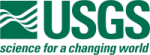 |
Isis 3 Programmer Reference
|
10 #include "Displacement.h"
11 #include "IException.h"
13 #include "SpecialPixel.h"
206 IString msg =
"Distance has not been initialized, you must initialize "
207 "it first before comparing with another distance using [>]";
224 IString msg =
"Distance has not been initialized, you must initialize "
225 "it first before comparing with another distance using [<]";
240 if(
this == &distanceToCopy)
return *
this;
407 double resultingDistance =
Null;
411 switch(distanceUnit) {
413 resultingDistance = distanceInMeters;
417 resultingDistance = distanceInMeters / 1000.0;
421 IString msg =
"Cannot call distance() with pixels, ask for another "
428 resultingDistance = distanceInMeters / 6.9599e8;
432 if(resultingDistance ==
Null) {
433 IString msg =
"Distance does not understand the enumerated value [" +
434 IString(distanceUnit) +
"] as a unit";
438 return resultingDistance;
453 double distanceInMeters =
Null;
460 switch(distanceUnit) {
466 distanceInMeters =
distance * 1000.0;
470 IString msg =
"Cannot setDistance with pixels, must convert to another "
477 distanceInMeters =
distance * 6.9599e8;
481 if(distanceInMeters ==
Null) {
482 IString msg =
"Distance does not understand the enumerated value [" +
483 IString(distanceUnit) +
"] as a unit";
487 if (distanceInMeters < 0.0) {
488 IString msg =
"Negative distances are not supported, the value [" +
489 IString(distanceInMeters) +
" meters] cannot be stored in the Distance "
double kilometers() const
Get the distance in kilometers.
void setPixels(double distanceInPixels, double pixelsPerMeter=1.0)
Set the distance in pixels.
bool operator>(const Distance &otherDistance) const
Compare two distances with the greater than operator.
@ Meters
The distance is being specified in meters.
@ Pixels
The distance is being specified in pixels.
bool operator<(const Distance &otherDistance) const
Compare two distances with the less than operator.
Angle operator*(double mult, Angle angle)
Multiply this angle by a double and return the resulting angle.
Distance & operator=(const Distance &distanceToCopy)
Assign this distance to the value of another distance.
void setKilometers(double distanceInKilometers)
Set the distance in kilometers.
double solarRadii() const
Get the distance in solar radii (a unit of ~696,265km).
Units
This is a list of available units to access and store Distances in.
@ SolarRadii
"Solar radius is a unit of distance used to express the size of stars in astronomy equal to the curre...
QString toString(bool boolToConvert)
Global function to convert a boolean to a string.
bool IsSpecial(const double d)
Returns if the input pixel is special.
void setMeters(double distanceInMeters)
Set the distance in meters.
Distance measurement, usually in meters.
@ Kilometers
The distance is being specified in kilometers.
Displacement is a signed length, usually in meters.
void setSolarRadii(double distanceInSolarRadii)
Set the distance in solar radii.
@ Meters
The distance is being specified in meters.
virtual ~Distance()
This will free the memory allocated by this instance of the Distance class.
QString toString() const
Get a textual representation of this distance.
bool isValid() const
Test if this distance has been initialized or not.
Displacement operator-(const Distance &distanceToSub) const
Subtract another distance from this distance (1km - 1m = 995m).
virtual double distance(Units distanceUnit) const
This is a helper method to access distances in a universal manner with uniform error checking.
const double Null
Value for an Isis Null pixel.
Distance()
This initializes the distance to an invalid state.
void operator/=(const double &valueToDiv)
Divide this distance by a value and assign the result to ourself.
virtual void setDistance(const double &distance, Units distanceUnit)
This is a helper method to set distances in a universal manner with uniform error checking.
@ Programmer
This error is for when a programmer made an API call that was illegal.
void operator+=(const Distance &distanceToAdd)
Add and assign the given distance to ourselves.
void operator*=(const double &valueToMult)
Multiply this distance by a value and assign the result to ourself.
void operator-=(const Distance &distanceToSub)
Subtract and assign the given distance from ourself.
double meters() const
Get the distance in meters.
Distance operator+(const Distance &distanceToAdd) const
Add another distance to this distance (1km + 1m = 1005m)
Adds specific functionality to C++ strings.
double m_distanceInMeters
This is the distance value that this class is encapsulating, always stored in meters.
Distance operator*(const double &valueToMult) const
Multiply this distance by a value (5m * 2 = 10m).
double pixels(double pixelsPerMeter=1.0) const
Get the distance in pixels using the given conversion ratio.
This is free and unencumbered software released into the public domain.
double operator/(const Distance &distanceToDiv) const
Divide another distance into this distance (5m / 1m = 5).