File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
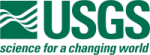 |
Isis 3 Programmer Reference
|
7 #include "Displacement.h"
10 #include "IException.h"
12 #include "SpecialPixel.h"
47 if(displacementUnit ==
Pixels)
63 double pixelsPerMeter) {
131 double pixelsPerMeter) {
156 IString msg =
"Displacement has not been initialized, you must initialize "
157 "it first before comparing with another displacement using [>]";
175 IString msg =
"Displacement has not been initialized, you must initialize "
176 "it first before comparing with another displacement using [<]";
192 if(!isValid() || !displacementToAdd.isValid())
return Displacement();
194 return Displacement(meters() + displacementToAdd.meters(), Meters);
207 if(!isValid() || !displacementToSub.isValid())
return Displacement();
209 Displacement result(meters() - displacementToSub.meters(), Meters);
222 -(
const Distance &distanceToSub)
const {
223 if(!isValid() || !distanceToSub.isValid())
return Displacement();
225 Displacement result(meters() - distanceToSub.meters(), Meters);
360 double resultingDisplacement =
Null;
364 switch(displacementUnit) {
366 resultingDisplacement = displacementInMeters;
370 resultingDisplacement = displacementInMeters / 1000.0;
374 IString msg =
"Cannot call displacement with pixels, ask for another "
380 if(resultingDisplacement ==
Null) {
381 IString msg =
"Displacement does not understand the enumerated value [" +
382 IString(displacementUnit) +
"] as a unit";
386 return resultingDisplacement;
401 double displacementInMeters =
Null;
408 switch(displacementUnit) {
418 IString msg =
"Cannot setDisplacement with pixels, must convert to "
419 "another unit first";
424 if(displacementInMeters ==
Null) {
425 IString msg =
"Displacement does not understand the enumerated value [" +
426 IString(displacementUnit) +
"] as a unit";
double m_displacementInMeters
This is the displacement value that this class is encapsulating, always stored in meters.
@ Meters
The distance is being specified in meters.
void operator/=(const double &valueToDiv)
Divide this displacement by a value and assign the result to ourself.
Displacement operator*(const double &valueToMult) const
Multiply this displacement by a value (5m * 2 = 10m).
Angle operator*(double mult, Angle angle)
Multiply this angle by a double and return the resulting angle.
Units
This is a list of available units to access and store Distances in.
bool operator<(const Displacement &otherDisplacement) const
Compare two displacements with the less than operator.
void setDisplacement(const double &displacement, Units displacementUnit)
This is a helper method to set displacements in a universal manner with uniform error checking.
void setKilometers(double displacementInKilometers)
Set the displacement in kilometers.
@ Pixels
The distance is being specified in pixels.
void operator-=(const Displacement &displacementToSub)
Subtract the given displacement from ourself and assign.
bool IsSpecial(const double d)
Returns if the input pixel is special.
Distance measurement, usually in meters.
Displacement is a signed length, usually in meters.
double operator/(const Displacement &displacementToDiv) const
Divide another displacement into this displacement (5m / 1m = 5).
double meters() const
Get the displacement in meters.
void setMeters(double displacementInMeters)
Set the displacement in meters.
void operator+=(const Displacement &displacementToAdd)
Add and assign the given displacement to ourselves.
Displacement()
This initializes the displacement to an invalid state.
bool isValid() const
Test if this distance has been initialized or not.
double pixels(double pixelsPerMeter=1.0) const
Get the displacement in pixels using the given conversion ratio.
const double Null
Value for an Isis Null pixel.
void setPixels(double distanceInPixels, double pixelsPerMeter=1.0)
Set the displacement in pixels.
double kilometers() const
Get the displacement in kilometers.
double displacement(Units displacementUnit) const
This is a helper method to access displacements in a universal manner with uniform error checking.
@ Programmer
This error is for when a programmer made an API call that was illegal.
bool isValid() const
Test if this displacement has been initialized or not.
double meters() const
Get the distance in meters.
void operator*=(const double &valueToMult)
Multiply this displacement by a value and assign the result to ourself.
bool operator>(const Displacement &otherDisplacement) const
Get the displacement in meters.
Adds specific functionality to C++ strings.
@ Kilometers
The distance is being specified in kilometers.
This is free and unencumbered software released into the public domain.