File failed to load: https://isis.astrogeology.usgs.gov/6.0.0/Object/assets/jax/output/NativeMML/config.js
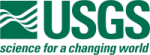 |
Isis 3 Programmer Reference
|
1 #ifndef RadarGroundMap_h
2 #define RadarGroundMap_h
11 #include "CameraGroundMap.h"
12 #include "SurfacePoint.h"
16 #ifndef RADAR_LOOK_DIR
18 enum LookDirection { Left, Right };
20 #define RADAR_LOOK_DIR
106 double *cudy,
bool test=
false);
108 int coefIndex,
double *cudx,
double *cudy);
109 virtual bool GetdXYdPoint(std::vector<double> d_lookB,
double *dx,
138 double ComputeXv(SpiceDouble X[3]);
141 bool Iterate(SpiceDouble &R,
const double &slantRangeSqr,
const SpiceDouble c[],
142 const SpiceDouble r[], SpiceDouble X[], SpiceDouble &lat,
143 SpiceDouble &lon,
const std::vector<double> &Xsc,
const bool &useSlopeEqn,
144 const double &slope);
146 Radar::LookDirection p_lookDirection;
150 double p_timeTolerance;
154 std::vector<double> p_lookB;
155 std::vector<double> p_sB;
virtual bool SetFocalPlane(const double ux, const double uy, const double uz)
Compute ground position from slant range.
virtual bool GetXY(const SurfacePoint &spoint, double *cudx, double *cudy, bool test=false)
Compute undistorted focal plane coordinate from ground position using current Spice from SetImage cal...
This class is designed to encapsulate the concept of a Latitude.
double p_slantRange
units are km
double p_rangeSigma
Scaling factor to convert meters to focal plane coord.
void SetDopplerSigma(double dopplerSigma)
Set the doppler sigma.
double p_groundDopplerFreq
units are hertz
virtual bool SetGround(const Latitude &lat, const Longitude &lon)
Compute undistorted focal plane coordinate from ground position.
This class is designed to encapsulate the concept of a Longitude.
virtual ~RadarGroundMap()
Destructor.
Convert between undistorted focal plane and ground coordinates.
void SetRangeSigma(double rangeSigma)
Set the range sigma.
double WaveLength()
Return the wavelength.
double p_dopplerFreq
units are hertz
double p_dopplerSigma
Scaling factor to convert hertz to focal plane coord.
bool Iterate(SpiceDouble &R, const double &slantRangeSqr, const SpiceDouble c[], const SpiceDouble r[], SpiceDouble X[], SpiceDouble &lat, SpiceDouble &lon, const std::vector< double > &Xsc, const bool &useSlopeEqn, const double &slope)
Iteration loop for computing ground position from slant range.
double p_groundSlantRange
units are km
Convert between undistorted focal plane coordinate (slant range) and ground coordinates.
virtual bool GetdXYdPoint(std::vector< double > d_lookB, double *dx, double *dy)
Compute derivative of focal plane coordinate w/r to ground point from ground position using current S...
virtual bool GetdXYdPosition(const SpicePosition::PartialType varType, int coefIndex, double *cudx, double *cudy)
Compute derivative w/r to position of focal plane coordinate from ground position using current Spice...
double YScale()
Return the doppler sigma.
This class defines a body-fixed surface point.
This is free and unencumbered software released into the public domain.
double RangeSigma()
Return the range sigma.