Loading [MathJax]/jax/output/NativeMML/config.js
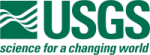 |
Isis 3 Programmer Reference
|
16 #include "AlphaCube.h"
20 class CameraDetectorMap;
21 class CameraFocalPlaneMap;
22 class CameraDistortionMap;
23 class CameraGroundMap;
246 virtual bool SetImage(
const double sample,
const double line);
247 virtual bool SetImage(
const double sample,
const double line,
const double deltaT);
251 const double radius);
257 Angle & emission,
bool &success);
265 virtual void SetBand(
const int band);
266 virtual double Sample()
const;
268 virtual double Line()
const;
270 bool GroundRange(
double &minlat,
double &maxlat,
double &minlon,
271 double &maxlon,
Pvl &pvl);
272 bool ringRange(
double &minRingRadius,
double &maxRingRadius,
273 double &minRingLongitude,
double &maxRingLongitude,
Pvl &pvl);
301 const int band = -1)
const;
313 double &mindec,
double &maxdec);
340 static double GroundAzimuth(
double glat,
double glon,
double slat,
347 int CacheSize(
double startTime,
double endTime);
virtual void SetBand(const int band)
Virtual method that sets the band number.
int CacheSize(double startTime, double endTime)
This method calculates the spice cache size.
virtual int CkFrameId() const =0
Provides the NAIF frame code for an instruments CK kernel.
virtual ~Camera()
Destroys the Camera Object.
void IgnoreProjection(bool ignore)
Set whether or not the camera should ignore the Projection.
bool SetRightAscensionDeclination(const double ra, const double dec)
Sets the right ascension declination.
Projection * p_projection
A pointer to the Projection.
virtual double exposureDuration() const
Return the exposure duration for the pixel that the camera is set to.
double p_minRingRadius
The minimum ring radius.
double p_mindec
The minimum declination.
double p_minlon
The minimum longitude.
void SetGeometricTilingHint(int startSize=128, int endSize=8)
This method sets the best geometric tiling size for projecting from this camera model.
bool SetImageSkyMapProjection(const double sample, const double line, ShapeModel *shape)
Sets the sample/line values of the image to get the lat/lon values for a Skymap Projected image.
CameraSkyMap * p_skyMap
A pointer to the SkyMap.
double p_maxdec
The maximum declination.
int Band() const
Returns the current band.
Convert between parent image coordinates and detector coordinates.
int ParentLines() const
Returns the number of lines in the parent alphacube.
virtual double ObliquePixelResolution()
Returns the oblique pixel resolution at the current position in meters/pixel.
CameraSkyMap * SkyMap()
Returns a pointer to the CameraSkyMap object.
virtual int SpkTargetId() const
Provides target code for instruments SPK NAIF kernel.
double p_childLine
Line value for child.
This class is designed to encapsulate the concept of a Latitude.
void SetSkyMap(CameraSkyMap *map)
Sets the Sky Map.
virtual bool SetImage(const double sample, const double line)
Sets the sample/line values of the image to get the lat/lon values.
double p_maxra180
The maximum right ascension in the 180 domain.
int p_lines
The number of lines in the image.
double p_minRingLongitude180
The minimum ring longitude in the 180 domain.
bool IntersectsLongitudeDomain(Pvl &pvl)
Checks whether the ground range intersects the longitude domain or not.
void SetDetectorMap(CameraDetectorMap *map)
Sets the Detector Map.
void SetGroundMap(CameraGroundMap *map)
Sets the Ground Map.
virtual double Sample() const
Returns the current sample number.
virtual CameraType GetCameraType() const =0
Returns the type of camera that was created.
double p_maxlon180
The maximum longitude in the 180 domain.
QString m_instrumentNameLong
Full instrument name.
virtual double ObliqueDetectorResolution()
This method returns the Oblique Detector Resolution if the Look Vector intersects the target and if t...
double p_maxRingLongitude
The maximum ring longitude (azimuth)
int p_referenceBand
The reference band.
virtual double ObliqueSampleResolution()
Returns the oblique sample resolution at the current position in m.
virtual double resolution()
Returns the resolution of the camera.
void GroundRangeResolution()
Computes the ground range and min/max resolution.
QString instrumentNameLong() const
This method returns the full instrument name.
bool RaDecRange(double &minra, double &maxra, double &mindec, double &maxdec)
Computes the RaDec range.
Container for cube-like labels.
Convert between radar ground range and slant range.
CameraDistortionMap * DistortionMap()
Returns a pointer to the CameraDistortionMap object.
double p_minlat
The minimum latitude.
Camera(Cube &cube)
Constructs the Camera object.
double p_maxobliqueres
The maximum oblique resolution.
bool HasReferenceBand() const
Checks to see if the Camera object has a reference band.
virtual bool SetGround(Latitude latitude, Longitude longitude)
Sets the lat/lon values to get the sample/line values.
int p_bands
The number of bands in the image.
void basicRingMapping(Pvl &map)
Writes the basic mapping group for ring plane to the specified Pvl.
@ RollingShutter
RollingShutter.
bool RawFocalPlanetoImage()
Computes the image coordinate for the current universal ground point.
This class is designed to encapsulate the concept of a Longitude.
QString m_instrumentId
The InstrumentId as it appears on the cube.
Convert between undistorted focal plane and ground coordinates.
double p_maxres
The maximum resolution.
std::pair< double, double > StartEndEphemerisTimes()
Calculates the start and end ephemeris times.
virtual int CkReferenceId() const =0
Provides the NAIF reference code for an instruments CK kernel.
@ LineScan
Line Scan Camera.
double p_focalLength
The focal length, in units of millimeters.
double SpacecraftAzimuth()
Return the Spacecraft Azimuth.
double p_minRingLongitude
The minimum ring longitude (azimuth)
int ParentSamples() const
Returns the number of samples in the parent alphacube.
double OffNadirAngle()
Return the off nadir angle in degrees.
void ringRangeResolution()
Analogous to above GroundRangeResolution method.
bool p_ignoreProjection
Whether or no to ignore the Projection.
bool InCube()
This returns true if the current Sample() or Line() value is outside of the cube (meaning the point m...
void SetPixelPitch()
Reads the Pixel Pitch from the instrument kernel.
CameraDetectorMap * p_detectorMap
A pointer to the DetectorMap.
CameraFocalPlaneMap * p_focalPlaneMap
A pointer to the FocalPlaneMap.
static double GroundAzimuth(double glat, double glon, double slat, double slon)
Computes and returns the ground azimuth between the ground point and another point of interest,...
CameraType
This enum defines the types of cameras supported in this class.
double ComputeAzimuth(const double lat, const double lon)
Computes the image azimuth value from your current position (origin) to a point of interest specified...
double LowestObliqueImageResolution()
Returns the lowest/worst oblique resolution in the entire image.
double HighestImageResolution()
Returns the highest/best resolution in the entire image.
QString instrumentId()
This method returns the InstrumentId as it appears in the cube.
@ PushFrame
Push Frame Camera.
int p_childBand
Band value for child.
bool p_ringRangeComputed
Flag showing if ring range was computed successfully.
virtual double LineResolution()
Returns the line resolution at the current position in meters.
CameraDistortionMap * p_distortionMap
A pointer to the DistortionMap.
double p_childSample
Sample value for child.
virtual bool SetUniversalGround(const double latitude, const double longitude)
Sets the lat/lon values to get the sample/line values.
double p_pixelPitch
The pixel pitch, in millimeters per pixel.
virtual QList< QPointF > PixelIfovOffsets()
Returns the pixel ifov offsets from center of pixel, which defaults to the (pixel pitch * summing mod...
double CelestialNorthClockAngle()
Computes the celestial north clock angle at the current line/sample or ra/dec.
double p_minlon180
The minimum longitude in the 180 domain.
AlphaCube * p_alphaCube
A pointer to the AlphaCube.
Distort/undistort focal plane coordinates.
double p_minres
The minimum resolution.
void SetFocalPlaneMap(CameraFocalPlaneMap *map)
Sets the Focal Plane Map.
double NorthAzimuth()
Returns the North Azimuth.
IO Handler for Isis Cubes.
This class is used to rewrite the "alpha" keywords out of the AlphaCube group or Instrument group.
QString m_spacecraftNameLong
Full spacecraft name.
Defines an angle and provides unit conversions.
int p_samples
The number of samples in the image.
bool SetImageMapProjection(const double sample, const double line, ShapeModel *shape)
Sets the sample/line values of the image to get the lat/lon values for a Map Projected image.
double p_maxRingRadius
The maximum ring radius.
double p_maxlon
The maximum longitude.
@ Csm
Community Sensor Model Camera.
int Bands() const
Returns the number of bands in the image.
bool p_raDecRangeComputed
Flag showing if the raDec range has been computed successfully.
double p_maxRingLongitude180
The maximum ring longitude in the 180 domain.
virtual bool IsBandIndependent()
Virtual method that checks if the band is independent.
int Samples() const
Returns the number of samples in the image.
bool p_pointComputed
Flag showing if Sample/Line has been computed.
void BasicMapping(Pvl &map)
Writes the basic mapping group to the specified Pvl.
bool p_groundRangeComputed
Flag showing if ground range was computed successfully.
double RaDecResolution()
Returns the RaDec resolution.
Class for computing sensor ground coordinates.
double p_maxra
The maxumum right ascension.
int p_geometricTilingStartSize
The ideal geometric tile size to start with when projecting.
CameraFocalPlaneMap * FocalPlaneMap()
Returns a pointer to the CameraFocalPlaneMap object.
double FocalLength() const
Returns the focal length.
void LoadCache()
This loads the spice cache big enough for this image.
Convert between undistorted focal plane coordinate (slant range) and ground coordinates.
Define shapes and provide utilities for Isis targets.
QString m_instrumentNameShort
Shortened instrument name.
Convert between distorted focal plane and detector coordinates.
CameraGroundMap * GroundMap()
Returns a pointer to the CameraGroundMap object.
CameraDetectorMap * DetectorMap()
Returns a pointer to the CameraDetectorMap object.
double PixelPitch() const
Returns the pixel pitch.
virtual double PixelResolution()
Returns the pixel resolution at the current position in meters/pixel.
Convert between undistorted focal plane and ra/dec coordinates.
virtual int SpkCenterId() const
Provides the center of motion body for SPK NAIF kernel.
bool GroundRange(double &minlat, double &maxlat, double &minlon, double &maxlon, Pvl &pvl)
Computes the Ground Range.
double p_minobliqueres
The minimum oblique resolution.
CameraGroundMap * p_groundMap
A pointer to the GroundMap.
void GetLocalNormal(double normal[3])
This method will find the local normal at the current (sample, line) and set it to the passed in arra...
double HighestObliqueImageResolution()
Returns the highest/best oblique resolution in the entire image.
int Lines() const
Returns the number of lines in the image.
void LocalPhotometricAngles(Angle &phase, Angle &incidence, Angle &emission, bool &success)
Calculates LOCAL photometric angles using the DEM (not ellipsoid).
QString instrumentNameShort() const
This method returns the shortened instrument name.
virtual double SampleResolution()
Returns the sample resolution at the current position in meters.
virtual double Line() const
Returns the current line number.
QString spacecraftNameLong() const
This method returns the full spacecraft name.
virtual int SpkReferenceId() const =0
Provides reference frame for instruments SPK NAIF kernel.
Base class for Map Projections.
virtual double ObliqueLineResolution()
Returns the oblique line resolution at the current position in meters.
int ReferenceBand() const
Returns the reference band.
QString m_spacecraftNameShort
Shortened spacecraft name.
double p_minra180
The minimum right ascension in the 180 domain.
double LowestImageResolution()
Returns the lowest/worst resolution in the entire image.
bool HasProjection()
Checks to see if the camera object has a projection.
double SunAzimuth()
Returns the Sun Azimuth.
QString spacecraftNameShort() const
This method returns the shortened spacecraft name.
bool ringRange(double &minRingRadius, double &maxRingRadius, double &minRingLongitude, double &maxRingLongitude, Pvl &pvl)
Analogous to the above Ground Range method.
double p_maxlat
The maximum latitude.
virtual double DetectorResolution()
Returns the detector resolution at the current position in meters.
void GetGeometricTilingHint(int &startSize, int &endSize)
This will get the geometric tiling hint; these values are typically used for ProcessRubberSheet::SetT...
This class defines a body-fixed surface point.
int p_geometricTilingEndSize
The ideal geometric tile size to end with when projecting.
void SetFocalLength()
Reads the focal length from the instrument kernel.
This is free and unencumbered software released into the public domain.
double p_minra
The minimum right ascension.
void SetDistortionMap(CameraDistortionMap *map, bool deleteExisting=true)
Sets the Distortion Map.