void computeRaDec()
Computes the ra/dec from the look direction.
virtual bool isVisibleFrom(const std::vector< double > observerPos, const std::vector< double > lookDirection)
Default occulsion implementation.
This class defines a body-fixed surface point.
This is free and unencumbered software released into the public domain.
virtual double SlantDistance() const
Return the distance between the spacecraft and surface point in kmv.
Distance LocalRadius() const
Returns the local radius at the intersection point.
bool SetGround(const SurfacePoint &surfacePt, bool backCheck=true)
This overloaded method has the opposite function as SetLookDirection.
virtual double UniversalLatitude() const
Returns the planetocentric latitude, in degrees, at the surface intersection point in the body fixed ...